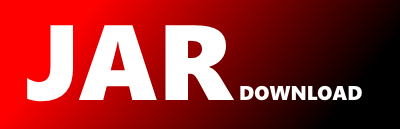
com.github.rexsheng.springboot.faster.system.notice.domain.SysNotice Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.notice.domain;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.github.rexsheng.springboot.faster.common.constant.CommonConstant;
import com.github.rexsheng.springboot.faster.system.file.application.dto.FileDetailResponse;
import com.github.rexsheng.springboot.faster.system.utils.AuthenticationUtil;
import com.github.rexsheng.springboot.faster.util.DateUtil;
import java.time.LocalDateTime;
import java.util.*;
import java.util.stream.Collectors;
public class SysNotice {
private Long noticeId;
private Long messageId;
private String noticeTitle;
private String noticeContent;
private String noticeType;
private LocalDateTime endTime;
private Integer status;
private Boolean isDel;
private LocalDateTime createTime;
private Long createUserId;
private LocalDateTime updateTime;
private Long updateUserId;
private LocalDateTime readTime;
private List noticeUsers;
private List noticeFiles;
public static Integer STATUS_NORMAL = CommonConstant.STATUS_RUNNING;
public String getBroadcastContent(){
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy