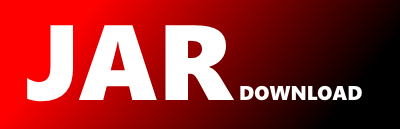
com.exacttarget.fuelsdk.internal.SendSummary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fuelsdk Show documentation
Show all versions of fuelsdk Show documentation
Salesforce Marketing Cloud Java SDK
package com.exacttarget.fuelsdk.internal;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
import org.apache.commons.lang.builder.ToStringBuilder;
import org.apache.cxf.xjc.runtime.JAXBToStringStyle;
/**
* Java class for SendSummary complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="SendSummary">
* <complexContent>
* <extension base="{http://exacttarget.com/wsdl/partnerAPI}APIObject">
* <sequence>
* <element name="AccountID" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="AccountName" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="AccountEmail" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="IsTestAccount" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="SendID" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="DeliveredTime" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="TotalSent" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="Transactional" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="NonTransactional" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "SendSummary", propOrder = {
"accountID",
"accountName",
"accountEmail",
"isTestAccount",
"sendID",
"deliveredTime",
"totalSent",
"transactional",
"nonTransactional"
})
public class SendSummary
extends APIObject
{
@XmlElement(name = "AccountID")
protected Integer accountID;
@XmlElement(name = "AccountName")
protected String accountName;
@XmlElement(name = "AccountEmail")
protected String accountEmail;
@XmlElement(name = "IsTestAccount")
protected Boolean isTestAccount;
@XmlElement(name = "SendID")
protected Integer sendID;
@XmlElement(name = "DeliveredTime")
protected String deliveredTime;
@XmlElement(name = "TotalSent")
protected Integer totalSent;
@XmlElement(name = "Transactional")
protected Integer transactional;
@XmlElement(name = "NonTransactional")
protected Integer nonTransactional;
/**
* Gets the value of the accountID property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getAccountID() {
return accountID;
}
/**
* Sets the value of the accountID property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setAccountID(Integer value) {
this.accountID = value;
}
/**
* Gets the value of the accountName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAccountName() {
return accountName;
}
/**
* Sets the value of the accountName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAccountName(String value) {
this.accountName = value;
}
/**
* Gets the value of the accountEmail property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAccountEmail() {
return accountEmail;
}
/**
* Sets the value of the accountEmail property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAccountEmail(String value) {
this.accountEmail = value;
}
/**
* Gets the value of the isTestAccount property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean getIsTestAccount() {
return isTestAccount;
}
/**
* Sets the value of the isTestAccount property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setIsTestAccount(Boolean value) {
this.isTestAccount = value;
}
/**
* Gets the value of the sendID property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getSendID() {
return sendID;
}
/**
* Sets the value of the sendID property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setSendID(Integer value) {
this.sendID = value;
}
/**
* Gets the value of the deliveredTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDeliveredTime() {
return deliveredTime;
}
/**
* Sets the value of the deliveredTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDeliveredTime(String value) {
this.deliveredTime = value;
}
/**
* Gets the value of the totalSent property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getTotalSent() {
return totalSent;
}
/**
* Sets the value of the totalSent property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setTotalSent(Integer value) {
this.totalSent = value;
}
/**
* Gets the value of the transactional property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getTransactional() {
return transactional;
}
/**
* Sets the value of the transactional property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setTransactional(Integer value) {
this.transactional = value;
}
/**
* Gets the value of the nonTransactional property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getNonTransactional() {
return nonTransactional;
}
/**
* Sets the value of the nonTransactional property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setNonTransactional(Integer value) {
this.nonTransactional = value;
}
/**
* Generates a String representation of the contents of this type.
* This is an extension method, produced by the 'ts' xjc plugin
*
*/
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, JAXBToStringStyle.DEFAULT_STYLE);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy