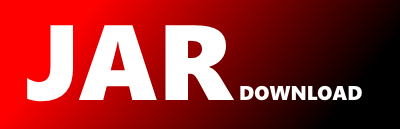
com.github.rexsheng.springboot.faster.system.user.domain.SysUserToken Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.user.domain;
import java.time.LocalDateTime;
public class SysUserToken {
private Long userId;
private Long tokenId;
private String tokenName;
private String tokenValue;
private String businessKey;
private LocalDateTime expireTime;
private LocalDateTime createTime;
private Long createUserId;
private Long updateUserId;
private LocalDateTime updateTime;
private Boolean isDel;
public Long getUserId() {
return userId;
}
public void setUserId(Long userId) {
this.userId = userId;
}
public Long getTokenId() {
return tokenId;
}
public void setTokenId(Long tokenId) {
this.tokenId = tokenId;
}
public String getTokenName() {
return tokenName;
}
public void setTokenName(String tokenName) {
this.tokenName = tokenName;
}
public String getTokenValue() {
return tokenValue;
}
public void setTokenValue(String tokenValue) {
this.tokenValue = tokenValue;
}
public String getBusinessKey() {
return businessKey;
}
public void setBusinessKey(String businessKey) {
this.businessKey = businessKey;
}
public LocalDateTime getExpireTime() {
return expireTime;
}
public void setExpireTime(LocalDateTime expireTime) {
this.expireTime = expireTime;
}
public LocalDateTime getCreateTime() {
return createTime;
}
public void setCreateTime(LocalDateTime createTime) {
this.createTime = createTime;
}
public Boolean getDel() {
return isDel;
}
public void setDel(Boolean del) {
isDel = del;
}
public Long getCreateUserId() {
return createUserId;
}
public void setCreateUserId(Long createUserId) {
this.createUserId = createUserId;
}
public Long getUpdateUserId() {
return updateUserId;
}
public void setUpdateUserId(Long updateUserId) {
this.updateUserId = updateUserId;
}
public LocalDateTime getUpdateTime() {
return updateTime;
}
public void setUpdateTime(LocalDateTime updateTime) {
this.updateTime = updateTime;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy