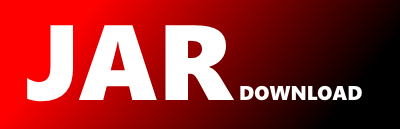
com.github.sdnwiselab.sdnwise.node.SensorNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdn-wise-node Show documentation
Show all versions of sdn-wise-node Show documentation
The stateful Software Defined Networking solution for WIreless SEnsor Networks
/*
* Copyright (C) 2015 SDN-WISE
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package com.github.sdnwiselab.sdnwise.node;
import com.github.sdnwiselab.sdnwise.flowtable.*;
import static com.github.sdnwiselab.sdnwise.flowtable.Window.*;
import static com.github.sdnwiselab.sdnwise.node.Constants.*;
import com.github.sdnwiselab.sdnwise.packet.*;
import static com.github.sdnwiselab.sdnwise.packet.NetworkPacket.*;
import com.github.sdnwiselab.sdnwise.util.NodeAddress;
import java.util.logging.*;
/**
*
* @author Sebastiano Milardo
*/
public class SensorNode extends Node {
private final Logger LOGGER;
public SensorNode(byte net_id, NodeAddress node,
int port,
String neighboursPath,
String logLevel) {
super(node, net_id, port, neighboursPath, logLevel);
cnt_sleep_max = SDN_WISE_DFLT_CNT_SLEEP_MAX;
LOGGER = Logger.getLogger(addr.toString());
}
@Override
public final void initSdnWise() {
super.initSdnWise();
setNum_hop_vs_sink(ttl_max + 1);
setRssi_vs_sink(0);
setSemaphore(0);
}
@Override
final void resetSemaphore() {
setSemaphore(0);
setNum_hop_vs_sink(255);
}
@Override
public void SDN_WISE_Callback(DataPacket packet) {
if (this.functions.get(1) == null) {
packet.setSrc(addr)
.setDst(getActualSinkAddress())
.setTtl((byte) ttl_max);
runFlowMatch(packet);
} else {
this.functions.get(1).function(adcRegister,
flowTable,
neighborTable,
statusRegister,
acceptedId,
flowTableQueue,
txQueue,
0,
0,
0,
packet);
}
}
@Override
public void rxBeacon(BeaconPacket bp, int rssi) {
if (rssi > rssi_min) {
if (bp.getDist() < this.getDistanceFromSink()
&& (rssi > getRssiSink())) {
NodeAddress oldSink = getActualSinkAddress();
if (!(oldSink.equals(bp.getSinkAddress()))) {
LOGGER.log(Level.FINE, "[{0}]: There is a better Sink", addr);
}
this.setSemaphore(1);
FlowTableEntry toSink = new FlowTableEntry();
toSink.addWindow(new Window()
.setOperator(SDN_WISE_EQUAL)
.setSize(SDN_WISE_SIZE_2)
.setLhsLocation(SDN_WISE_PACKET)
.setLhs(SDN_WISE_DST_H)
.setRhsLocation(SDN_WISE_CONST)
.setRhs(bp.getSinkAddress().intValue()));
toSink.addWindow(Window.fromString("P.TYPE > 127"));
toSink.addAction(new ForwardUnicastAction()
.setNextHop(bp.getSrc()));
flowTable.set(0, toSink);
setNum_hop_vs_sink(bp.getDist() + 1);
setRssi_vs_sink(rssi);
} else if ((bp.getDist() + 1) == this.getDistanceFromSink()
&& getNextHopVsSink().equals(bp.getSrc())) {
flowTable.get(0).getStats().restoreTtl();
flowTable.get(0).getWindows().get(0)
.setRhs(bp.getSinkAddress().intValue());
}
super.rxBeacon(bp, rssi);
}
}
@Override
public final void controllerTX(NetworkPacket pck) {
pck.setNxhop(getNextHopVsSink());
radioTX(pck, SDN_WISE_MAC_SEND_UNICAST);
}
@Override
public void rxConfig(ConfigPacket packet) {
NodeAddress dest = packet.getDst();
if (!dest.equals(addr)) {
runFlowMatch(packet);
} else {
if (this.marshalPacket(packet) != 0) {
packet.setSrc(addr);
packet.setDst(getActualSinkAddress());
packet.setTtl((byte) ttl_max);
runFlowMatch(packet);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy