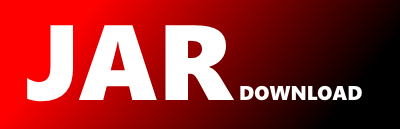
com.github.rexsheng.springboot.faster.system.notice.application.NoticeServiceImpl Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.notice.application;
import com.github.rexsheng.springboot.faster.common.constant.CommonConstant;
import com.github.rexsheng.springboot.faster.common.domain.PagedList;
import com.github.rexsheng.springboot.faster.spring.SpringContext;
import com.github.rexsheng.springboot.faster.system.file.application.FileService;
import com.github.rexsheng.springboot.faster.system.file.application.dto.*;
import com.github.rexsheng.springboot.faster.system.modular.AppModuleProperties;
import com.github.rexsheng.springboot.faster.system.notice.application.dto.*;
import com.github.rexsheng.springboot.faster.system.notice.domain.NoticeBroadcastEvent;
import com.github.rexsheng.springboot.faster.system.notice.domain.SysNotice;
import com.github.rexsheng.springboot.faster.system.notice.domain.gateway.NoticeGateway;
import com.github.rexsheng.springboot.faster.system.notice.domain.gateway.QueryNoticeDO;
import com.github.rexsheng.springboot.faster.system.notice.domain.gateway.QueryUserNoticeDO;
import jakarta.annotation.Resource;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.context.annotation.Lazy;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.util.Assert;
import org.springframework.util.ObjectUtils;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
@Service
@Lazy
public class NoticeServiceImpl implements NoticeService {
private static final Logger logger= LoggerFactory.getLogger(NoticeServiceImpl.class);
@Resource
@Lazy
private NoticeGateway noticeGateway;
@Resource
@Lazy
private FileService fileService;
@Resource
@Lazy
private AppModuleProperties appModuleProperties;
@Override
public PagedList pagedList(QueryNoticeRequest request) {
QueryNoticeDO query=new QueryNoticeDO();
query.setTitle(request.getTitle());
query.setPageIndex(request.getPageIndex());
query.setPageSize(request.getPageSize());
PagedList dataList=noticeGateway.paginateNotices(query);
return PagedList.of(dataList, NoticeDetailResponse::of);
}
@Override
@Transactional
public void addNotice(AddNoticeRequest request) {
SysNotice notice = request.toDomain();
validateUploadFiles(notice);
noticeGateway.insertNotice(notice);
if(!ObjectUtils.isEmpty(notice.getNoticeFiles())){
if(fileService==null){
logger.warn("请启用app.module.file.enabled=true");
return;
}
for(SysNotice.SysNoticeFile sysNoticeFile:notice.getNoticeFiles()){
sysNoticeFile.setFileId(saveNoticeFile(sysNoticeFile,notice));
}
}
SpringContext.getApplicationContext().publishEvent(new NoticeBroadcastEvent(notice));
}
/**
* 保存新上传的文件
* @param sysNoticeFile
* @param notice
* @return
*/
private String saveNoticeFile(SysNotice.SysNoticeFile sysNoticeFile, SysNotice notice){
String noticeIdStr=String.valueOf(notice.getNoticeId());
AddFileRequest addFileRequest=new AddFileRequest(CommonConstant.FILE_BUSINESS_TYPE_NOTICE,noticeIdStr,
appModuleProperties.getNotice().getBucketName(),CommonConstant.FILE_BUSINESS_TYPE_NOTICE+"/"+noticeIdStr+"/"+sysNoticeFile.generateObjectKey(),
sysNoticeFile.getFileName(),sysNoticeFile.getOriginalFileName(),sysNoticeFile.getFileSize(),sysNoticeFile.getFileContent());
AddFileResponse addFileResponse=fileService.addFile(addFileRequest);
if(addFileResponse==null){
throw new RuntimeException("文件保存失败:"+sysNoticeFile.getOriginalFileName());
}
else{
return addFileResponse.getId();
}
}
@Override
public NoticeDetailResponse getNotice(Long id) {
SysNotice data= noticeGateway.getNotice(id);
Assert.isTrue(data!=null && !Boolean.TRUE.equals(data.getDel()),"公告不存在");
QueryFileRequest queryFileRequest=new QueryFileRequest();
queryFileRequest.setBusinessType(CommonConstant.FILE_BUSINESS_TYPE_NOTICE);
queryFileRequest.setBusinessId(String.valueOf(id));
List fileList=fileService.queryList(queryFileRequest);
if(!ObjectUtils.isEmpty(fileList)){
data.setNoticeFiles(fileList.stream().map(SysNotice.SysNoticeFile::of).collect(Collectors.toList()));
}
return NoticeDetailResponse.of(data);
}
private void validateUploadFiles(SysNotice sysNotice){
if(sysNotice.getNoticeFiles()!=null && appModuleProperties.getNotice()!=null && appModuleProperties.getNotice().getFileSuffix()!=null){
for(SysNotice.SysNoticeFile sysNoticeFile:sysNotice.getNoticeFiles()){
if(sysNoticeFile.getFileId()!=null){
continue;
}
if(!Arrays.stream(appModuleProperties.getNotice().getFileSuffix())
.anyMatch(a->sysNoticeFile.getOriginalFileName().toLowerCase().endsWith(a))){
throw new IllegalArgumentException("不支持的文件格式:"+sysNoticeFile.getOriginalFileName());
}
}
}
}
@Override
@Transactional
public void update(UpdateNoticeRequest request) {
SysNotice data=request.toDomain();
validateUploadFiles(data);
noticeGateway.updateNoticeById(data);
String noticeIdStr=String.valueOf(data.getNoticeId());
QueryFileRequest queryFileRequest=new QueryFileRequest();
queryFileRequest.setBusinessType(CommonConstant.FILE_BUSINESS_TYPE_NOTICE);
queryFileRequest.setBusinessId(noticeIdStr);
List oldFileList=fileService.queryList(queryFileRequest).stream().map(a->a.getId()).collect(Collectors.toList());
List currentList=!ObjectUtils.isEmpty(data.getNoticeFiles())?
data.getNoticeFiles().stream().filter(a->a.getFileId()!=null).map(a->a.getFileId()).collect(Collectors.toList())
:new ArrayList<>();
if(!ObjectUtils.isEmpty(currentList) && !ObjectUtils.isEmpty(oldFileList) && fileService==null){
logger.warn("请启用app.module.file.enabled=true");
return;
}
if(ObjectUtils.isEmpty(currentList) && !ObjectUtils.isEmpty(oldFileList)){
fileService.deleteFiles(oldFileList);
}
else if(!ObjectUtils.isEmpty(currentList)){
fileService.deleteFilesIfNotExists(currentList,oldFileList);
}
if(!ObjectUtils.isEmpty(data.getNoticeFiles())){
for(SysNotice.SysNoticeFile sysNoticeFile:data.getNoticeFiles()){
if(sysNoticeFile.getFileId()!=null){
continue;
}
sysNoticeFile.setFileId(saveNoticeFile(sysNoticeFile,data));
}
}
SpringContext.getApplicationContext().publishEvent(new NoticeBroadcastEvent(data));
}
@Override
public void updateStatus(List request) {
List dataList=request.stream().map(UpdateNoticeRequest::toDomain).collect(Collectors.toList());
noticeGateway.updateNoticeStatus(dataList);
}
@Override
@Transactional
public void delete(List ids) {
noticeGateway.deleteNotices(SysNotice.of(ids,true));
for(Long id:ids){
DeleteFileRequest deleteFileRequest=new DeleteFileRequest();
deleteFileRequest.setBusinessType(CommonConstant.FILE_BUSINESS_TYPE_NOTICE);
deleteFileRequest.setBusinessId(String.valueOf(id));
fileService.deleteFiles(deleteFileRequest);
}
}
@Override
public PagedList userNoticeList(QueryUserNoticeRequest request) {
QueryUserNoticeDO query=new QueryUserNoticeDO();
query.setUserId(request.getUserId());
query.setReaded(request.getReaded());
query.setPageIndex(request.getPageIndex());
query.setPageSize(request.getPageSize());
PagedList dataList=noticeGateway.queryUserNotices(query);
return PagedList.of(dataList, NoticeDetailResponse::of);
}
@Override
public void updateNoticeToReaded(Long userId, Long noticeId, Long relationId) {
SysNotice notice=SysNotice.ofReadEntity(userId,noticeId, relationId);
noticeGateway.updateReaded(notice);
}
@Override
public void addInternalNotice(AddInternalNoticeRequest request) {
SysNotice notice=request.toDomain();
noticeGateway.insertInternalNotice(notice);
SpringContext.getApplicationContext().publishEvent(new NoticeBroadcastEvent(notice));
}
@Override
public void readAllNotice(Long userId) {
noticeGateway.updateAllReadedByUserId(userId);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy