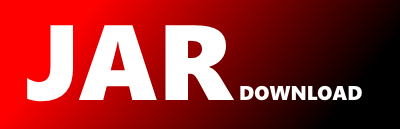
com.github.ruediste.salta.standard.DependencyKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of salta-core Show documentation
Show all versions of salta-core Show documentation
Core of the Salta Framework. Provides most of the infrastructure, but no API
package com.github.ruediste.salta.standard;
import java.lang.annotation.Annotation;
import java.lang.reflect.AnnotatedElement;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import com.github.ruediste.salta.core.CoreDependencyKey;
import com.github.ruediste.salta.standard.binder.AnnotationProxy;
import com.google.common.reflect.TypeToken;
/**
* The type and Qualifiers to lookup an instance.
*/
public class DependencyKey extends CoreDependencyKey {
private final TypeToken type;
private Class rawType;
private final Map, Annotation> annotations;
private final int hashCode;
public static DependencyKey of(TypeToken type) {
return new DependencyKey<>(type, null, new HashMap<>());
}
public static DependencyKey of(TypeToken type, Class rawType) {
return new DependencyKey<>(type, rawType, new HashMap<>());
}
public static DependencyKey of(Class type) {
return new DependencyKey<>(TypeToken.of(type), type, new HashMap<>());
}
private DependencyKey(TypeToken type, Class rawType,
Map, Annotation> annotations) {
this.type = type;
this.rawType = rawType;
this.annotations = annotations;
// don't use Objects.hash() for performance reason
this.hashCode = type.hashCode() + 31 * annotations.hashCode();
}
@Override
public TypeToken getType() {
return type;
}
@Override
public AnnotatedElement getAnnotatedElement() {
return new AnnotatedElement() {
@Override
public Annotation[] getDeclaredAnnotations() {
return getAnnotations();
}
@Override
public Annotation[] getAnnotations() {
return annotations.values().toArray(new Annotation[] {});
}
@SuppressWarnings("unchecked")
@Override
public A getAnnotation(Class annotationClass) {
return (A) annotations.get(annotationClass);
}
};
}
public Map, Annotation> getAnnotations() {
return Collections.unmodifiableMap(annotations);
}
@SafeVarargs
public final DependencyKey withAnnotations(Class extends Annotation>... cls) {
Annotation[] annotations = new Annotation[cls.length];
for (int i = 0; i < cls.length; i++) {
annotations[i] = AnnotationProxy.newProxy(cls[i]);
}
return withAnnotations(annotations);
}
public DependencyKey withAnnotations(Annotation... additionalAnnotations) {
HashMap, Annotation> tmp = new HashMap<>(annotations);
for (Annotation a : additionalAnnotations) {
if (a == null)
continue;
tmp.put(a.annotationType(), a);
}
return new DependencyKey<>(type, rawType, tmp);
}
@Override
public int hashCode() {
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (obj == this)
return true;
if (obj == null)
return false;
if (!Objects.equals(getClass(), obj.getClass()))
return false;
DependencyKey> other = (DependencyKey>) obj;
return Objects.equals(type, other.type) && Objects.equals(annotations, other.annotations);
}
@SuppressWarnings("unchecked")
@Override
public Class getRawType() {
// no synchronization required. In the worst case, multiple
// threads will compute the value and overwrite each other's result,
// which is No Problem
if (rawType == null)
rawType = (Class) type.getRawType();
return rawType;
}
@Override
public String toString() {
String result = getType().toString();
if (!annotations.values().isEmpty())
result += annotations.values();
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy