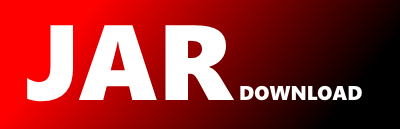
org.thavam.util.concurrent.blockingMapTester.BlockingMapTester Maven / Gradle / Ivy
The newest version!
/*x
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package org.thavam.util.concurrent.blockingMapTester;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Queue;
import java.util.concurrent.*;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.thavam.util.concurrent.blockingMap.BlockingHashMap;
import org.thavam.util.concurrent.blockingMap.BlockingMap;
/**
*
* @author Sarveswaran M
*/
public class BlockingMapTester {
private final Map referenceMap;
private final BlockingMap blockingMap;
private final Queue productionErrors
= new ConcurrentLinkedQueue();
private final Queue> comsumptionErrors
= new ConcurrentLinkedQueue>();
private List>> consumers;
private List> producers;
private final ExecutorService executor;
//these attributes need to be used from inner classes
//hence forced to be defined here
int i = 0;
int j = 0;
public BlockingMapTester() {
referenceMap = new ConcurrentHashMap();
for (i = 0; i < 100; i++) {
referenceMap.put(i, "Stringy " + i);
}
blockingMap = new BlockingHashMap();
executor = Executors.newCachedThreadPool();
}
void createConsumers() {
consumers = new ArrayList>>();
for (i = 0; i < 100; i++) {
consumers.add(new Callable>() {
final int key = i;
@Override
public Map.Entry call() {
Map.Entry entry = null;
try {
// System.out.println("taking " + i);
final String valueString = blockingMap.take(key);
System.out.println("blockingmap.take(" + key + ") = " + valueString);
entry = new Map.Entry() {
final Integer entryKey = key;
final String value = valueString;
@Override
public Integer getKey() {
return entryKey;
}
@Override
public String getValue() {
return value;
}
@Override
public String setValue(String value) {
throw new UnsupportedOperationException("Not supported yet.");
}
@Override
public String toString() {
return ("key,value : " + entryKey + ":" + value);
}
};
} catch (InterruptedException ex) {
Logger.getLogger(BlockingMapTester.class.getName()).log(Level.SEVERE, null, ex);
}
return entry;
}
});
}
}
void startConsuming() {
executor.submit(new FutureTask(new Callable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy