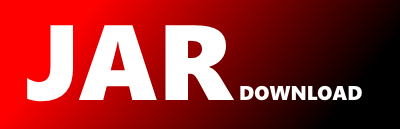
seleniumConsulting.ch.selenium.framework.database.DatabaseScriptExecutor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of selenium-java-toolkit-base Show documentation
Show all versions of selenium-java-toolkit-base Show documentation
Selenium-Toolkit is a Java based test-toolkit for selenium-testing with testNg.
The goal of the toolkit is, to suport you in different things like 'how to manage testdata in the source', parallelisation, Webdriver-managemet, reporting and a lot more.
package seleniumConsulting.ch.selenium.framework.database;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.Reader;
import java.io.StringReader;
import java.net.URL;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.sql.Connection;
import java.sql.DriverManager;
import java.util.Arrays;
import java.util.Map;
import org.apache.ibatis.jdbc.ScriptRunner;
import io.qameta.allure.model.Parameter;
import io.qameta.allure.model.Status;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import seleniumConsulting.ch.selenium.framework.allure.AllureUtils;
/**
* This Class executes a a Database SQL Script. In most cases it si used to setup data for Selenium Tests
*
* Created by C910309 on 11.02.2019.
*/
public class DatabaseScriptExecutor {
private static final Logger LOGGER = LoggerFactory.getLogger(DatabaseScriptExecutor.class);
public static void executeSqlScript(String sqlScriptFilePath) {
try {
executeSqlScript(getReader(sqlScriptFilePath),
getConnection(sqlScriptFilePath));
AllureUtils.stopStepPassed();
} catch (Exception e){
LOGGER.error("Error during executeSqlScript: " + e.getMessage());
AllureUtils.stopStepWithStatus(Status.FAILED);
}
}
public static void executeSqlScriptParametrized(String sqlScriptFilePath, Map parameters) throws Exception {
executeSqlScript(getReaderParametrized(sqlScriptFilePath, parameters),
getConnection(sqlScriptFilePath));
}
private static Connection getConnection(String sqlScriptFilePath) throws Exception {
String dbUser = System.getProperty("javax.persistence.jdbc.user");
String dbPw = System.getProperty("javax.persistence.jdbc.password");
String url = System.getProperty("javax.persistence.jdbc.url");
String dbScriptDirectory = System.getProperty("persistence.script.dir");
sqlScriptFilePath = dbScriptDirectory + sqlScriptFilePath;
AllureUtils.startStep("Execute Script", Arrays.asList(
new Parameter().setName("Sql-File").setValue(sqlScriptFilePath),
new Parameter().setName("Url").setValue(url),
new Parameter().setName("dbUser").setValue(dbUser))
);
return DriverManager.getConnection(url, dbUser, dbPw);
}
private static Reader getReader(String sqlScriptFilePath) throws Exception{
ClassLoader loader = Thread.currentThread().getContextClassLoader();
URL file = loader.getResource(sqlScriptFilePath);
// Give the input file to Reader
return new BufferedReader(new FileReader(file.getFile()));
}
private static Reader getReaderParametrized(String sqlScriptFilePath, Map parameters) throws Exception{
ClassLoader loader = Thread.currentThread().getContextClassLoader();
URL file = loader.getResource(sqlScriptFilePath);
String fileContent = new String(Files.readAllBytes(Paths.get(file.toURI())));
for(Map.Entry entry: parameters.entrySet() ){
fileContent = fileContent.replaceAll(entry.getKey(), entry.getValue());
}
return new StringReader(fileContent);
}
private static void executeSqlScript(Reader reader, Connection con) throws Exception {
// Initialize object for ScripRunner
ScriptRunner sr = new ScriptRunner(con);
// Exctute script
sr.runScript(reader);
con.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy