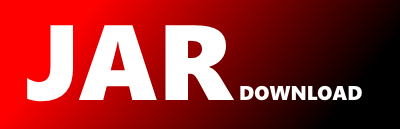
com.github.salomonbrys.kodein.lazy.kt Maven / Gradle / Ivy
package com.github.salomonbrys.kodein
class LazyKodein(private val _lazy: Lazy) : () -> Kodein {
override fun invoke() = _lazy.value
constructor(silentOverride: Boolean = false, init: Kodein.Builder.() -> Unit) : this(lazy { Kodein(silentOverride, init) })
}
inline fun _lazyFactory(tag: Any? = null, noinline kodein: () -> Kodein) : Lazy<(A) -> T> = lazy { kodein().factory(tag) }
inline fun _lazyProvider(tag: Any? = null, noinline kodein: () -> Kodein) : Lazy<() -> T> = lazy { kodein().provider(tag) }
inline fun _lazyInstance(tag: Any? = null, noinline kodein: () -> Kodein) : Lazy = lazy { kodein().instance(tag) }
/**
* To be used as a property delegate to inject a factory
*/
inline fun Kodein.lazyFactory(tag: Any? = null) : Lazy<(A) -> T> = _lazyFactory(tag) { this }
/**
* To be used as a property delegate to inject a provider
*/
inline fun Kodein.lazyProvider(tag: Any? = null) : Lazy<() -> T> = _lazyProvider(tag) { this }
/**
* To be used as a property delegate to inject an instance
*/
inline fun Kodein.lazyInstance(tag: Any? = null) : Lazy = _lazyInstance(tag) { this }
/**
* To be used as a property delegate to inject a factory
*/
inline fun Lazy.lazyFactory(tag: Any? = null) : Lazy<(A) -> T> = _lazyFactory(tag) { this.value }
/**
* To be used as a property delegate to inject a provider
*/
inline fun Lazy.lazyProvider(tag: Any? = null) : Lazy<() -> T> = _lazyProvider(tag) { this.value }
/**
* To be used as a property delegate to inject an instance
*/
inline fun Lazy.lazyInstance(tag: Any? = null) : Lazy = _lazyInstance(tag) { this.value }
/**
* To be used as a property delegate to inject a factory
*/
inline fun (() -> Kodein).lazyFactory(tag: Any? = null) : Lazy<(A) -> T> = _lazyFactory(tag) { this() }
/**
* To be used as a property delegate to inject a provider
*/
inline fun (() -> Kodein).lazyProvider(tag: Any? = null) : Lazy<() -> T> = _lazyProvider(tag) { this() }
/**
* To be used as a property delegate to inject an instance
*/
inline fun (() -> Kodein).lazyInstance(tag: Any? = null) : Lazy = _lazyInstance(tag) { this() }
fun Lazy<(A) -> T>.toLazyProvider(arg: A): Lazy<() -> T> = lazy { { value(arg) } }
fun Lazy<(A) -> T>.toLazyInstance(arg: A): Lazy = lazy { value(arg) }
© 2015 - 2025 Weber Informatics LLC | Privacy Policy