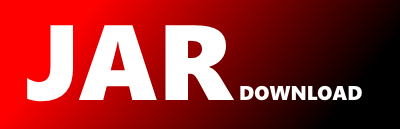
com.github.romanqed.jutils.util.Checks Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jutils Show documentation
Show all versions of jutils Show documentation
A set of simple utilities for implementing missing functionality in different versions of Java.
package com.github.romanqed.jutils.util;
import java.util.Objects;
import java.util.concurrent.Callable;
import java.util.function.Consumer;
import java.util.function.Predicate;
public class Checks {
public static T requireNonException(Callable expression, Callable def) {
Objects.requireNonNull(expression);
Objects.requireNonNull(def);
try {
return expression.call();
} catch (Exception e) {
try {
return def.call();
} catch (Exception defException) {
throw new IllegalStateException("Exception when calling def!", defException);
}
}
}
public static T requireNonNullElse(T object, T def) {
if (object == null) {
return Objects.requireNonNull(def);
}
return object;
}
public static T requireCorrectValue(T object, Predicate predicate) {
if (!predicate.test(object)) {
throw new IllegalArgumentException("Incorrect value!");
}
return object;
}
public static T requireCorrectValueElse(T object, Predicate predicate, T def) {
if (!predicate.test(object)) {
return requireCorrectValue(def, predicate);
}
return object;
}
public static String requireNonEmptyString(String object) {
return requireCorrectValue(object, (string) -> Objects.nonNull(object) && !string.isEmpty());
}
public static T safetyCall(Callable callable, Consumer failure) {
try {
return callable.call();
} catch (Exception e) {
if (failure != null) {
failure.accept(e);
}
return null;
}
}
public static void safetyRun(Runnable runnable, Consumer failure) {
try {
runnable.run();
} catch (Exception e) {
if (failure != null) {
failure.accept(e);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy