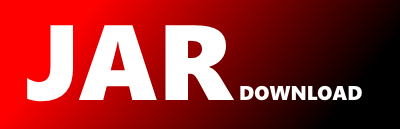
com.github.rkmk.mapper.FieldHelper Maven / Gradle / Ivy
package com.github.rkmk.mapper;
import java.lang.reflect.Field;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import static java.util.Objects.nonNull;
public class FieldHelper {
public static void set(Field field, Object object, Object value) {
field.setAccessible(true);
try {
field.set(object, value);
} catch (IllegalAccessException e) {
throw new IllegalArgumentException(String.format("The field %s is not accessible",field.getName()), e);
}
}
public static Object get(Field field, Object object) {
field.setAccessible(true);
try {
return field.get(object);
} catch (IllegalAccessException e) {
throw new IllegalArgumentException(String.format("The field %s is not accessible",field.getName()), e);
}
}
public static T getInstance(Class type) {
try {
return type.newInstance();
}
catch (Exception e) {
throw new IllegalArgumentException(String.format("A bean, %s, was mapped " +
"which was not instantiable", type.getName()), e);
}
}
public static Class> getParameterisedReturnType(Field field){
Class> result = null ;
Type genericFieldType = field.getGenericType();
if(genericFieldType instanceof ParameterizedType){
ParameterizedType aType = (ParameterizedType) genericFieldType;
Type[] fieldArgTypes = aType.getActualTypeArguments();
result = fieldArgTypes.length >0 ? (Class)fieldArgTypes[0] : null ;
}
if(nonNull(result)) {
return result;
}
throw new IllegalArgumentException(String.format("The field type %s is not inferrable",field.getName()));
}
public static List getFields(Class> type) {
List result = new ArrayList<>();
Class> clazz = type;
while(clazz.getSuperclass() != null) {
result.addAll(Arrays.asList(clazz.getDeclaredFields()));
clazz = clazz.getSuperclass();
}
return result;
}
public static Object accessField(String fieldName, O o) {
Field field = null;
try {
field = o.getClass().getDeclaredField(fieldName);
} catch (NoSuchFieldException e) {
throw new IllegalArgumentException("The field must not be null");
}
if (field == null) {
throw new IllegalArgumentException("The field must not be null");
}
field.setAccessible(true);
try {
return field.get(o);
} catch (IllegalAccessException e) {
throw new IllegalArgumentException("The field not accessible");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy