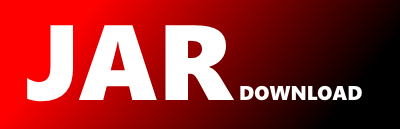
com.github.rexsheng.springboot.faster.system.menu.infrastructure.MenuGatewayImpl Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.menu.infrastructure;
import com.github.rexsheng.springboot.faster.system.entity.Menu;
import com.github.rexsheng.springboot.faster.system.entity.table.MenuTableDef;
import com.github.rexsheng.springboot.faster.system.mapper.MenuMapper;
import com.github.rexsheng.springboot.faster.system.menu.domain.SysMenu;
import com.github.rexsheng.springboot.faster.system.menu.domain.gateway.MenuGateway;
import com.github.rexsheng.springboot.faster.system.menu.domain.gateway.QueryMenuDO;
import com.mybatisflex.core.BaseMapper;
import com.mybatisflex.core.query.QueryWrapper;
import com.mybatisflex.core.row.Db;
import com.mybatisflex.core.update.UpdateChain;
import com.mybatisflex.core.util.UpdateEntity;
import jakarta.annotation.Resource;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.stereotype.Repository;
import org.springframework.util.StringUtils;
import java.util.List;
import java.util.stream.Collectors;
@Repository
@ConditionalOnClass(BaseMapper.class)
public class MenuGatewayImpl implements MenuGateway {
@Resource
private MenuMapper menuMapper;
@Override
public void insertMenu(SysMenu menu) {
Menu target=new Menu();
target.setParentId(menu.getParentId());
target.setCode(menu.getMenuCode());
target.setName(menu.getMenuName());
target.setPath(menu.getMenuPath());
target.setQuery(menu.getMenuPathQuery());
target.setOrder(menu.getMenuOrder());
target.setType(menu.getMenuType());
target.setIcon(menu.getMenuIcon());
target.setComponent(menu.getComponent());
target.setIsVisible(menu.getVisible());
target.setIsCache(menu.getCache());
target.setIsFrame(menu.getFrame());
target.setIsFullscreen(menu.getFullscreen());
target.setIsTag(menu.getTag());
target.setStatus(menu.getStatus());
target.setIsDel(menu.getDel());
target.setCreateTime(menu.getCreateTime());
target.setCreateUser(menu.getCreateUserId());
menuMapper.insert(target);
}
@Override
public List queryMenus(QueryMenuDO query) {
QueryWrapper queryWrapper=QueryWrapper.create().select()
.where(MenuTableDef.MENU.IS_DEL.eq(false))
.orderBy(MenuTableDef.MENU.ORDER,true)
.orderBy(MenuTableDef.MENU.ID,true)
;
if(query.getStatus()!=null){
queryWrapper.and(MenuTableDef.MENU.STATUS.eq(query.getStatus()));
}
if(StringUtils.hasText(query.getKeyword())){
queryWrapper.and(MenuTableDef.MENU.NAME.like(query.getKeyword()).or(MenuTableDef.MENU.CODE.like(query.getKeyword())));
}
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy