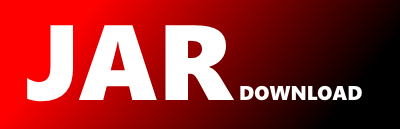
com.github.rexsheng.springboot.faster.system.log.application.LogServiceImpl Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.log.application;
import com.github.rexsheng.springboot.faster.common.domain.PagedList;
import com.github.rexsheng.springboot.faster.logging.QueueLogWriter;
import com.github.rexsheng.springboot.faster.system.log.application.dto.LogDetailResponse;
import com.github.rexsheng.springboot.faster.system.log.application.dto.QueryLogRequest;
import com.github.rexsheng.springboot.faster.system.log.domain.SysLog;
import com.github.rexsheng.springboot.faster.system.log.domain.gateway.LogGateway;
import com.github.rexsheng.springboot.faster.system.log.domain.gateway.QueryLogDO;
import com.github.rexsheng.springboot.faster.util.DateUtil;
import org.mybatis.spring.SqlSessionFactoryBean;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty;
import org.springframework.util.StringUtils;
@ConditionalOnClass(SqlSessionFactoryBean.class)
@ConditionalOnProperty(prefix = "app.module.log",name = "storage",havingValue = "db",matchIfMissing = true)
public class LogServiceImpl extends QueueLogWriter implements LogService{
private static final Logger logger= LoggerFactory.getLogger(LogServiceImpl.class);
private LogGateway logGateway;
public LogServiceImpl(LogGateway logGateway) {
this.logGateway = logGateway;
}
@Override
protected void flush(RequestLogEntity log) throws Exception {
SysLog sysLog = SysLog.of(log);
if(sysLog!=null){
logGateway.writeLog(sysLog);
}
}
@Override
public PagedList queryLogList(QueryLogRequest request) {
QueryLogDO query=new QueryLogDO();
query.setKeyword(request.getKeyword());
query.setError(request.getError());
query.setModule(request.getModule());
query.setRequestMethod(request.getRequestMethod());
query.setRequestUrl(request.getRequestUrl());
query.setUserId(request.getOperateUser());
query.setSearchNullableMethodNote(request.getSearchNullableMethodNote());
if(StringUtils.hasText(request.getStartTime())){
query.setStartTime(DateUtil.parseDateTime(request.getStartTime(),"yyyy-MM-dd HH:mm:ss"));
}
if(StringUtils.hasText(request.getEndTime())){
query.setEndTime(DateUtil.parseDateTime(request.getEndTime(),"yyyy-MM-dd HH:mm:ss"));
}
query.setPageIndex(request.getPageIndex());
query.setPageSize(request.getPageSize());
PagedList dataList=logGateway.paginateLog(query);
return PagedList.of(dataList, LogDetailResponse::of);
}
}