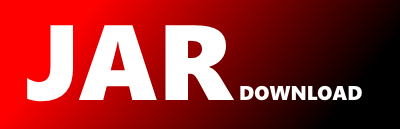
com.semoncat.geach.core.BaseGameFragment Maven / Gradle / Ivy
package com.semoncat.geach.core;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.Fragment;
import android.content.Context;
import android.content.SharedPreferences;
import android.content.pm.PackageManager;
import android.os.Bundle;
import android.preference.PreferenceManager;
import android.util.Log;
import android.view.ContextThemeWrapper;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.MenuInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Toast;
import com.semoncat.geach.core.bean.Game;
import com.semoncat.geach.core.bean.ScoreResult;
import com.semoncat.geach.core.bean.Topic;
import com.semoncat.geach.core.bean.VoteResult;
/**
* Created by SemonCat on 2014/7/3.
*/
@SuppressLint("ValidFragment")
public abstract class BaseGameFragment extends Fragment{
public interface OnGameEventListener{
public void OnGameOver(ScoreResult gameScore);
public void OnVoteOver(VoteResult gameScore);
}
private static final String TAG = BaseGameFragment.class.getName();
private OnGameEventListener mListener;
private Topic mGameResource;
public static final String GAME = "game";
private Game game;
private void setupGame(){
game = getArguments().getParcelable(GAME);
if (game==null){
throw new NullPointerException("You need pass Game Object by use setArguments() method.");
}
}
private void setupData(){
mGameResource = new Topic();
testDataInject(mGameResource);
InjectGeachGameResource(mGameResource);
}
@Override
public void onAttach(Activity activity) {
super.onAttach(activity);
setupGame();
if(activity instanceof ProxyActivity){
try {
Context mContext = activity.getApplicationContext().createPackageContext(
game.packageName,
Context.CONTEXT_INCLUDE_CODE | Context.CONTEXT_IGNORE_SECURITY);
((ProxyActivity)activity).setPackageContext(mContext);
} catch (PackageManager.NameNotFoundException e) {
throw new IllegalArgumentException("PackageManager.NameNotFoundException.");
}
}else{
throw new IllegalArgumentException("You must use ProxyActivity.");
}
setupData();
}
public Game getGame() {
return game;
}
public Topic getGeachGameResource(){
if (mGameResource==null)
throw new NullPointerException("Please wait for Resource Inject.");
return mGameResource;
}
public void InjectGeachGameResource(Topic bundle){
this.mGameResource = bundle;
}
public abstract void OnGameStart();
protected void OnGameOver(ScoreResult gameScore){
if (mListener!=null){
mListener.OnGameOver(gameScore);
}
}
protected void OnVoteOver(VoteResult voteResult){
if (mListener!=null){
mListener.OnVoteOver(voteResult);
}
}
protected void testDataInject(Topic GameResource){
}
protected abstract void setupView(View rootView);
protected abstract void setupAdapter();
protected abstract void setupEvent();
protected abstract int setupLayout();
protected int setupTheme(){
return android.R.style.Theme_Holo_Light;
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
if (setupLayout()==0){
throw new IllegalArgumentException("You must overwrite setupLayout() method.");
}
ContextThemeWrapper contextThemeWrapper = new ContextThemeWrapper(getActivity(),setupTheme());
LayoutInflater context_inflater=(LayoutInflater)contextThemeWrapper.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View rootView = context_inflater.inflate(setupLayout(), container, false);
setupView(rootView);
setupAdapter();
setupEvent();
return rootView;
}
protected void finish(){
if (getFragmentManager()!=null){
getFragmentManager().beginTransaction().remove(this).commit();
}
}
protected SharedPreferences getSharedPreferences(){
return PreferenceManager.getDefaultSharedPreferences(getActivity());
}
Toast mToast;
protected void showToast(String Message){
if (mToast==null){
mToast = Toast.makeText(getActivity(),Message,Toast.LENGTH_SHORT);
}else{
mToast.setText(Message);
}
mToast.show();
}
public void setGameEventListener(OnGameEventListener mListener) {
this.mListener = mListener;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy