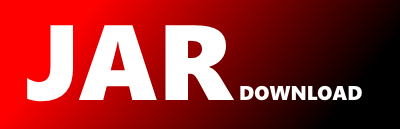
inet.ipaddr.format.util.AssociativeAddedTree Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ipaddress Show documentation
Show all versions of ipaddress Show documentation
Library for handling IP addresses, both IPv4 and IPv6
/*
* Copyright 2022 Sean C Foley
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
* or at
* https://github.com/seancfoley/IPAddress/blob/master/LICENSE
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package inet.ipaddr.format.util;
import java.util.List;
import inet.ipaddr.Address;
import inet.ipaddr.format.util.AssociativeAddressTrie.AssociativeTrieNode;
import inet.ipaddr.format.util.AssociativeAddressTrie.SubNodesMappingAssociative;
/**
* AssociativeAddedTree is similar to AddedTree but originates from an AssociativeTrie.
* The nodes of this tree have the same values as the corresponding nodes in the original trie.
*/
public class AssociativeAddedTree extends AddedTreeBase> {
public AssociativeAddedTree(AssociativeAddressTrie> wrapped) {
super(wrapped);
}
/**
* AssociativeAddedTreeNode represents a node in an AssociativeAddedTree.
*/
public static class AssociativeAddedTreeNode extends AddedTreeNodeBase> {
public AssociativeAddedTreeNode(AssociativeTrieNode> node) {
super(node);
}
@Override
public AssociativeAddedTreeNode[] getSubNodes() {
SubNodesMappingAssociative value = node.getValue();
if(value == null) {
return null;
}
List>> subNodes = value.subNodes;
if(subNodes == null || subNodes.size() == 0) {
return null;
}
@SuppressWarnings("unchecked")
AssociativeAddedTreeNode[] nodes = (AssociativeAddedTreeNode[]) new AssociativeAddedTreeNode[subNodes.size()];
for(int i = 0; i < nodes.length; i++) {
nodes[i] = new AssociativeAddedTreeNode(subNodes.get(i));
}
return nodes;
}
}
/**
* Returns the root of this tree, which corresponds to the root of the originating trie.
*/
@Override
public AssociativeAddedTreeNode getRoot() {
return new AssociativeAddedTreeNode(wrapped.getRoot());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy