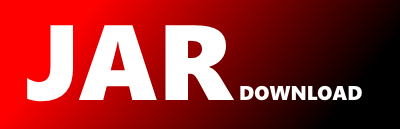
com.github.rexsheng.springboot.faster.system.post.domain.SysPost Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.post.domain;
import com.github.rexsheng.springboot.faster.system.utils.AuthenticationUtil;
import com.github.rexsheng.springboot.faster.util.DateUtil;
import java.time.LocalDateTime;
import java.util.List;
import java.util.stream.Collectors;
public class SysPost {
private Integer postId;
private String postName;
private Integer postOrder;
private Integer status;
private Boolean isDel;
private LocalDateTime createTime;
private Long createUserId;
private LocalDateTime updateTime;
private Long updateUserId;
public static List of(List ids, Boolean isDel){
return ids.stream().map(a->{
SysPost sysPost=new SysPost();
sysPost.setPostId(a);
sysPost.setDel(isDel);
sysPost.setUpdateTime(DateUtil.currentDateTime());
sysPost.setUpdateUserId(AuthenticationUtil.currentUserId());
return sysPost;
}).collect(Collectors.toList());
}
public Integer getPostId() {
return postId;
}
public void setPostId(Integer postId) {
this.postId = postId;
}
public String getPostName() {
return postName;
}
public void setPostName(String postName) {
this.postName = postName;
}
public Integer getPostOrder() {
return postOrder;
}
public void setPostOrder(Integer postOrder) {
this.postOrder = postOrder;
}
public Integer getStatus() {
return status;
}
public void setStatus(Integer status) {
this.status = status;
}
public Boolean getDel() {
return isDel;
}
public void setDel(Boolean del) {
isDel = del;
}
public LocalDateTime getCreateTime() {
return createTime;
}
public void setCreateTime(LocalDateTime createTime) {
this.createTime = createTime;
}
public Long getCreateUserId() {
return createUserId;
}
public void setCreateUserId(Long createUserId) {
this.createUserId = createUserId;
}
public LocalDateTime getUpdateTime() {
return updateTime;
}
public void setUpdateTime(LocalDateTime updateTime) {
this.updateTime = updateTime;
}
public Long getUpdateUserId() {
return updateUserId;
}
public void setUpdateUserId(Long updateUserId) {
this.updateUserId = updateUserId;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy