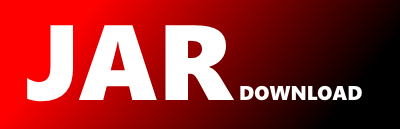
sparksoniq.jsoniq.runtime.iterator.functions.arrays.ArrayFlattenFunctionIterator Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Authors: Stefan Irimescu, Can Berker Cikis
*
*/
package sparksoniq.jsoniq.runtime.iterator.functions.arrays;
import sparksoniq.exceptions.IteratorFlowException;
import sparksoniq.jsoniq.runtime.iterator.RuntimeIterator;
import sparksoniq.jsoniq.runtime.iterator.functions.base.LocalFunctionCallIterator;
import sparksoniq.jsoniq.runtime.metadata.IteratorMetadata;
import sparksoniq.semantics.DynamicContext;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
import java.util.Queue;
import org.rumbledb.api.Item;
public class ArrayFlattenFunctionIterator extends LocalFunctionCallIterator {
private static final long serialVersionUID = 1L;
private RuntimeIterator _iterator;
private Queue- _nextResults; // queue that holds the results created by the current item in inspection
public ArrayFlattenFunctionIterator(List
arguments, IteratorMetadata iteratorMetadata) {
super(arguments, iteratorMetadata);
}
@Override
public Item next() {
if (_hasNext == true) {
Item result = _nextResults.remove(); // save the result to be returned
if (_nextResults.isEmpty()) {
// if there are no more results left in the queue, trigger calculation for the next result
setNextResult();
}
return result;
}
throw new IteratorFlowException(RuntimeIterator.FLOW_EXCEPTION_MESSAGE + " FLATTEN function",
getMetadata());
}
@Override
public void open(DynamicContext context) {
super.open(context);
_iterator = this._children.get(0);
_iterator.open(context);
_nextResults = new LinkedList<>();
setNextResult();
}
public void setNextResult() {
while (_iterator.hasNext()) {
Item item = _iterator.next();
List- singleItemList = new ArrayList<>();
singleItemList.add(item);
flatten(singleItemList);
if (!(_nextResults.isEmpty())) {
break;
}
}
if (_nextResults.isEmpty()) {
this._hasNext = false;
_iterator.close();
} else {
this._hasNext = true;
}
}
public void flatten(List
- items) {
for (Item item : items) {
if (item.isArray()) {
flatten(item.getItems());
} else {
_nextResults.add(item);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy