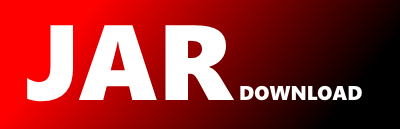
vendors.grid.Element34VendorSBox Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of selenium-java-toolkit-base Show documentation
Show all versions of selenium-java-toolkit-base Show documentation
Selenium-Toolkit is a Java based test-toolkit for selenium-testing with testNg.
The goal of the toolkit is, to suport you in different things like 'how to manage testdata in the source', parallelisation, Webdriver-managemet, reporting and a lot more.
package vendors.grid;
import java.io.File;
import java.io.IOException;
import java.net.URL;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.Base64;
import java.util.HashMap;
import java.util.Map;
import com.google.common.base.Strings;
import org.openqa.selenium.MutableCapabilities;
import org.openqa.selenium.remote.SessionId;
import com.google.common.base.Splitter;
import com.google.common.collect.ImmutableMap;
import io.qameta.allure.Allure;
import io.qameta.allure.model.Link;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import seleniumConsulting.ch.selenium.framework.allure.AllureTextEnum;
import seleniumConsulting.ch.selenium.framework.allure.AllureUtils;
import seleniumConsulting.ch.selenium.framework.dataLoader.TestDataProvider;
import seleniumConsulting.ch.selenium.framework.database.DatabaseScriptExecutor;
import seleniumConsulting.ch.selenium.framework.driver.WebDriverFactory;
import seleniumConsulting.ch.selenium.framework.metadata.MetadataKey;
public class Element34VendorSBox implements VendorInterface {
private static final Logger LOGGER = LoggerFactory.getLogger(Element34VendorSBox.class);
public static final String P_12 = "pki.p12";
public static final String PW = "pki.pw";
@Override
public void addCapibilities(TestInfo info, MutableCapabilities mutableCapabilities) {
mutableCapabilities.setCapability("e34:l_testName", info.getTestname());
if(! Strings.isNullOrEmpty(TestDataProvider.getTestData(P_12))) {
File p12 = new File(TestDataProvider.getTestData(P_12));
try {
byte[] bytes = Files.readAllBytes(Paths.get(p12.getAbsolutePath()));
String myP12InBase64 = Base64.getEncoder().encodeToString(bytes);
Map pkiAuth = new HashMap<>();
pkiAuth.put("p12", myP12InBase64);
pkiAuth.put("password", TestDataProvider.getTestData(PW));
mutableCapabilities.setCapability("e34:pki_auth", pkiAuth);
} catch (IOException e) {
e.printStackTrace();
throw new RuntimeException("Fail PKI init", e);
}
}
}
@Override
public void onTestSuccess(TestInfo info) {
}
@Override
public void onTestFailure(TestInfo info) {
}
@Override
public void onTestStart(TestInfo info) {
}
@Override
public void onTestSkipped(TestInfo info) {
}
@Override
public void createVideo(TestInfo info, SessionId sessionId) {
createVideoLink(sessionId);
}
/**
* Download the Video of a {@link org.openqa.selenium.remote.RemoteWebDriver}, if its not working, it will create a Link to the video in the Report
* It will test is there a {@link org.openqa.selenium.remote.RemoteWebDriver} and is e34:video on in this Test
* @param id of {@link org.openqa.selenium.remote.RemoteWebDriver} to download Video / create Link
*/
private static void createVideoLink(SessionId id) {
boolean isVideoActive = false;
if (!Strings.isNullOrEmpty(System.getProperty(MetadataKey.CAPABILITIES))) {
Map map = ImmutableMap.copyOf(Splitter.on(',')
.withKeyValueSeparator("=")
.split(System.getProperty(MetadataKey.CAPABILITIES)));
if (map.get("e34:video") != null && map.get("e34:video").equals("true")) {
isVideoActive = true;
}
}
if (id != null && isVideoActive) {
saveVideo(id);
}
}
/**
* Report Allure Step 'Video Download' and adds the Video as Attachment.
* If its not working will add Link to the Video to Report
* @param id to the {@link org.openqa.selenium.remote.RemoteWebDriver}
*/
private static void saveVideo(SessionId id) {
try {
AllureUtils.startStep(TestDataProvider.getTestData(AllureTextEnum.VideoDownload));
Thread.sleep(200);
Allure.addAttachment("Video", "video/mp4", new URL(System.getProperty(WebDriverFactory.REMOTE_GRID_URL)+"/videos/"+ id + ".mp4").openStream(), "mp4");
AllureUtils.stopStepPassed();
} catch (Exception e) {
LOGGER.error("Downloading Video Faild, create Link");
Allure.addLinks(new Link()
.setName(TestDataProvider.getTestData(AllureTextEnum.VideoLinkName))
.setUrl(System.getProperty(WebDriverFactory.REMOTE_GRID_URL)+"/videos/"+ id + ".mp4"));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy