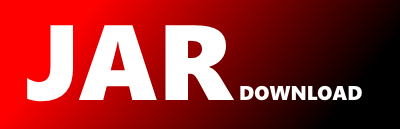
redis.clients.jedis.MultiKeyCommands Maven / Gradle / Ivy
Show all versions of jedis Show documentation
package redis.clients.jedis;
import java.util.List;
import java.util.Set;
public interface MultiKeyCommands {
Long del(String... keys);
Long unlink(String... keys);
Long exists(String... keys);
List blpop(int timeout, String... keys);
List brpop(int timeout, String... keys);
List blpop(String... args);
List brpop(String... args);
/**
* Returns all the keys matching the glob-style pattern. For example if
* you have in the database the keys "foo" and "foobar" the command "KEYS foo*" will return
* "foo foobar".
* Warning: consider this as a command that should be used in production environments with extreme care.
* It may ruin performance when it is executed against large databases.
* This command is intended for debugging and special operations, such as changing your keyspace layout.
* Don't use it in your regular application code.
* If you're looking for a way to find keys in a subset of your keyspace, consider using {@link #scan(String, ScanParams)} or sets.
*
* While the time complexity for this operation is O(N), the constant times are fairly low.
* For example, Redis running on an entry level laptop can scan a 1 million key database in 40 milliseconds.
*
* Glob style patterns examples:
*
* - h?llo will match hello hallo hhllo
*
- h*llo will match hllo heeeello
*
- h[ae]llo will match hello and hallo, but not hillo
*
*
* Use \ to escape special chars if you want to match them verbatim.
*
* Time complexity: O(n) (with n being the number of keys in the DB, and assuming keys and pattern
* of limited length)
* @param pattern
* @return Multi bulk reply
* @see Redis KEYS documentation
*/
Set keys(String pattern);
List mget(String... keys);
String mset(String... keysvalues);
Long msetnx(String... keysvalues);
String rename(String oldkey, String newkey);
Long renamenx(String oldkey, String newkey);
String rpoplpush(String srckey, String dstkey);
Set sdiff(String... keys);
Long sdiffstore(String dstkey, String... keys);
Set sinter(String... keys);
Long sinterstore(String dstkey, String... keys);
Long smove(String srckey, String dstkey, String member);
Long sort(String key, SortingParams sortingParameters, String dstkey);
Long sort(String key, String dstkey);
Set sunion(String... keys);
Long sunionstore(String dstkey, String... keys);
String watch(String... keys);
String unwatch();
Long zinterstore(String dstkey, String... sets);
Long zinterstore(String dstkey, ZParams params, String... sets);
Long zunionstore(String dstkey, String... sets);
Long zunionstore(String dstkey, ZParams params, String... sets);
String brpoplpush(String source, String destination, int timeout);
Long publish(String channel, String message);
void subscribe(JedisPubSub jedisPubSub, String... channels);
void psubscribe(JedisPubSub jedisPubSub, String... patterns);
String randomKey();
Long bitop(BitOP op, String destKey, String... srcKeys);
@Deprecated
/**
* @see #scan(String, ScanParams)
*/
ScanResult scan(int cursor);
ScanResult scan(String cursor);
/**
* Iterates the set of keys in the currently selected Redis database.
*
* Since this command allows for incremental iteration, returning only a small number of elements per call,
* it can be used in production without the downside of commands like {@link #keys(String)} or
* {@link JedisCommands#smembers(String)} )} that may block the server for a long time (even several seconds)
* when called against big collections of keys or elements.
*
* SCAN basic usage
* SCAN is a cursor based iterator. This means that at every call of the command, the server returns an updated cursor
* that the user needs to use as the cursor argument in the next call.
* An iteration starts when the cursor is set to 0, and terminates when the cursor returned by the server is 0.
*
* Scan guarantees
* The SCAN command, and the other commands in the SCAN family, are able to provide to the user a set of guarantees
* associated to full iterations.
*
* - A full iteration always retrieves all the elements that were present in the collection from the start to the
* end of a full iteration. This means that if a given element is inside the collection when an iteration is started,
* and is still there when an iteration terminates, then at some point SCAN returned it to the user.
*
- A full iteration never returns any element that was NOT present in the collection from the start to the end of
* a full iteration. So if an element was removed before the start of an iteration, and is never added back to the
* collection for all the time an iteration lasts, SCAN ensures that this element will never be returned.
*
* However because SCAN has very little state associated (just the cursor) it has the following drawbacks:
*
* - A given element may be returned multiple times. It is up to the application to handle the case of duplicated
* elements, for example only using the returned elements in order to perform operations that are safe when re-applied
* multiple times.
*
- Elements that were not constantly present in the collection during a full iteration, may be returned or not:
* it is undefined.
*
*
* Time complexity: O(1) for every call. O(N) for a complete iteration, including enough command calls for the cursor
* to return back to 0. N is the number of elements inside the DB.
*
* @param cursor The cursor.
* @param params the scan parameters. For example a glob-style match pattern
* @return the scan result with the results of this iteration and the new position of the cursor
* @see Redis SCAN documentation
*/
ScanResult scan(String cursor, ScanParams params);
String pfmerge(String destkey, String... sourcekeys);
Long pfcount(String... keys);
Long touch(String... keys);
}