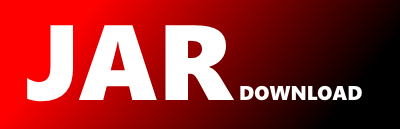
com.semoncat.geach.core.GameManager Maven / Gradle / Ivy
package com.semoncat.geach.core;
import android.content.ComponentName;
import android.content.Context;
import android.content.Intent;
import android.content.pm.PackageInfo;
import android.content.pm.PackageManager;
import android.content.pm.ResolveInfo;
import android.graphics.drawable.Drawable;
import com.semoncat.geach.core.bean.Game;
import dalvik.system.PathClassLoader;
import java.lang.reflect.InvocationTargetException;
import java.util.ArrayList;
import java.util.List;
/**
* 負責載入遊戲
* Created by SemonCat on 2014/7/3.
*/
public class GameManager {
private static final String TAG = GameManager.class.getName();
private Context context;
public GameManager(Context context) {
this.context = context;
}
/**
* 列出 deivce 中所有 Geach 的遊戲。
* @return List
*/
public List getAllInstallGame() {
List games = new ArrayList();
Intent queryIntent = new Intent(GeachGame.ACTION_Geach_Game);
PackageManager pm = context.getPackageManager();
List resolveInfos = pm.queryIntentServices(queryIntent,
PackageManager.GET_META_DATA);
for (ResolveInfo ri : resolveInfos) {
try {
ComponentName componentName = new ComponentName(ri.serviceInfo.packageName, ri.serviceInfo.name);
PackageInfo packageInfo = pm.getPackageInfo(componentName.getPackageName(), 0);
String name = ri.loadLabel(pm).toString();
Drawable icon = ri.loadIcon(pm);
String pkgName = packageInfo.packageName;
String scrDir = packageInfo.applicationInfo.sourceDir;
int versionCode = packageInfo.versionCode;
String versionName = packageInfo.versionName;
games.add(new Game(name, icon, pkgName,scrDir, versionCode, versionName, componentName));
} catch (PackageManager.NameNotFoundException e) {
}
}
return games;
}
/**
* 取得遊戲的 Fragment
* @param targetGame
* @return GameFragment
* @throws ClassNotFoundException
* @throws NoSuchMethodException
* @throws IllegalAccessException
* @throws InvocationTargetException
* @throws InstantiationException
* @throws PackageManager.NameNotFoundException
*/
public BaseGameFragment getGameFragment(Game targetGame) throws ClassNotFoundException, NoSuchMethodException, IllegalAccessException, InvocationTargetException, InstantiationException, PackageManager.NameNotFoundException {
PathClassLoader classLoader = new PathClassLoader(
targetGame.sourceDir,
context.getClassLoader());
Class service = classLoader.loadClass(targetGame.componentName.getClassName());
GeachGame geachGame = (GeachGame)service.getConstructor().newInstance();
Context moduleContext = context.createPackageContext(
targetGame.packageName,
Context.CONTEXT_INCLUDE_CODE | Context.CONTEXT_IGNORE_SECURITY);
return geachGame.getGameFragment(moduleContext);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy