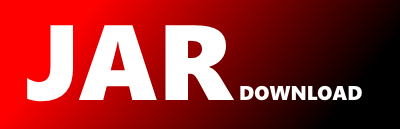
com.github.ruediste.salta.standard.ScopeImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of salta-core Show documentation
Show all versions of salta-core Show documentation
Core of the Salta Framework. Provides most of the infrastructure, but no API
package com.github.ruediste.salta.standard;
import java.util.function.Supplier;
import org.objectweb.asm.Type;
import org.objectweb.asm.commons.GeneratorAdapter;
import org.objectweb.asm.commons.Method;
import com.github.ruediste.salta.core.Binding;
import com.github.ruediste.salta.core.CompiledSupplier;
import com.github.ruediste.salta.core.RecipeCreationContext;
import com.github.ruediste.salta.core.Scope;
import com.github.ruediste.salta.core.compile.MethodCompilationContext;
import com.github.ruediste.salta.core.compile.SupplierRecipe;
import com.google.common.reflect.TypeToken;
public class ScopeImpl implements Scope {
private ScopeHandler handler;
public interface ScopeHandler {
/**
* Scope the given binding
*
* @param supplier
* supplier of the unscoped instance
* @param binding
* binding beeing scoped
* @param requestedType
* type beeing requested by the injection point
* @return
*/
Supplier
© 2015 - 2024 Weber Informatics LLC | Privacy Policy