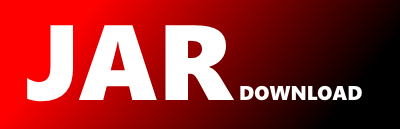
org.rx.core.Container Maven / Gradle / Ivy
package org.rx.core;
import lombok.NonNull;
import lombok.SneakyThrows;
import org.rx.exception.InvalidException;
import java.util.Collections;
import java.util.Map;
import java.util.WeakHashMap;
import java.util.concurrent.ConcurrentHashMap;
import static org.rx.core.Constants.NON_UNCHECKED;
@SuppressWarnings(NON_UNCHECKED)
public final class Container {
static final Map, Object> HOLDER = new ConcurrentHashMap<>(8);
//不要放值类型, ReferenceQueue、ConcurrentMap> 不准
static final Map WEAK_MAP = Collections.synchronizedMap(new WeakHashMap<>());
@SneakyThrows
public static T getOrDefault(Class type, Class extends T> defType) {
T instance = (T) HOLDER.get(type);
if (instance == null) {
Class.forName(type.getName());
instance = (T) HOLDER.get(type);
if (instance == null) {
return get(defType);
}
}
return instance;
}
@SneakyThrows
public static T get(Class type) {
T instance = (T) HOLDER.get(type);
if (instance == null) {
Class.forName(type.getName());
instance = (T) HOLDER.get(type);
if (instance == null) {
throw new InvalidException("Bean {} not registered", type.getName());
}
}
return instance;
}
public static void register(@NonNull Class type, @NonNull T instance) {
HOLDER.put(type, instance);
}
public static void unregister(Class type) {
HOLDER.remove(type);
}
static Map weakMap() {
return WEAK_MAP;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy