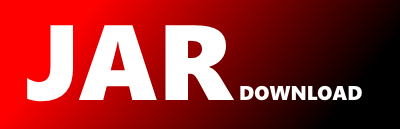
com.github.sanity4j.gen.pmd_4_2_1.Violation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sanity4j Show documentation
Show all versions of sanity4j Show documentation
Sanity4J was created to simplify running multiple static code
analysis tools on the Java projects. It provides a single entry
point to run all the selected tools and produce a consolidated
report, which presents all findings in an easily accessible
manner.
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.11
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2017.05.24 at 08:49:56 AM AEST
//
package com.github.sanity4j.gen.pmd_4_2_1;
import java.math.BigInteger;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlValue;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <simpleContent>
* <extension base="<http://www.w3.org/2001/XMLSchema>string">
* <attribute name="beginline" use="required" type="{http://www.w3.org/2001/XMLSchema}integer" />
* <attribute name="endline" use="required" type="{http://www.w3.org/2001/XMLSchema}integer" />
* <attribute name="begincolumn" use="required" type="{http://www.w3.org/2001/XMLSchema}integer" />
* <attribute name="endcolumn" use="required" type="{http://www.w3.org/2001/XMLSchema}integer" />
* <attribute name="rule" use="required" type="{http://www.w3.org/2001/XMLSchema}NMTOKEN" />
* <attribute name="ruleset" use="required" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="package" use="required" type="{http://www.w3.org/2001/XMLSchema}NMTOKEN" />
* <attribute name="class" type="{http://www.w3.org/2001/XMLSchema}string" />
* <attribute name="method" type="{http://www.w3.org/2001/XMLSchema}NMTOKEN" />
* <attribute name="externalInfoUrl" type="{http://www.w3.org/2001/XMLSchema}anyURI" />
* <attribute name="priority" use="required" type="{http://www.w3.org/2001/XMLSchema}integer" />
* <attribute name="variable" type="{http://www.w3.org/2001/XMLSchema}NMTOKEN" />
* </extension>
* </simpleContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"value"
})
@XmlRootElement(name = "violation")
public class Violation {
@XmlValue
protected String value;
@XmlAttribute(name = "beginline", required = true)
protected BigInteger beginline;
@XmlAttribute(name = "endline", required = true)
protected BigInteger endline;
@XmlAttribute(name = "begincolumn", required = true)
protected BigInteger begincolumn;
@XmlAttribute(name = "endcolumn", required = true)
protected BigInteger endcolumn;
@XmlAttribute(name = "rule", required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
protected String rule;
@XmlAttribute(name = "ruleset", required = true)
protected String ruleset;
@XmlAttribute(name = "package", required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
protected String _package;
@XmlAttribute(name = "class")
protected String className;
@XmlAttribute(name = "method")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
protected String method;
@XmlAttribute(name = "externalInfoUrl")
@XmlSchemaType(name = "anyURI")
protected String externalInfoUrl;
@XmlAttribute(name = "priority", required = true)
protected BigInteger priority;
@XmlAttribute(name = "variable")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
protected String variable;
/**
* Gets the value of the value property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getValue() {
return value;
}
/**
* Sets the value of the value property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setValue(String value) {
this.value = value;
}
/**
* Gets the value of the beginline property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getBeginline() {
return beginline;
}
/**
* Sets the value of the beginline property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setBeginline(BigInteger value) {
this.beginline = value;
}
/**
* Gets the value of the endline property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getEndline() {
return endline;
}
/**
* Sets the value of the endline property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setEndline(BigInteger value) {
this.endline = value;
}
/**
* Gets the value of the begincolumn property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getBegincolumn() {
return begincolumn;
}
/**
* Sets the value of the begincolumn property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setBegincolumn(BigInteger value) {
this.begincolumn = value;
}
/**
* Gets the value of the endcolumn property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getEndcolumn() {
return endcolumn;
}
/**
* Sets the value of the endcolumn property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setEndcolumn(BigInteger value) {
this.endcolumn = value;
}
/**
* Gets the value of the rule property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRule() {
return rule;
}
/**
* Sets the value of the rule property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRule(String value) {
this.rule = value;
}
/**
* Gets the value of the ruleset property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRuleset() {
return ruleset;
}
/**
* Sets the value of the ruleset property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRuleset(String value) {
this.ruleset = value;
}
/**
* Gets the value of the package property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPackage() {
return _package;
}
/**
* Sets the value of the package property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPackage(String value) {
this._package = value;
}
/**
* Gets the value of the className property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getClassName() {
return className;
}
/**
* Sets the value of the className property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setClassName(String value) {
this.className = value;
}
/**
* Gets the value of the method property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMethod() {
return method;
}
/**
* Sets the value of the method property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMethod(String value) {
this.method = value;
}
/**
* Gets the value of the externalInfoUrl property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getExternalInfoUrl() {
return externalInfoUrl;
}
/**
* Sets the value of the externalInfoUrl property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setExternalInfoUrl(String value) {
this.externalInfoUrl = value;
}
/**
* Gets the value of the priority property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getPriority() {
return priority;
}
/**
* Sets the value of the priority property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setPriority(BigInteger value) {
this.priority = value;
}
/**
* Gets the value of the variable property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVariable() {
return variable;
}
/**
* Sets the value of the variable property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVariable(String value) {
this.variable = value;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy