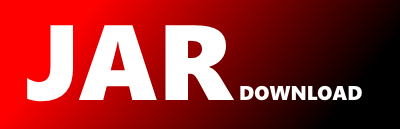
bdsup2sub.tools.Props Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2014 Volker Oth (0xdeadbeef) / Miklos Juhasz (mjuhasz)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package bdsup2sub.tools;
import java.io.*;
import java.net.URL;
import java.util.Properties;
/**
* Property class to ease use of ini files to save/load properties
*/
public class Props {
/** extended hash to store properties */
private Properties hash;
/** header string */
private String header;
public Props() {
this.hash = new Properties();
this.header = "";
}
/**
* Set the property file header
* @param header String containing Header information
*/
public void setHeader(String header) {
this.header = header;
}
/**
* Clear all properties
*/
public void clear() {
hash.clear();
}
/**
* Remove key
* @param key Name of key
*/
public void remove(String key) {
hash.remove(key);
}
/**
* Set string property
* @param key Name of the key to set value for
* @param value Value to set
*/
public void set(String key, String value) {
hash.setProperty(key, value);
}
/**
* Set integer property
* @param key Name of the key to set value for
* @param value Value to set
*/
public void set(String key, int value) {
hash.setProperty(key, String.valueOf(value));
}
/**
* Set boolean property
* @param key Name of the key to set value for
* @param value Value to set
*/
public void set(String key, boolean value) {
hash.setProperty(key, String.valueOf(value));
}
/**
* Set double property
* @param key Name of the key to set value for
* @param value Value to set
*/
public void set(String key, double value) {
hash.setProperty(key, String.valueOf(value));
}
/**
* Get string property
* @param key Name of the key to get value for
* @param def Default value in case key is not found
* @return Value of key as String
*/
public String get(String key, String def) {
String s = hash.getProperty(key,def);
return removeComment(s);
}
/**
* Get integer property
* @param key Name of the key to get value for
* @param def Default value in case key is not found
* @return Value of key as int
*/
public int get(String key, int def) {
String s = hash.getProperty(key);
if (s == null) {
return def;
}
s = removeComment(s);
return parseString(s);
}
/**
* Get integer array property
* @param key Name of the key to get value for
* @param def Default value in case key is not found
* @return Value of key as array of int
*/
public int[] get(String key, int def[]) {
String s = hash.getProperty(key);
if (s == null) {
return def;
}
s = removeComment(s);
String members[] = s.split(",");
// remove trailing and leading spaces
for (int i=0; i 2 && s.charAt(1) == 'x') {
return Integer.parseInt(s.substring(2),16); // hex
} else if (s.charAt(1) == 'b') {
return Integer.parseInt(s.substring(2),2); // binary
} else {
return Integer.parseInt(s.substring(0),8); // octal
}
}
int retval;
try {
retval = Integer.parseInt(s);
} catch (NumberFormatException ex) {
retval = 0;
}
return retval;
}
/**
* Remove comment from line. Comment character is "#".
* Everything behind (including "#") will be removed
* @param s String to search for comment
* @return String without comment
*/
private String removeComment(String s) {
int pos = s.indexOf('#');
if (pos != -1) {
return s.substring(0,pos);
} else {
return s;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy