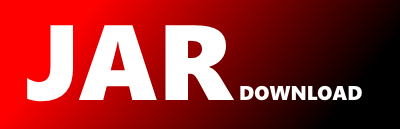
cc.mallet.cluster.neighbor_evaluator.RandomEvaluator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mallet Show documentation
Show all versions of mallet Show documentation
MALLET is a Java-based package for statistical natural language processing,
document classification, clustering, topic modeling, information extraction,
and other machine learning applications to text.
The newest version!
package cc.mallet.cluster.neighbor_evaluator;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.Serializable;
import cc.mallet.util.Randoms;
/**
* Randomly scores {@link Neighbor}s.
*
* @author "Aron Culotta"
* @version 1.0
* @since 1.0
* @see NeighborEvaluator
*/
public class RandomEvaluator implements NeighborEvaluator, Serializable {
Randoms random;
public RandomEvaluator (Randoms random) {
this.random = random;
}
/**
*
* @param neighbor
* @return A higher score indicates that the modified Clustering is preferred.
*/
public double evaluate (Neighbor neighbor) {
return random.nextUniform(0, 1);
}
/**
*
* @param neighbors
* @return One score per neighbor. A higher score indicates that the
* modified Clustering is preferred.
*
*/
public double[] evaluate (Neighbor[] neighbors) {
double[] scores = new double[neighbors.length];
for (int i = 0; i < neighbors.length; i++)
scores[i] = evaluate(neighbors[i]);
return scores;
}
/**
* Reset the state of the evaluator.
*/
public void reset () {}
// SERIALIZATION
private static final long serialVersionUID = 1;
private static final int CURRENT_SERIAL_VERSION = 1;
private void writeObject (ObjectOutputStream out) throws IOException {
out.defaultWriteObject ();
out.writeInt (CURRENT_SERIAL_VERSION);
}
private void readObject (ObjectInputStream in) throws IOException, ClassNotFoundException {
in.defaultReadObject ();
int version = in.readInt ();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy