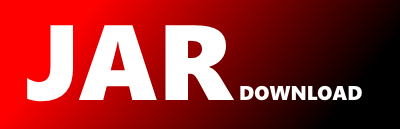
rinde.sim.pdptw.central.SolverDebugger Maven / Gradle / Ivy
The newest version!
/**
*
*/
package rinde.sim.pdptw.central;
import static com.google.common.collect.Lists.newArrayList;
import static java.util.Collections.unmodifiableList;
import java.util.List;
import rinde.sim.pdptw.common.ParcelDTO;
import com.google.common.collect.ImmutableList;
/**
* Allows keeping track of the inputs and outputs of a {@link Solver}.
* @author Rinde van Lon
*/
public final class SolverDebugger implements Solver {
private final List inputs;
private final List>> outputs;
private final Solver delegate;
private final boolean print;
private SolverDebugger(Solver delegate, boolean debugPrints) {
this.delegate = delegate;
print = debugPrints;
inputs = newArrayList();
outputs = newArrayList();
}
@Override
public ImmutableList> solve(GlobalStateObject state) {
if (print) {
System.out.println(state);
}
inputs.add(state);
final ImmutableList> result = delegate
.solve(state);
outputs.add(result);
if (print) {
System.out.println(result);
}
return result;
}
/**
* @return An unmodifiable view on the inputs that were used when calling
* {@link #solve(GlobalStateObject)}. The list is in order of
* invocation.
*/
public List getInputs() {
return unmodifiableList(inputs);
}
/**
* @return An unmodifiable view on the outputs that are generated when calling
* {@link #solve(GlobalStateObject)}. The list is in order of
* invocation.
*/
public List>> getOutputs() {
return unmodifiableList(outputs);
}
/**
* Wraps the specified solver in a {@link SolverDebugger} instance to allow
* easier debugging of a solver.
* @param s The solver to wrap.
* @param print Whether debug printing should be enabled.
* @return The wrapped solver.
*/
public static SolverDebugger wrap(Solver s, boolean print) {
return new SolverDebugger(s, print);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy