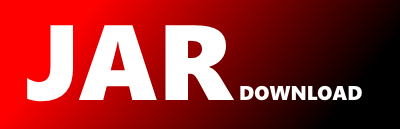
remote.Remote Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of remote4j Show documentation
Show all versions of remote4j Show documentation
Thin functional layer on top of RMI.
package remote;
import java.lang.ref.Reference;
import java.lang.ref.WeakReference;
import java.net.MalformedURLException;
import java.rmi.Naming;
import java.rmi.NotBoundException;
import java.rmi.RemoteException;
import java.util.Map;
import java.util.WeakHashMap;
public interface Remote extends java.rmi.Remote {
public Remote map(Function f) throws RemoteException;
public Remote flatMap(Function> f) throws RemoteException;
public T get() throws RemoteException;
static final Factory factory = new Factory();
static class Factory implements RemoteFactory {
final Map, Reference>> cache = new WeakHashMap<>();
public Remote apply(T value) throws RemoteException {
final Remote obj = new RemoteImpl<>(value);
cache.put(obj, new WeakReference<>(obj));
return obj;
}
Remote> replace(Remote> obj) {
final Reference> w = cache.get(obj);
return w == null ? obj : w.get();
}
public void rebind(String name, T value) throws RemoteException, MalformedURLException {
Naming.rebind(name, apply(value));
}
@SuppressWarnings("unchecked")
public Remote lookup(String name) throws MalformedURLException, RemoteException, NotBoundException {
return (Remote)Naming.lookup(name);
}
}
public static Remote apply(T value) throws RemoteException {
return factory.apply(value);
}
public static void rebind(String name, T value) throws RemoteException, MalformedURLException {
factory.rebind(name, value);
}
public static Remote lookup(String name) throws MalformedURLException, RemoteException, NotBoundException {
return factory.lookup(name);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy