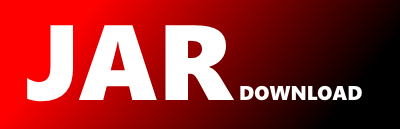
com.github.rvesse.airline.help.common.AbstractCommandUsageGenerator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of airline Show documentation
Show all versions of airline Show documentation
Java library provided an annotation-based framework for parsing Git like command line structures
/**
* Copyright (C) 2010-16 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.rvesse.airline.help.common;
import java.io.IOException;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
import java.util.Map.Entry;
import com.github.rvesse.airline.Channels;
import com.github.rvesse.airline.help.CommandUsageGenerator;
import com.github.rvesse.airline.help.UsageHelper;
import com.github.rvesse.airline.help.sections.HelpHint;
import com.github.rvesse.airline.help.sections.HelpSection;
import com.github.rvesse.airline.model.CommandMetadata;
import com.github.rvesse.airline.model.OptionMetadata;
import com.github.rvesse.airline.model.ParserMetadata;
import com.github.rvesse.airline.utils.comparators.HelpSectionComparator;
/**
* Abstract command usage generator
*
*/
public abstract class AbstractCommandUsageGenerator extends AbstractUsageGenerator implements CommandUsageGenerator {
private final Comparator super Entry> exitCodeComparator;
public AbstractCommandUsageGenerator() {
this(UsageHelper.DEFAULT_OPTION_COMPARATOR);
}
public AbstractCommandUsageGenerator(boolean includeHidden) {
this(UsageHelper.DEFAULT_HINT_COMPARATOR, UsageHelper.DEFAULT_OPTION_COMPARATOR,
UsageHelper.DEFAULT_EXIT_CODE_COMPARATOR, includeHidden);
}
public AbstractCommandUsageGenerator(Comparator super OptionMetadata> optionComparator) {
this(UsageHelper.DEFAULT_HINT_COMPARATOR, optionComparator, UsageHelper.DEFAULT_EXIT_CODE_COMPARATOR, false);
}
public AbstractCommandUsageGenerator(Comparator super OptionMetadata> optionComparator, boolean includeHidden) {
this(UsageHelper.DEFAULT_HINT_COMPARATOR, optionComparator, UsageHelper.DEFAULT_EXIT_CODE_COMPARATOR,
includeHidden);
}
public AbstractCommandUsageGenerator(Comparator super HelpHint> hintComparator,
Comparator super OptionMetadata> optionComparator,
Comparator super Entry> exitCodeComparator, boolean includeHidden) {
super(hintComparator, optionComparator, UsageHelper.DEFAULT_COMMAND_COMPARATOR, includeHidden);
this.exitCodeComparator = exitCodeComparator;
}
@Override
public void usage(CommandMetadata command, ParserMetadata parserConfig, OutputStream output) throws IOException {
usage(null, null, command.getName(), command, parserConfig, output);
}
@Override
public void usage(String programName, String[] groupNames, String commandName, CommandMetadata command,
ParserMetadata parserConfig) throws IOException {
usage(programName, groupNames, commandName, command, parserConfig, Channels.output());
}
/**
* Sorts the exit codes assuming a non-null comparator was provided at
* instantiation time
*
* @param exitCodes
* Exit codes
* @return Sorted exit codes
*/
protected List> sortExitCodes(List> exitCodes) {
if (exitCodeComparator != null) {
exitCodes = new ArrayList<>(exitCodes);
Collections.sort(exitCodes, exitCodeComparator);
}
return exitCodes;
}
/**
* Finds the help sections
*
* @param command
* Command meta-data
* @param preSections
* Sections that should be placed before base content
* @param postSections
* Sections that should be placed after base content
*/
protected void findHelpSections(CommandMetadata command, List preSections,
List postSections) {
for (HelpSection section : command.getHelpSections()) {
if (section.suggestedOrder() < 0) {
preSections.add(section);
} else {
postSections.add(section);
}
}
HelpSectionComparator comparator = new HelpSectionComparator();
Collections.sort(preSections, comparator);
Collections.sort(postSections, comparator);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy