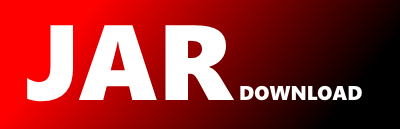
com.github.rexsheng.springboot.faster.system.user.domain.SysUser Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.user.domain;
import com.github.rexsheng.springboot.faster.common.constant.LocaleCategoryConstant;
import com.github.rexsheng.springboot.faster.common.domain.DomainFactory;
import com.github.rexsheng.springboot.faster.i18n.ObjectMessageSource;
import com.github.rexsheng.springboot.faster.system.user.domain.gateway.QueryUserDO;
import com.github.rexsheng.springboot.faster.system.user.domain.gateway.QueryUserTokenDO;
import com.github.rexsheng.springboot.faster.system.user.domain.gateway.UserGateway;
import com.github.rexsheng.springboot.faster.system.utils.AuthenticationUtil;
import com.github.rexsheng.springboot.faster.util.DateUtil;
import com.github.rexsheng.springboot.faster.util.PasswordUtils;
import org.springframework.context.i18n.LocaleContextHolder;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.util.ObjectUtils;
import java.time.LocalDateTime;
import java.util.List;
import java.util.stream.Collectors;
public class SysUser {
private Long userId;
private String userName;
private String loginAccount;
private String loginPassword;
private SysUserDept dept;
private List postList;
private List roleList;
private String mail;
private String phone;
private Boolean sex;
private String sexName;
private LocalDateTime lastLoginTime;
private LocalDateTime lastPasswordTime;
private LocalDateTime accountExpiredTime;
private LocalDateTime accountLockedTime;
private LocalDateTime passwordExpiredTime;
private Integer status;
private String statusName;
private String avatar;
private Boolean isDel;
private LocalDateTime createTime;
private Long createUserId;
private LocalDateTime updateTime;
private Long updateUserId;
private List tokenList;
public static SysUser of(Long userId){
SysUser sysUser=new SysUser();
sysUser.setUserId(userId);
return sysUser;
}
public static List of(List ids,Boolean isDel){
return ids.stream().map(a->{
SysUser sysUser=new SysUser();
sysUser.setUserId(a);
sysUser.setDel(isDel);
sysUser.setUpdateTime(DateUtil.currentDateTime());
sysUser.setUpdateUserId(AuthenticationUtil.currentUserId());
return sysUser;
}).collect(Collectors.toList());
}
public static SysUser deleteTokens(List ids){
SysUser sysUser=new SysUser();
LocalDateTime now = DateUtil.currentDateTime();
Long userId=AuthenticationUtil.currentUserId();
sysUser.setUpdateTime(now);
sysUser.setUpdateUserId(userId);
sysUser.setTokenList(ids.stream().map(a->{
SysUserToken userToken=new SysUserToken();
userToken.setTokenId(a);
userToken.setDel(true);
userToken.setUpdateTime(now);
userToken.setUpdateUserId(userId);
return userToken;
}).collect(Collectors.toList()));
return sysUser;
}
public void validateCurrentPassword(PasswordEncoder passwordEncoder,String oldPassword){
if(oldPassword!=null && !passwordEncoder.matches(oldPassword,this.getLoginPassword())){
throw new RuntimeException("旧密码错误");
}
}
public Boolean isAccountDuplicated(){
QueryUserDO query=new QueryUserDO();
query.setUserId(this.getUserId());
query.setAccount(this.getLoginAccount());
return DomainFactory.create(UserGateway.class).queryAccountCount(query)>0L;
}
public Boolean validateSex(String sexName){
ObjectMessageSource objectMessageSource= DomainFactory.create(ObjectMessageSource.class);
String sexId=objectMessageSource.resolveMessageToCode(sexName, LocaleContextHolder.getLocale(), LocaleCategoryConstant.SYS_USER_SEX);
if(!ObjectUtils.isEmpty(sexId)){
return Boolean.parseBoolean(sexId);
}
return null;
}
public Boolean isBusinessKeyDuplicated(){
if(!ObjectUtils.isEmpty(tokenList)){
QueryUserTokenDO query=new QueryUserTokenDO();
query.setBusinessKey(tokenList.get(0).getBusinessKey());
SysUser sysUser=DomainFactory.create(UserGateway.class).queryUserTokens(query);
return sysUser!=null && !ObjectUtils.isEmpty(sysUser.getTokenList());
}
return true;
}
public void createToken(){
if(this.getTokenList()!=null){
for (SysUserToken token:this.getTokenList()){
if(token.getTokenName()==null){
token.setTokenName(generateTokenName(token));
token.setTokenValue(generateTokenValue(token));
}
}
}
}
public boolean isTokenValid(){
if(this.getTokenList()!=null){
for (SysUserToken token:this.getTokenList()){
String rawTokenValue=generateTokenValue(token);
return rawTokenValue.equals(token.getTokenValue());
}
}
return false;
}
private String generateTokenName(SysUserToken token){
StringBuilder builder=new StringBuilder();
builder.append(token.getUserId());
builder.append("_");
builder.append(System.currentTimeMillis());
builder.append("_");
builder.append(PasswordUtils.createRandomPassword());
return PasswordUtils.md5(builder.toString());
}
private String generateTokenValue(SysUserToken token){
StringBuilder builder=new StringBuilder();
if(token.getTokenName()!=null){
builder.append(token.getTokenName());
}
builder.append("|");
if(token.getBusinessKey()!=null){
builder.append(token.getBusinessKey());
}
builder.append("|");
if(token.getUserId()!=null){
builder.append(token.getUserId());
}
builder.append("|");
return PasswordUtils.base64Encode(PasswordUtils.md5(builder.toString()));
}
public Long getUserId() {
return userId;
}
public void setUserId(Long userId) {
this.userId = userId;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getLoginAccount() {
return loginAccount;
}
public void setLoginAccount(String loginAccount) {
this.loginAccount = loginAccount;
}
public String getLoginPassword() {
return loginPassword;
}
public void setLoginPassword(String loginPassword) {
this.loginPassword = loginPassword;
}
public SysUserDept getDept() {
return dept;
}
public void setDept(SysUserDept dept) {
this.dept = dept;
}
public List getPostList() {
return postList;
}
public void setPostList(List postList) {
this.postList = postList;
}
public List getRoleList() {
return roleList;
}
public void setRoleList(List roleList) {
this.roleList = roleList;
}
public String getMail() {
return mail;
}
public void setMail(String mail) {
this.mail = mail;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public Boolean getSex() {
return sex;
}
public void setSex(Boolean sex) {
this.sex = sex;
}
public String getSexName() {
return sexName;
}
public void setSexName(String sexName) {
this.sexName = sexName;
}
public LocalDateTime getLastLoginTime() {
return lastLoginTime;
}
public void setLastLoginTime(LocalDateTime lastLoginTime) {
this.lastLoginTime = lastLoginTime;
}
public LocalDateTime getLastPasswordTime() {
return lastPasswordTime;
}
public void setLastPasswordTime(LocalDateTime lastPasswordTime) {
this.lastPasswordTime = lastPasswordTime;
}
public LocalDateTime getAccountExpiredTime() {
return accountExpiredTime;
}
public void setAccountExpiredTime(LocalDateTime accountExpiredTime) {
this.accountExpiredTime = accountExpiredTime;
}
public LocalDateTime getAccountLockedTime() {
return accountLockedTime;
}
public void setAccountLockedTime(LocalDateTime accountLockedTime) {
this.accountLockedTime = accountLockedTime;
}
public LocalDateTime getPasswordExpiredTime() {
return passwordExpiredTime;
}
public void setPasswordExpiredTime(LocalDateTime passwordExpiredTime) {
this.passwordExpiredTime = passwordExpiredTime;
}
public Integer getStatus() {
return status;
}
public void setStatus(Integer status) {
this.status = status;
}
public String getStatusName() {
return statusName;
}
public void setStatusName(String statusName) {
this.statusName = statusName;
}
public String getAvatar() {
return avatar;
}
public void setAvatar(String avatar) {
this.avatar = avatar;
}
public Boolean getDel() {
return isDel;
}
public void setDel(Boolean del) {
isDel = del;
}
public LocalDateTime getCreateTime() {
return createTime;
}
public void setCreateTime(LocalDateTime createTime) {
this.createTime = createTime;
}
public Long getCreateUserId() {
return createUserId;
}
public void setCreateUserId(Long createUserId) {
this.createUserId = createUserId;
}
public LocalDateTime getUpdateTime() {
return updateTime;
}
public void setUpdateTime(LocalDateTime updateTime) {
this.updateTime = updateTime;
}
public Long getUpdateUserId() {
return updateUserId;
}
public void setUpdateUserId(Long updateUserId) {
this.updateUserId = updateUserId;
}
public List getTokenList() {
return tokenList;
}
public void setTokenList(List tokenList) {
this.tokenList = tokenList;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy