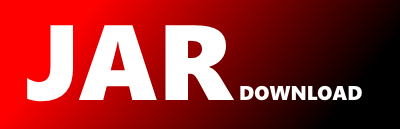
remote.server.RemoteImpl_Stub Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of remote4j Show documentation
Show all versions of remote4j Show documentation
Thin functional layer on top of RMI.
package remote.server;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectStreamException;
import java.io.Serializable;
import java.rmi.RemoteException;
import remote.Function;
import remote.Remote;
@SuppressWarnings("serial")
public class RemoteImpl_Stub extends RemoteObject implements Remote, Serializable {
private transient RemoteFactory factory;
private final String id;
private boolean state;
String getId() {
return id;
}
void setState(final boolean state) {
this.state = state;
}
RemoteImpl_Stub(final String id, final long num, final RemoteFactory factory) {
super(num);
this.id = id;
this.factory = factory;
}
@Override
@SuppressWarnings("unchecked")
public Remote map(Function f) throws RemoteException {
return (Remote) factory.invoke(id, getNum(), "map", new Class>[] {Function.class}, new Object[] {f});
}
@Override
@SuppressWarnings("unchecked")
public Remote flatMap(Function> f) throws RemoteException {
return (Remote) factory.invoke(id, getNum(), "flatMap", new Class>[] {Function.class}, new Object[] {f});
}
@Override
@SuppressWarnings("unchecked")
public T get() throws RemoteException {
return (T) factory.invoke(id, getNum(), "get", new Class>[] {}, new Object[] {});
}
private void readObject(final ObjectInputStream in) throws IOException, ClassNotFoundException {
in.defaultReadObject();
factory = ((InputStream) in).getFactory();
}
private Object readResolve() throws ObjectStreamException {
return factory.replace(this);
}
@Override
public String toString() {
return id + ":" + super.toString();
}
@Override
protected void finalize() {
if (state) {
factory.getClient().clean(id, getNum());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy