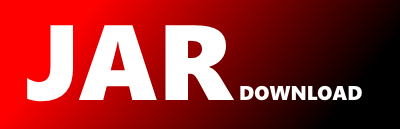
com.github.sarxos.xchange.ExchangeQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of exchange-rates-api Show documentation
Show all versions of exchange-rates-api Show documentation
Java API to access forex exchange rates via Yahoo YQL with fallback to OpenExchangeRates JSON
The newest version!
package com.github.sarxos.xchange;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.github.sarxos.xchange.impl.FetchOpenExchangeImpl;
import com.github.sarxos.xchange.impl.FetchYahooImpl;
public class ExchangeQuery {
/**
* I'm the logger.
*/
private static final Logger LOG = LoggerFactory.getLogger(ExchangeQuery.class);
/**
* Convert from currency.
*/
private Collection from;
/**
* Convert to currency.
*/
private String to;
public ExchangeQuery from(String... symbols) {
from = new ArrayList<>(Arrays.asList(symbols));
return this;
}
public ExchangeQuery from(Collection symbols) {
from = symbols;
return this;
}
public ExchangeQuery to(String symbol) {
to = symbol;
return this;
}
public Collection get() throws ExchangeException {
// return empty list if input is empty
if (from.isEmpty()) {
return Collections.emptyList();
}
// first, try Yahoo
try {
return new FetchYahooImpl().get(to, from);
} catch (ExchangeException e) {
LOG.error("Unable to get exchange data from Yahoo", e);
}
// then, if Yahoo failed, try OpenExchange
try {
return new FetchOpenExchangeImpl().get(to, from);
} catch (ExchangeException e) {
LOG.error("Unable to get exchange data from Yahoo", e);
}
return null;
}
public static void main(String[] args) throws ExchangeException {
for (ExchangeRate r : new ExchangeQuery()
.from("EUR", "JPY", "BGN", "PLN", "MXN", "ZAR", "LVL", "ZMK", "GBP")
.to("GBP")
.get()) {
System.out.println(r);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy