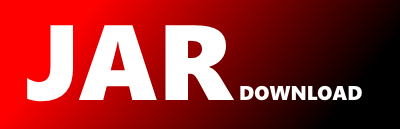
com.github.sdnwiselab.sdnwise.controller.ControllerFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdn-wise-ctrl Show documentation
Show all versions of sdn-wise-ctrl Show documentation
Control Plane solution for Software Defined Wireless Sensor Networks
The newest version!
/*
* Copyright (C) 2015 SDN-WISE
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package com.github.sdnwiselab.sdnwise.controller;
import com.github.sdnwiselab.sdnwise.adapter.AbstractAdapter;
import com.github.sdnwiselab.sdnwise.adapter.AdapterTcp;
import com.github.sdnwiselab.sdnwise.adapter.AdapterUdp;
import com.github.sdnwiselab.sdnwise.configuration.ConfigController;
import com.github.sdnwiselab.sdnwise.configuration.Configurator;
import com.github.sdnwiselab.sdnwise.topology.NetworkGraph;
import com.github.sdnwiselab.sdnwise.topology.VisualNetworkGraph;
import java.net.InetSocketAddress;
/**
* Builder of AbstractController objects given the specifications contained in a
* Configurator object. In the current version the only possible lower adapter
* is an AdapterUdp while the only possible algorithm is Dijkstra
*
* @author Sebastiano Milardo
*/
public class ControllerFactory {
/**
* Controller Identificator.
*/
private static InetSocketAddress id = null;
/**
* Returns the corresponding AbstractController object given a Configurator
* object.
*
* @param config a Configurator object.
* @return an AbstractController object.
*/
public final AbstractController getController(final Configurator config) {
AbstractAdapter adapt = getLower(config.getController());
NetworkGraph ng = getNetworkGraph(config.getController());
return getControllerType(config.getController(), id, adapt, ng);
}
/**
* Creates a controller. The only accepted type of controller at the moment
* is Dijkstra.
*
* @param conf a ConfigController class containing the config parameters of
* the controller
* @param newId the id of the controller
* @param adapt the lower adapter of the controller
* @param ng the NetworkGraph used
* @return an AbstractController
*/
public final AbstractController getControllerType(
final ConfigController conf,
final InetSocketAddress newId,
final AbstractAdapter adapt,
final NetworkGraph ng) {
String type = conf.getAlgorithm().get("TYPE");
switch (type) {
case "DIJKSTRA":
return new ControllerDijkstra(newId, adapt, ng);
default:
throw new UnsupportedOperationException(
"Error in Configuration file");
}
}
/**
* Creates a lower adapter for the controller.
*
* @param conf a ConfigController class containing the config parameters of
* the controller
* @return the lower adapter
*/
private AbstractAdapter getLower(final ConfigController conf) {
String type = conf.getLower().get("TYPE");
id = new InetSocketAddress(conf.getLower().get("IP"),
Integer.parseInt(conf.getLower().get("PORT")));
switch (type) {
case "TCP":
return new AdapterTcp(conf.getLower());
case "UDP":
return new AdapterUdp(conf.getLower());
default:
throw new UnsupportedOperationException("Error in config file");
}
}
/**
* Creates a NetworkGraph for the controller. This parameter manages the UI
* of the controller.
*
* @param conf a ConfigController class containing the config parameters of
* the controller
* @return the NetworkGraph object
*/
private NetworkGraph getNetworkGraph(final ConfigController conf) {
String graph = conf.getMap().get("GRAPH");
int timeout = Integer.parseInt(conf.getMap().get("TIMEOUT"));
int rssiResolution = Integer.parseInt(conf.getMap()
.get("RSSI_RESOLUTION"));
switch (graph) {
case "GUI":
return new VisualNetworkGraph(timeout, rssiResolution);
case "CLI":
return new NetworkGraph(timeout, rssiResolution);
default:
throw new UnsupportedOperationException(
"Error in Configuration file");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy