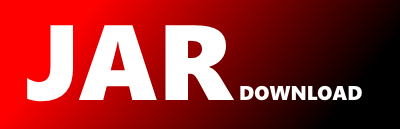
com.exacttarget.fuelsdk.internal.SaveOption Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fuelsdk Show documentation
Show all versions of fuelsdk Show documentation
Salesforce Marketing Cloud Java SDK
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy