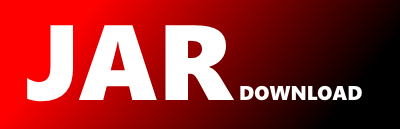
com.github.ruediste.salta.standard.DefaultCreationRecipeBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of salta-core Show documentation
Show all versions of salta-core Show documentation
Core of the Salta Framework. Provides most of the infrastructure, but no API
package com.github.ruediste.salta.standard;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.Optional;
import java.util.function.Function;
import java.util.function.Supplier;
import org.objectweb.asm.commons.GeneratorAdapter;
import com.github.ruediste.salta.core.RecipeCreationContext;
import com.github.ruediste.salta.core.SaltaException;
import com.github.ruediste.salta.core.compile.MethodCompilationContext;
import com.github.ruediste.salta.core.compile.SupplierRecipe;
import com.github.ruediste.salta.standard.config.StandardInjectorConfiguration;
import com.github.ruediste.salta.standard.recipe.RecipeEnhancer;
import com.google.common.reflect.TypeToken;
public class DefaultCreationRecipeBuilder {
public Supplier> constructionRecipeSupplier;
public Function> enhancersSupplier;
public DefaultCreationRecipeBuilder(StandardInjectorConfiguration config,
TypeToken> boundType) {
constructionRecipeSupplier = () -> {
Optional> tmp = config
.createConstructionRecipe(boundType);
if (!tmp.isPresent())
throw new SaltaException("Cannot find construction rule for "
+ boundType);
return tmp.get();
};
enhancersSupplier = ctx -> config.createEnhancers(ctx, boundType);
}
/**
* Build the recipe.
*/
public SupplierRecipe build(RecipeCreationContext ctx) {
// create seed recipe
SupplierRecipe seedRecipe = constructionRecipeSupplier.get().apply(ctx);
// apply enhancers
List enhancers = new ArrayList<>(
enhancersSupplier.apply(ctx));
SupplierRecipe innerRecipe = applyEnhancers(seedRecipe, enhancers);
return innerRecipe;
}
public static SupplierRecipe applyEnhancers(SupplierRecipe seedRecipe,
List enhancers) {
Collections.reverse(enhancers);
SupplierRecipe innerRecipe = createInnerRecipe(enhancers.iterator(),
seedRecipe);
return innerRecipe;
}
private static SupplierRecipe createInnerRecipe(
Iterator iterator, SupplierRecipe seedRecipe) {
if (!iterator.hasNext())
return seedRecipe;
// consume an enhancer
RecipeEnhancer enhancer = iterator.next();
// create a recipe for the rest of the chain
SupplierRecipe innerRecipe = createInnerRecipe(iterator, seedRecipe);
// return recipe
return new SupplierRecipe() {
@Override
protected Class> compileImpl(GeneratorAdapter mv,
MethodCompilationContext ctx) {
return enhancer.compile(ctx, innerRecipe);
}
};
}
public static SupplierRecipe applyEnhancers(SupplierRecipe seedRecipe,
StandardInjectorConfiguration config, RecipeCreationContext ctx,
TypeToken> type) {
return applyEnhancers(seedRecipe, config.createEnhancers(ctx, type));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy