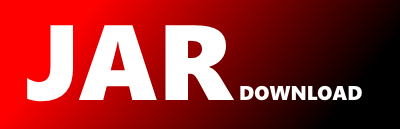
pers.zr.opensource.magic.dao.action.ConditionalAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of magic-dao Show documentation
Show all versions of magic-dao Show documentation
simplify to access database with java.
package pers.zr.opensource.magic.dao.action;
import org.springframework.util.CollectionUtils;
import pers.zr.opensource.magic.dao.constants.ConditionType;
import pers.zr.opensource.magic.dao.constants.MatchType;
import pers.zr.opensource.magic.dao.matcher.Matcher;
import pers.zr.opensource.magic.dao.shard.TableShardHandler;
import pers.zr.opensource.magic.dao.shard.TableShardStrategy;
import java.util.*;
/**
* Created by zhurong on 2016/4/30.
*/
public abstract class ConditionalAction extends Action {
private Collection conditions = new ArrayList();
private String conSql = null;
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy