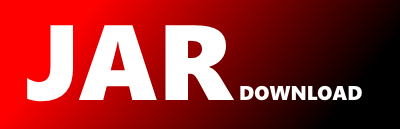
com.github.rexsheng.springboot.faster.system.modular.AppModuleProperties Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.modular;
import com.github.rexsheng.springboot.faster.io.file.minio.MinioProperties;
import com.github.rexsheng.springboot.faster.io.file.obs.ObsProperties;
import com.github.rexsheng.springboot.faster.io.file.oss.OssProperties;
import com.github.rexsheng.springboot.faster.io.file.qiniu.QiniuProperties;
import org.springframework.boot.context.properties.ConfigurationProperties;
import java.time.Duration;
import java.util.HashMap;
import java.util.Map;
@ConfigurationProperties(prefix = "app.module")
public class AppModuleProperties {
private ModuleProperty job;
private ModuleProperty workflow;
private ManagementModuleProperty management;
private NoticeModuleProperty notice;
private Boolean mybatisExtension;
private Boolean springdoc;
private LogModuleProperty log;
private Boolean oauth2;
private MonitorModuleProperty monitor;
private Boolean globalException;
private FileSystemModuleProperty file;
public ModuleProperty getJob() {
return job;
}
public void setJob(ModuleProperty job) {
this.job = job;
}
public ModuleProperty getWorkflow() {
return workflow;
}
public void setWorkflow(ModuleProperty workflow) {
this.workflow = workflow;
}
public ManagementModuleProperty getManagement() {
return management;
}
public void setManagement(ManagementModuleProperty management) {
this.management = management;
}
public NoticeModuleProperty getNotice() {
return notice;
}
public void setNotice(NoticeModuleProperty notice) {
this.notice = notice;
}
public Boolean getMybatisExtension() {
return mybatisExtension;
}
public void setMybatisExtension(Boolean mybatisExtension) {
this.mybatisExtension = mybatisExtension;
}
public Boolean getSpringdoc() {
return springdoc;
}
public void setSpringdoc(Boolean springdoc) {
this.springdoc = springdoc;
}
public LogModuleProperty getLog() {
return log;
}
public void setLog(LogModuleProperty log) {
this.log = log;
}
public Boolean getOauth2() {
return oauth2;
}
public void setOauth2(Boolean oauth2) {
this.oauth2 = oauth2;
}
public MonitorModuleProperty getMonitor() {
return monitor;
}
public void setMonitor(MonitorModuleProperty monitor) {
this.monitor = monitor;
}
public Boolean getGlobalException() {
return globalException;
}
public void setGlobalException(Boolean globalException) {
this.globalException = globalException;
}
public FileSystemModuleProperty getFile() {
return file;
}
public void setFile(FileSystemModuleProperty file) {
this.file = file;
}
public static class ModuleProperty{
private Boolean enabled;
private Boolean controller;
public Boolean getEnabled() {
return enabled;
}
public void setEnabled(Boolean enabled) {
this.enabled = enabled;
}
public Boolean getController() {
return controller;
}
public void setController(Boolean controller) {
this.controller = controller;
}
}
public static class ManagementModuleProperty extends ModuleProperty{
private Integer passwordExpirationDays=90;
public Integer getPasswordExpirationDays() {
return passwordExpirationDays;
}
public void setPasswordExpirationDays(Integer passwordExpirationDays) {
this.passwordExpirationDays = passwordExpirationDays;
}
}
public static class NoticeModuleProperty extends ModuleProperty{
private Boolean sse;
private String[] imageSuffix=new String[]{".png",".jpg",".jpeg",".gif",".bmp"};
private Long imageSize=2*1024*1024L;
private String bucketName="notice";
private String[] fileSuffix=new String[]{".pdf",".ppt",".xlsx",".xls",".csv",".doc",".docx",".zip",".7z",".rar",
".jpg",".jpeg",".bmp",".png",".gif",".tif",
".mp3",".wma",".wav",".ape",".flac",".ogg",".aac",
".mp4",".3gp",".mov",".avi",".rm",".rmvb",".wmv",".mkv",".flv",".mpeg"};
public Boolean getSse() {
return sse;
}
public void setSse(Boolean sse) {
this.sse = sse;
}
public String[] getImageSuffix() {
return imageSuffix;
}
public void setImageSuffix(String[] imageSuffix) {
this.imageSuffix = imageSuffix;
}
public Long getImageSize() {
return imageSize;
}
public void setImageSize(Long imageSize) {
this.imageSize = imageSize;
}
public String getBucketName() {
return bucketName;
}
public void setBucketName(String bucketName) {
this.bucketName = bucketName;
}
public String[] getFileSuffix() {
return fileSuffix;
}
public void setFileSuffix(String[] fileSuffix) {
this.fileSuffix = fileSuffix;
}
}
public static class LogModuleProperty extends ModuleProperty{
private StorageLocation storage=StorageLocation.file;
private Log4j2ModuleProperty log4j;
public Log4j2ModuleProperty getLog4j() {
return log4j;
}
public void setLog4j(Log4j2ModuleProperty log4j) {
this.log4j = log4j;
}
public enum StorageLocation{
file,db;
}
public static class Log4j2ModuleProperty{
/**
* 日志存放目录
*/
private String home;
/**
* 文件前缀
*/
private String filePrefix;
/**
* 文件超过最大值后生成归档, 例如10MB
*/
private String fileSize;
/**
* 最多一天滚动文件个数,默认10个
*/
private Integer fileIndex;
/**
* 最长存放时间,默认3600D
*/
private String fileAge;
public String getHome() {
return home;
}
public void setHome(String home) {
this.home = home;
}
public String getFilePrefix() {
return filePrefix;
}
public void setFilePrefix(String filePrefix) {
this.filePrefix = filePrefix;
}
public String getFileSize() {
return fileSize;
}
public void setFileSize(String fileSize) {
this.fileSize = fileSize;
}
public Integer getFileIndex() {
return fileIndex;
}
public void setFileIndex(Integer fileIndex) {
this.fileIndex = fileIndex;
}
public String getFileAge() {
return fileAge;
}
public void setFileAge(String fileAge) {
this.fileAge = fileAge;
}
}
}
public static class MonitorModuleProperty extends ModuleProperty{
private Duration interval=Duration.ofSeconds(60L);
private Duration timeout=Duration.ofMinutes(3L);
/**
* 最大历史记录数量
*/
private Integer historyLimit=10;
private Disk disk;
private NetworkInterface networkInterface;
private Process process;
public Duration getInterval() {
return interval;
}
public void setInterval(Duration interval) {
this.interval = interval;
}
public Duration getTimeout() {
return timeout;
}
public void setTimeout(Duration timeout) {
this.timeout = timeout;
}
public Integer getHistoryLimit() {
return historyLimit;
}
public void setHistoryLimit(Integer historyLimit) {
this.historyLimit = historyLimit;
}
public Disk getDisk() {
return disk;
}
public void setDisk(Disk disk) {
this.disk = disk;
}
public NetworkInterface getNetworkInterface() {
return networkInterface;
}
public void setNetworkInterface(NetworkInterface networkInterface) {
this.networkInterface = networkInterface;
}
public Process getProcess() {
return process;
}
public void setProcess(Process process) {
this.process = process;
}
public static class Disk{
private String[] include;
public String[] getInclude() {
return include;
}
public void setInclude(String[] include) {
this.include = include;
}
}
public static class NetworkInterface{
private String[] include;
/**
* set the networkinterface max speed with unit MB when can't detect it
*/
private Long maxSpeed;
public String[] getInclude() {
return include;
}
public void setInclude(String[] include) {
this.include = include;
}
public Long getMaxSpeed() {
return maxSpeed;
}
public void setMaxSpeed(Long maxSpeed) {
this.maxSpeed = maxSpeed;
}
}
public static class Process{
/**
* 是否获取程序运行参数,默认是
*/
private Boolean arg=true;
/**
* 是否获取环境变量,默认否
*/
private Boolean env=false;
/**
* 最大进行数量
*/
private Integer limit=10;
public Boolean getArg() {
return arg;
}
public void setArg(Boolean arg) {
this.arg = arg;
}
public Boolean getEnv() {
return env;
}
public void setEnv(Boolean env) {
this.env = env;
}
public Integer getLimit() {
return limit;
}
public void setLimit(Integer limit) {
this.limit = limit;
}
}
}
public static class FileSystemModuleProperty extends ModuleProperty{
private FileSystemType type=FileSystemType.local;
private LocalFileProperties local;
private ObsProperties obs;
private OssProperties oss;
private MinioProperties minio;
private QiniuProperties qiniu;
public FileSystemType getType() {
return type;
}
public void setType(FileSystemType type) {
this.type = type;
}
public LocalFileProperties getLocal() {
return local;
}
public void setLocal(LocalFileProperties local) {
this.local = local;
}
public ObsProperties getObs() {
return obs;
}
public void setObs(ObsProperties obs) {
this.obs = obs;
}
public OssProperties getOss() {
return oss;
}
public void setOss(OssProperties oss) {
this.oss = oss;
}
public MinioProperties getMinio() {
return minio;
}
public void setMinio(MinioProperties minio) {
this.minio = minio;
}
public QiniuProperties getQiniu() {
return qiniu;
}
public void setQiniu(QiniuProperties qiniu) {
this.qiniu = qiniu;
}
public enum FileSystemType{
local,obs,oss,minio,qiniu;
}
public static class LocalFileProperties{
private Map pathMapping=new HashMap<>();
public Map getPathMapping() {
return pathMapping;
}
public void setPathMapping(Map pathMapping) {
this.pathMapping = pathMapping;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy