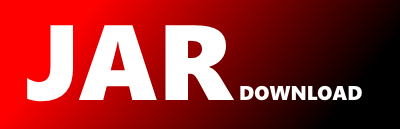
com.exacttarget.fuelsdk.internal.ValidationResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fuelsdk Show documentation
Show all versions of fuelsdk Show documentation
Salesforce Marketing Cloud Java SDK
The newest version!
package com.exacttarget.fuelsdk.internal;
import java.util.Date;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.apache.commons.lang.builder.ToStringBuilder;
import org.apache.cxf.xjc.runtime.JAXBToStringStyle;
/**
* Java class for ValidationResult complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="ValidationResult">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Subscriber" type="{http://exacttarget.com/wsdl/partnerAPI}Subscriber" minOccurs="0"/>
* <element name="CheckTime" type="{http://www.w3.org/2001/XMLSchema}dateTime" minOccurs="0"/>
* <element name="CheckTimeUTC" type="{http://www.w3.org/2001/XMLSchema}dateTime" minOccurs="0"/>
* <element name="IsResultValid" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="IsSpam" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="Score" type="{http://www.w3.org/2001/XMLSchema}double" minOccurs="0"/>
* <element name="Threshold" type="{http://www.w3.org/2001/XMLSchema}double" minOccurs="0"/>
* <element name="Message" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ValidationResult", propOrder = {
"subscriber",
"checkTime",
"checkTimeUTC",
"isResultValid",
"isSpam",
"score",
"threshold",
"message"
})
public class ValidationResult {
@XmlElement(name = "Subscriber")
protected Subscriber subscriber;
@XmlElement(name = "CheckTime", type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected Date checkTime;
@XmlElement(name = "CheckTimeUTC", type = String.class)
@XmlJavaTypeAdapter(Adapter1 .class)
@XmlSchemaType(name = "dateTime")
protected Date checkTimeUTC;
@XmlElement(name = "IsResultValid")
protected Boolean isResultValid;
@XmlElement(name = "IsSpam")
protected Boolean isSpam;
@XmlElement(name = "Score")
protected Double score;
@XmlElement(name = "Threshold")
protected Double threshold;
@XmlElement(name = "Message")
protected String message;
/**
* Gets the value of the subscriber property.
*
* @return
* possible object is
* {@link Subscriber }
*
*/
public Subscriber getSubscriber() {
return subscriber;
}
/**
* Sets the value of the subscriber property.
*
* @param value
* allowed object is
* {@link Subscriber }
*
*/
public void setSubscriber(Subscriber value) {
this.subscriber = value;
}
/**
* Gets the value of the checkTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public Date getCheckTime() {
return checkTime;
}
/**
* Sets the value of the checkTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCheckTime(Date value) {
this.checkTime = value;
}
/**
* Gets the value of the checkTimeUTC property.
*
* @return
* possible object is
* {@link String }
*
*/
public Date getCheckTimeUTC() {
return checkTimeUTC;
}
/**
* Sets the value of the checkTimeUTC property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCheckTimeUTC(Date value) {
this.checkTimeUTC = value;
}
/**
* Gets the value of the isResultValid property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean getIsResultValid() {
return isResultValid;
}
/**
* Sets the value of the isResultValid property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setIsResultValid(Boolean value) {
this.isResultValid = value;
}
/**
* Gets the value of the isSpam property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean getIsSpam() {
return isSpam;
}
/**
* Sets the value of the isSpam property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setIsSpam(Boolean value) {
this.isSpam = value;
}
/**
* Gets the value of the score property.
*
* @return
* possible object is
* {@link Double }
*
*/
public Double getScore() {
return score;
}
/**
* Sets the value of the score property.
*
* @param value
* allowed object is
* {@link Double }
*
*/
public void setScore(Double value) {
this.score = value;
}
/**
* Gets the value of the threshold property.
*
* @return
* possible object is
* {@link Double }
*
*/
public Double getThreshold() {
return threshold;
}
/**
* Sets the value of the threshold property.
*
* @param value
* allowed object is
* {@link Double }
*
*/
public void setThreshold(Double value) {
this.threshold = value;
}
/**
* Gets the value of the message property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMessage() {
return message;
}
/**
* Sets the value of the message property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMessage(String value) {
this.message = value;
}
/**
* Generates a String representation of the contents of this type.
* This is an extension method, produced by the 'ts' xjc plugin
*
*/
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, JAXBToStringStyle.DEFAULT_STYLE);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy