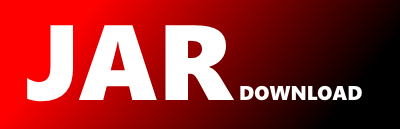
com.github.rmannibucau.asciidoctor.backend.DocumentVisitor Maven / Gradle / Ivy
package com.github.rmannibucau.asciidoctor.backend;
import java.util.Map;
import java.util.function.Function;
import java.util.function.Supplier;
import org.asciidoctor.ast.Block;
import org.asciidoctor.ast.Cell;
import org.asciidoctor.ast.DescriptionList;
import org.asciidoctor.ast.Document;
import org.asciidoctor.ast.List;
import org.asciidoctor.ast.Section;
import org.asciidoctor.ast.Table;
public interface DocumentVisitor {
String onDocument(Document document, String transform, Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy