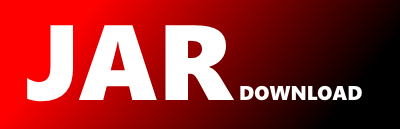
seleniumConsulting.ch.selenium.framework.dataLoader.TestDataProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of selenium-java-toolkit-base Show documentation
Show all versions of selenium-java-toolkit-base Show documentation
Selenium-Toolkit is a Java based test-toolkit for selenium-testing with testNg.
The goal of the toolkit is, to suport you in different things like 'how to manage testdata in the source', parallelisation, Webdriver-managemet, reporting and a lot more.
package seleniumConsulting.ch.selenium.framework.dataLoader;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.util.Map;
import java.util.Properties;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import seleniumConsulting.ch.selenium.framework.allure.AllureTextEnum;
import seleniumConsulting.ch.selenium.framework.database.DatabaseScriptExecutor;
/**
* TestDataProvider to get the Datas from testconfig/data.properties and testdata/data.properties
*/
public class TestDataProvider {
/**
* Logger for Class
*/
private static final Logger LOGGER = LoggerFactory.getLogger(TestDataProvider.class);
/**
* Destination/Name of testdata-Folder
*/
private static final String FOLDER_TESTDATA_PROPERTIES = "testdata/";
/**
* Destination/Name of testconfig-Folder
*/
private static final String FOLDER_TESTCONFIG_PROPERTIES = "testconfig/";
/**
* Name of testdata-File
*/
private static final String FILE_TESTDATA_PROPERTIES = "data.properties";
/**
* Name of testconfig-File
*/
private static final String FILE_TESTCONFIG_PROPERTIES = "data.properties";
/**
* Name of AllureTexte File
*/
private static final String FILE_ALLURETEXT_PROPERTIES = "allureText.properties";
/**
* Property-Name to prefix the File-Names to load specific additional Property-File
*/
public static final String USER_NAME = "user.name";
/**
* All loaded Properties
*/
private Properties testDataProperties;
/**
* Instance of the TestDataProvider for Singelton-Pattern
*/
private static TestDataProvider instance = null;
/**
* Getting the {@link TestDataProvider}-Instance (Singelton-Pattern)
* @return TestDataProvider
*/
public static TestDataProvider getInstance() {
if (instance == null) {
instance = new TestDataProvider();
}
return instance;
}
/**
* Create the {@link TestDataProvider} and loads:
* - The testdata-Files
* - The testconfig-Files
* - The Allure texte-Files
*/
private TestDataProvider() {
testDataProperties = loadConfigAndSetAsSystemProperties(FOLDER_TESTCONFIG_PROPERTIES, FILE_TESTCONFIG_PROPERTIES, false);
testDataProperties.putAll(loadConfigAndSetAsSystemProperties(FOLDER_TESTDATA_PROPERTIES, FILE_TESTDATA_PROPERTIES, false));
testDataProperties.putAll(loadConfigAndSetAsSystemProperties("", FILE_ALLURETEXT_PROPERTIES, true));
testDataProperties.putAll(loadConfigAndSetAsSystemProperties(FOLDER_TESTCONFIG_PROPERTIES, FILE_ALLURETEXT_PROPERTIES, false));
}
/**
* Getting the Value of the Property to the key
* @param key to Property
* @return Value of Property
*/
public static String getTestData(String key) {
try {
return getInstance().getTestDataProperties().get(key).toString();
} catch (NullPointerException e) {
LOGGER.info("TestDataProvider.getTestData: NullPointer, key:("+key+") and returns \"\"");
return "";
}
}
/**
* Getting the Allure Text to an {@link AllureTextEnum}
* @param key as {@link AllureTextEnum} to Load Text
* @return Text for Allure Reporting
*/
public static String getTestData(AllureTextEnum key) {
try {
return getInstance().getTestDataProperties().get(key.getValue()).toString();
} catch (NullPointerException e) {
return "";
}
}
/**
* Load Properties from folder/propertieFileName and Prefix-Property-File from Toolkit or Testproject and set it
* as System-Properties
* @param folder to the Property-File
* @param propertieFileName name of Property-File
* @param fromToolkit should the Properties be loaded from Toolkit-Project or Testproject
* @return Properties
*/
private static Properties loadConfigAndSetAsSystemProperties(String folder, String propertieFileName, boolean fromToolkit) {
Properties props = loadConfig(folder, propertieFileName, fromToolkit);
for (Object o : props.entrySet()) {
Map.Entry entry = (Map.Entry) o;
System.setProperty((String) entry.getKey(), (String) entry.getValue());
}
return props;
}
/**
* Load Properties from folder/propertieFileName and Prefix-Property-File from Toolkit or Testproject
* @param folder to the Property-File
* @param propertieFileName name of Property-File
* @param fromToolkit should the Properties be loaded from Toolkit-Project or Testproject
* @return Properties
*/
private static Properties loadConfig(String folder, String propertieFileName, boolean fromToolkit) {
String defaultProps = folder + propertieFileName;
Properties properties = loadProperties(defaultProps, fromToolkit);
String filename = folder + System.getProperties().getProperty(USER_NAME) + "." + propertieFileName;
Properties userProperties = loadProperties(filename, fromToolkit);
if (userProperties != null) {
properties.putAll(userProperties);
}
return properties;
}
/**
* Load Properties from File out of the Toolkit or Testproject
* @param name File folder and name
* @param fromToolkit should the Properties be loaded from Toolkit-Project or Testproject
* @return
*/
private static Properties loadProperties(String name, boolean fromToolkit) {
ClassLoader loader;
if(fromToolkit) {
loader = TestDataProvider.class.getClassLoader();
} else {
loader = Thread.currentThread().getContextClassLoader();
}
URL url = loader.getResource(name);
Properties props = new Properties();
if (url != null) {
try {
InputStream e = url.openStream();
props.load(e);
} catch (IOException e) {
LOGGER.error("TestDataProvider.loadProperties faild. Exception-Message:" + e.getMessage());
}
}
return props;
}
/**
* Getting the loaded Properties
* @return Properties
*/
private Properties getTestDataProperties() {
return testDataProperties;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy