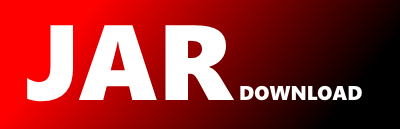
seleniumConsulting.ch.selenium.framework.allure.AllureUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of selenium-java-toolkit-base Show documentation
Show all versions of selenium-java-toolkit-base Show documentation
Selenium-Toolkit is a Java based test-toolkit for selenium-testing with testNg.
The goal of the toolkit is, to suport you in different things like 'how to manage testdata in the source', parallelisation, Webdriver-managemet, reporting and a lot more.
package seleniumConsulting.ch.selenium.framework.allure;
import java.util.*;
import io.qameta.allure.Allure;
import io.qameta.allure.model.Parameter;
import io.qameta.allure.model.Status;
import io.qameta.allure.model.StepResult;
/**
* AllureUtils can be used to start and stop TestSteps and to log informations
*/
public class AllureUtils {
/**
* Map to store the current Step UUID.
* The User haven't to store it after startStep for the stopStep, this map will do it for him.
*/
private static Map uuidThreadIdMap = new HashMap();
/**
* Start a Teststep for the AllureReport with one Parameter
* @param name of the Step
* @param value: Parameters, which should be attached to the Step in allure
*/
public static void startStep(String name, Parameter value){
startStep(name, Arrays.asList(value));
}
/**
* Start a Teststep for the AllureReport with a List of Parameters
* @param name of the Step
* @param values: List of Parameters, which should be attached to the Step in allure
*/
public static void startStep(String name, List values){
Allure.getLifecycle().startStep(createUuid(), new StepResult().setName(name).setParameters(values));
}
/**
* Start a Teststep for the AllureReport without Parameters
* @param name of the Step
*/
public static void startStep(String name){
Allure.getLifecycle().startStep(createUuid(), new StepResult().setName(name));
}
/**
* Stop the current Teststep with the Status 'Passed'
*/
public static void stopStepPassed(){
stopStepWithStatus(Status.PASSED);
}
/**
* Stop the current Teststep with Status
* @param status in which the Teststep should be reportet
*/
public static void stopStepWithStatus(Status status){
Allure.getLifecycle().updateStep(getUuid(), (s) -> s.setStatus(status));
Allure.getLifecycle().stopStep(getUuid());
delUuid();
}
/**
* Log a message with Status 'Passed'
* It starts a new Step with Name = message and stop the Step with the Status
* @param message to report
*/
public static void logSuccess(String message){
logInStatus(message, Status.PASSED);
}
/**
* Please use logSuccess or log Skipped
* There is no Status 'Info' so this will Log the message with Status 'Passed'
* @param message to report
*/
@Deprecated()
public static void logInfo(String message){
logInStatus(message, Status.PASSED);
}
/**
* Log a message with Status 'Skipped'
* It starts a new Step with Name = message and stop the Step with the Status
* @param message to report
*/
public static void logSkipped(String message){
logInStatus(message, Status.SKIPPED);
}
/**
* Log a message with Status 'Error'
* It starts a new Step with Name = message and stop the Step with the Status
* @param message to report
*/
public static void logError(String message){
logInStatus(message, Status.FAILED);
}
/**
* Starts a new Step with Name = message and stop the Step with the Status
* @param message is the Name of the Step
* @param status to close Step in this Status
*/
private static void logInStatus(String message, Status status){
startStep(message);
stopStepWithStatus(status);
}
/**
* Getting the UUID for this Thread out of the uuidThreadIdMap
* @return UUID of current Thread
*/
private static String getUuid() {
return (String) uuidThreadIdMap.get((int) (Thread.currentThread().getId()));
}
/**
* Create a new UUID for this Thread and store it into the uuidThreadIdMap
* @return UUID of current Thread
*/
private static String createUuid(){
String uuid = UUID.randomUUID().toString();
uuidThreadIdMap.put((int) (Thread.currentThread().getId()), uuid);
return uuid;
}
/**
* Delete the UUID of this Thread in the uuidThreadIdMap
*/
private static void delUuid(){
uuidThreadIdMap.remove((int) (Thread.currentThread().getId()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy