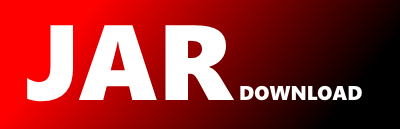
com.github.rexsheng.springboot.faster.system.utils.AuthenticationUtil Maven / Gradle / Ivy
The newest version!
package com.github.rexsheng.springboot.faster.system.utils;
import com.github.rexsheng.springboot.faster.system.auth.domain.SysUserDetail;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
import org.springframework.security.authentication.AnonymousAuthenticationToken;
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.context.SecurityContext;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.security.core.context.SecurityContextHolderStrategy;
import org.springframework.security.web.context.RequestAttributeSecurityContextRepository;
import org.springframework.security.web.context.SecurityContextRepository;
public class AuthenticationUtil {
private static SecurityContextHolderStrategy securityContextHolderStrategy = SecurityContextHolder.getContextHolderStrategy();
private static SecurityContextRepository securityContextRepository = new RequestAttributeSecurityContextRepository();
public static Long currentUserId(){
Authentication authentication=currentAuthentication();
return getUserIdFromAuthentication(authentication);
}
public static Long getUserIdFromAuthentication(Authentication authentication){
if(authentication==null){
return null;
}
SysUserDetail detail=getUserFromAuthentication(authentication);
if(detail!=null){
return detail.getUserId();
}
return null;
}
public static SysUserDetail currentUser(){
return getUserFromAuthentication(currentAuthentication());
}
public static SysUserDetail getUserFromAuthentication(Authentication authentication){
if(authentication==null || authentication instanceof AnonymousAuthenticationToken){
return null;
}
UsernamePasswordAuthenticationToken authenticationToken=(UsernamePasswordAuthenticationToken)authentication;
return (SysUserDetail) authenticationToken.getPrincipal();
}
public static Authentication currentAuthentication(){
SecurityContext holder= SecurityContextHolder.getContext();
if(holder!=null){
return holder.getAuthentication();
}
return null;
}
public static void setSecurityContext(Authentication authentication, HttpServletRequest request, HttpServletResponse response){
SecurityContext context = securityContextHolderStrategy.createEmptyContext();
context.setAuthentication(authentication);
securityContextHolderStrategy.setContext(context);
securityContextRepository.saveContext(context, request, response);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy