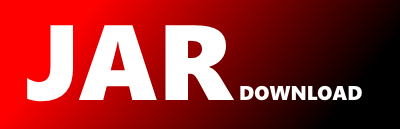
com.royken.generic.dao.impl.GenericDao Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of genericDao Show documentation
Show all versions of genericDao Show documentation
A library that provides CRUD and find all methods with JPA
The newest version!
package com.royken.generic.dao.impl;
import com.royken.generic.dao.DataAccessException;
import com.royken.generic.dao.IGenericDao;
import java.io.Serializable;
import java.lang.reflect.ParameterizedType;
import java.util.List;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
/**
* @author KENFACK Valmy-Roi
* @param
* @param
*/
public class GenericDao implements IGenericDao {
private Class entityClass;
@PersistenceContext
private EntityManager manager;
public GenericDao() {
this.entityClass = (Class) ((ParameterizedType) getClass().getGenericSuperclass()).getActualTypeArguments()[0];
}
public Class getEntityClass() {
return this.entityClass;
}
public EntityManager getManager() throws DataAccessException {
if (this.manager == null) {
throw new DataAccessException("Le gestionnaire des entit\u00e9s n'est pas encore d\u00e9fini");
}
return this.manager;
}
public void setManager(EntityManager manager) throws DataAccessException {
this.manager = manager;
}
public T create(T newInstance) throws DataAccessException{
try {
this.getManager().persist(newInstance);
return newInstance;
} catch (Exception e) {
}
return null;
}
public T findById(ID id) throws DataAccessException{
try {
return (T)this.getManager().find(this.entityClass, id);
} catch (Exception e) {
}
return null;
}
public T update(T transientObject) throws DataAccessException{
try {
return (T)this.getManager().merge(transientObject);
} catch (Exception e) {
}
return null;
}
public void delete(T persistentObject) throws DataAccessException{
this.getManager().remove(persistentObject);
}
public List findAll() throws DataAccessException {
return this.getManager().createQuery(" select u from " + this.entityClass.getName() + " u").getResultList();
}
public List findRange(int begin, int end) throws DataAccessException {
return this.getManager().createQuery(" select u from " + this.entityClass.getName() + " u").setMaxResults(end - begin + 1).setFirstResult(begin).getResultList();
}
public Long count() throws DataAccessException {
return (Long)(this.getManager().createQuery(" select count(*) from " + this.entityClass.getName() ).getResultList().get(0));
// return ((Long) q.getSingleResult()).intValue();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy