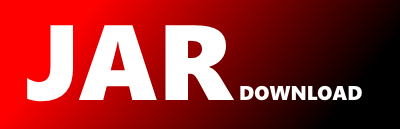
com.github.sevntu.checkstyle.checks.coding.checkstyle-metadata.properties Maven / Gradle / Ivy
AvoidConstantAsFirstOperandInConditionCheck.name = Avoid Constant As First Operand In Condition
AvoidConstantAsFirstOperandInConditionCheck.desc = If comparing values, C(C++) developers prefer to put the constant first in the equality check, to prevent situations of assignment rather than equality checking.
But in Java, in IF condition it is impossible to use assignment, so that habit become unnecessary and do damage readability of code.
In C(C++), comparison for null is tricky, and it is easy to write "=" instead of "==", and no complication error will be but condition will work in different way
Example:
if (null == variable)
rather than
if (variable == null)
because if you forget one (typo mistake) of the equal sign, you end up with
if (variable = null)
which assigns null to variable and IF always evaluate to true.
AvoidConstantAsFirstOperandInConditionCheck.targetConstantTypes = Target Constant Types
AvoidDefaultSerializableInInnerClassesCheck.name = Avoid default implementation of serializable interface
AvoidDefaultSerializableInInnerClassesCheck.desc = This check prevents the default implementation Serializable interface in inner classes (Serializable interface are default if methods readObject() or writeObject() are not override in class). Check has option, that allow implementation only one method, if it true, but if it false - class must implement both methods. For more information read "Effective Java (2nd edition)" chapter 11, item 74, page 294.
AvoidDefaultSerializableInInnerClassesCheck.allowPartialImplementation = Allow partial implementation for serializable interface
AvoidHidingCauseExceptionCheck.name = Avoid Hiding Cause of the Exception
AvoidHidingCauseExceptionCheck.desc = Warns when you try to hide cause of an exception when rethrowing
AvoidNotShortCircuitOperatorsForBooleanCheck.name = Avoid using bitwise operations for boolean expressions
AvoidNotShortCircuitOperatorsForBooleanCheck.desc = Avoid using bitwise operations for boolean expressions
AvoidModifiersForTypesCheck.name = Avoid Modifiers For Types
AvoidModifiersForTypesCheck.desc = Disallow some set of modifiers for Java types specified by regexp.
Only 4 types according to Java Spec: static, final, transient, volatile.
Example:
"static" modifier for ULCComponents (http://ulc-community.canoo.com/snipsnap/space/Good+Practices) is not allowed.
So we can disallow "static" modifier for all ULC* components by setting up "forbiddenClassesRegexpStatic" option to "ULC.+" regexp String.
AvoidModifiersForTypesCheck.forbiddenClassesRegexpAnnotation = The regexp defines the names of classes, that could not have 'Annotation' modifier.
AvoidModifiersForTypesCheck.forbiddenClassesRegexpFinal = The regexp defines the names of classes, that could not have 'final' modifier.
AvoidModifiersForTypesCheck.forbiddenClassesRegexpStatic = The regexp defines the names of classes, that could not have 'static' modifier.
AvoidModifiersForTypesCheck.forbiddenClassesRegexpTransient = The regexp defines the names of classes, that could not have 'transient' modifier.
AvoidModifiersForTypesCheck.forbiddenClassesRegexpVolatile = The regexp defines the names of classes, that could not have 'volatile' modifier.
AvoidModifiersForTypesCheck.forbiddenClassesRegexpPrivate = The regexp defines the names of classes, that could not have 'private' modifier.
AvoidModifiersForTypesCheck.forbiddenClassesRegexpPackagePrivate = The regexp defines the names of classes, that could not have 'package-private' modifier.
AvoidModifiersForTypesCheck.forbiddenClassesRegexpProtected = The regexp defines the names of classes, that could not have 'protected' modifier.
AvoidModifiersForTypesCheck.forbiddenClassesRegexpPublic = The regexp defines the names of classes, that could not have 'public' modifier.
CustomDeclarationOrderCheck.name = Custom Declaration Order
CustomDeclarationOrderCheck.desc = Checks that the parts of a class(main, nested, member inner) declaration appear in the rules order set by user using regular expressions.
The check forms line which consists of class member annotations, modifiers,type and name from your code and compares it with your RegExp.
The rule consists of:*ClassMember(RegExp)
To set class order use the following notation of the class members (case insensitive):- "Field" to denote the Fields
- "DeclareAnnonClassField" to denote the fields keeping objects of anonymous classes
- "Ctor" to denote the Constructors
- "Method" to denote the Methods
- "GetterSetter" to denote the group of getter and setter methods
- "MainMethod" to denote the main method
- "InnerClass" to denote the Inner Classes
- "InnerInterface" to denote the Inner Interfaces
- "InnerEnum" to denote the Inner Enums
RegExp can include:- Annotations
- Modifiers(public, protected, private, abstract, static,final)
- Type
- Name
ATTENTION!Use separator ' ', '.', '\s'
between declaration in the RegExp. Whitespace should be added after each modifier.
Example: Field(public .*final .*) Field(public final .*) Field(public\s*
final .*)
NOTICE!It is important to write exact order of modifiers in rules. So ruleField(public final)
does not match to final public value;
.ModifierOrderCheckis recommended to use.
If you set empty RegExp e.g. Field()
, it means that class member doesn't have modifiers(default modifier) and checking the type and name of member doesn't occur.
Between the declaration of a array and generic can't be whitespaces.E.g.: ArrayList<String[]> someName
Use the separator '###' between the class declarations.
For Example:
Field(private static final long serialVersionUID) ###Field(public static final .*) ### Field(.*private .*) ### Ctor(.*) ###GetterSetter(.*) ### Method(.*public .*final .*|@Ignore.*public .*) ###Method(public static .*(final|(new|edit|create).*).*) ###InnerClass(public abstract .*) ### InnerInterface(.*) ### InnerEnum(.*)
*What is group of getters and setters(GetterSetter
)?
It is ordered sequence of getters and setters like:
public int getValue() { log.info("Getting value"); return value;} *public void setValue(int newValue) { value = newValue;} *public Object getObj() { return obj;} *public void setObj(Object obj) { if (obj != null) { this.obj = obj; } else { throw new IllegalArgumentException("Null value"); }} *...
Getter is public method that returns class field. Name of getter should be'getFieldName' in camel case.
Setter is public method with one parameter that assigns this parameter to class field. Name of setter should be 'setFieldName' in camel case.
Setter of field X should be right after getter of field X.
*@author Danil Lopatin@author Baratali Izmailov
CustomDeclarationOrderCheck.fieldPrefix = Prefix of field's name.
CustomDeclarationOrderCheck.customDeclarationOrder = Regular expression, which sets the order of the parts of a class(main, nested, member inner).
CustomDeclarationOrderCheck.caseSensitive = Set false to ignore case.
ConfusingConditionCheck.name = Confusing Condition
ConfusingConditionCheck.desc = This check prevents negation within an "if" expression if "else" is present.
For example, rephrase:
if (x != y) smth1();
else smth2();
as:
if (x == y) smth2();
else smth1();
ConfusingConditionCheck.multiplyFactorForElseBlocks = Disable warnings if "else" block length is in "multiplyFactorForElseBlocks" time less then "if" block.
ConfusingConditionCheck.ignoreInnerIf = Disable warnings for all inner "if" . It is useful for save similarity.
ConfusingConditionCheck.ignoreSequentialIf = Disable warnings for all sequential "if" . It is useful for save similarity.
ConfusingConditionCheck.ignoreNullCaseInIf = Disable warnings for "if" if it expression contains "null".
ConfusingConditionCheck.ignoreThrowInElse = Disable warnings for "if" if "else" block contain "throw".
EmptyPublicCtorInClassCheck.name = Empty Public Ctor In Class
EmptyPublicCtorInClassCheck.desc = This Check looks for useless empty public constructors. Class constructor is considered useless by this Check if and only if class has exactly one ctor, which is public, empty(one that has no statements) and default.
Example 1. Check will generate violation for this code:
class Dummy {
public Dummy() {
}
}
Example 2. Check will not generate violation for this code:
class Dummy {
private Dummy() {
}
}
class Dummy has only one ctor, which is not public.Example 3. Check will not generate violation for this code:
class Dummy {
public Dummy() {
}
public Dummy(int i) {
}
}
class Dummy has multiple ctors.Check has two properties:
"classAnnotationNames" - This property contains regex for canonical names of class annotations,which require class to have empty public ctor. Check will not log violations for classes marked with annotations that match this regex. Default option value is "javax\\.persistence\\.Entity".
"ctorAnnotationNames" - This property contains regex for canonical names of ctor annotations,which make empty public ctor essential. Check will not log violations for ctors marked with annotations that match this regex. Default option value is "com\\.google\\.inject\\.Inject".
@author Zuy Alexey
EmptyPublicCtorInClassCheck.classAnnotationNames = Regex which matches names of class annotations which require class to have public no-argument ctor. Default value is "javax\\.persistence\\.Entity".
EmptyPublicCtorInClassCheck.ctorAnnotationNames = Regex which matches names of ctor annotations which make empty public ctor essential. Default value is "com\\.google\\.inject\\.Inject".
FinalizeImplementationCheck.name = Finalize Implementation
FinalizeImplementationCheck.desc = This Check detects 3 most common cases of incorrect finalize() method implementation:
- negates effect of superclass finalize
protected void finalize() { }
protected void finalize() { doSomething(); }
- useless (or worse) finalize
protected void finalize() { super.finalize(); }
- public finalize
public void finalize() { try { doSomething(); } finally { super.finalize() } }
ForbidCertainImportsCheck.name = Forbid Certain Imports
ForbidCertainImportsCheck.desc = Forbids certain imports usage in certain packages.
You can configure this check using following parameters:- Package qualified name regexp;
- Forbidden imports regexp;
- Forbidden imports excludes regexp.
This check reads packages and imports qualified names without words "package","import" and semicolons, so, please, do NOT include "package" or "import" words or semicolons into your regular expressions when configuring.
Real-life example of usage: forbid to use all "*.ui.*" packages in "*.dao.*" packages, but ignore all Exception imports (such as org.springframework.dao.InvalidDataAccessResourceUsageException). For doing that, you should use the following check parameters:
Package name regexp = ".*ui.*" Forbidden imports regexp = ".*dao.*" Forbidden imports excludes regexp = "^.+Exception$"
By means of few instances of this check will be possible to cover any rules.
Author: Daniil Yaroslavtsev
ForbidCertainImportsCheck.packageNameRegexp = Package name regexp.
ForbidCertainImportsCheck.forbiddenImportsRegexp = Regexp for matching forbidden imports
ForbidCertainImportsCheck.forbiddenImportsExcludesRegexp = Regexp for excluding imports from checking
ForbidInstantiationCheck.name = Forbid Instantiation
ForbidInstantiationCheck.desc = Forbids instantiation of certain object types by their full classname.
For example:
"java.lang.NullPointerException" will forbid the NPE instantiation.
Note: className should to be full: use "java.lang.NullPointerException" instead of "NullpointerException".
ForbidInstantiationCheck.forbiddenClasses = ClassNames for objects that are forbidden to instantiate.
ForbidCCommentsInMethodsCheck.name = Forbid C comments in method body
ForbidCCommentsInMethodsCheck.desc = Forbid C-style comments (/* ... */) in method body.
ForbidReturnInFinallyBlockCheck.name = Forbid return statement in finally block
ForbidReturnInFinallyBlockCheck.desc = Verifies the finally block design
ForbidThrowAnonymousExceptionsCheck.name = Forbid Throw Anonymous Exceptions
ForbidThrowAnonymousExceptionsCheck.desc = This Check warns on throwing anonymous exception.
Examples:
catch (Exception e) {
throw new RuntimeException() { //WARNING
//some code
};
}
catch (Exception e) {
RuntimeException run = new RuntimeException() {
//some code
};
throw run; //WARNING
}
The distinguishing of exception types occurs by analyzing variable's class's name.Check has an option which contains the regular expression for exception class name matching
Default value is "^.*Exception" because usually exception type ends with suffix "Exception".
Then, if we have an ObjBlock (distinguished by curly braces), it's anonymous
exception definition. It could be defined in throw statement immediately.
In that case, after literal new, there would be an expression type finishing with and ObjBlock.
@author Aleksey Nesterenko
@author Max Vetrenko ForbidThrowAnonymousExceptionsCheck.exceptionClassNameRegex = Regex for exception class name matching DiamondOperatorForVariableDefinitionCheck.name = Diamond Operator For Variable Definition DiamondOperatorForVariableDefinitionCheck.desc = This Check highlights variable definition statements where diamond operator could be used.
Rationale: using diamond operator (introduced in Java 1.7) leads to shorter code
and better code readability. It is suggested by Oracle that the diamond primarily using
for variable declarations.
E.g. of statements:
Without diamond operator: Map<String, Map<String, Integer>> someMap = new HashMap<String, Map<String, Integer>>();
With diamond operator:
Map<String, Map<String, Integer>> someMap = new HashMap<>();
- If you iterate over a map using map.keySet() or map.entrySet(), but your code uses only map values, Check will propose you to use map.values() instead of map.keySet() or map.entrySet(). Replacing map.keySet() or map.entrySet() with map.values() for such cases can a bit improve an iteration performance.
Bad:
for (Map.Entry
; entry : map.entrySet()){
System.out.println(entry.getValue());
}for (String key : map.keySet(){
System.out.println(map.get(key));
}Good:
for (String value : map.values()){
System.out.println(value);
} - If you iterate over a map using map.entrySet(), but never call entry.getValue(), Check will propose you to use map.keySet() instead of map.entrySet(). to iterate over map keys only.
Bad:
for (Map.Entry
entry : map.entrySet()){
System.out.println(entry.getKey());
}Good:
for (String key : map.keySet()){
System.out.println(key);
} - If you iterate over a map with map.keySet() and use both keys and values, check will propose you to use map.entrySet() to improve an iteration performance by avoiding search operations inside a map. For this case, iteration can significantly grow up a performance.
Bad:
for (String key : map.keySet()){
System.out.println(key + " " + map.get(key));
}Good:
for (Map.Entry
entry : map.entrySet()){
System.out.println(entry.getValue() + " " + entry.getKey());
}
\r\nRationale: Code duplication makes maintenance more difficult, so it can be better to replace the multiple occurrences with a constant. MultipleStringLiteralsExtendedCheck.ignoreOccurrenceContext = Token type names where duplicate strings are ignored even if they don't match ignoredStringsRegexp. This allows you to exclude syntactical contexts like Annotations or static initializers from the check. MultipleStringLiteralsExtendedCheck.ignoreStringsRegexp = Regexp pattern for ignored strings (with quotation marks) MultipleStringLiteralsExtendedCheck.name = Multiple String Literals Extended MultipleStringLiteralsExtendedCheck.highlightAllDuplicates = Check to highlight all dublicates TernaryPerExpressionCountCheck.name = Ternary Per Expression Count TernaryPerExpressionCountCheck.desc = Restricts the number of ternary operators in expression to a specific limit.
Rationale: This Check helps to improve code readability by pointing developer on
expressions which contain more than user-defined count of ternary operators.
It points to complicated ternary expressions. Reason:
- Complicated ternary expressions are not easy to read.
- Complicated ternary expressions could lead to ambiguous result if user
does not know Java's operators priority well, e.g.:
String str = null;
String x = str != null ? "A" : "B" + str == null ? "C" : "D";
System.out.println(x);
Check has following properties:
- maxTernaryPerExpressionCount - limit of ternary operators perexpression
- ignoreTernaryOperatorsInBraces - if true Check will ignore ternary operators
in braces (braces explicitly set priority level) - ignoreIsolatedTernaryOnLine - if true Check will ignore one line ternary operators,
if only it is places in line alone.
make Check less strict, e.g.:
Using ignoreTernaryOperatorsInBraces option (value = true)
does not put violation on code below:
callString = "{? = call " +
(StringUtils.hasLength(catalogNameToUse) ? catalogNameToUse + "." : "") +
(StringUtils.hasLength(schemaNameToUse) ? schemaNameToUse + "." : "") +
procedureNameToUse + "(";
ignoreTernaryOperatorsInBraces option Check won't warn on code below:
int a = (d == 5) ? d : f
+
((d == 6) ? g : k);
This check verifies the name of JUnit4 test class for compliance with user defined naming convention(by default Check expects test classes names matching ".+Test\\d*|.+Tests\\d*|Test.+|Tests.+|.+IT|.+ITs|.+TestCase\\d*|.+TestCases\\d*" regex).
Class is considered to be a test if its definition or one of its method definitions annotated with user defined annotations. By default Check looks for classes which contain methods annotated with "Test" or "org.junit.Test".
Check has following options:
"expectedClassNameRegex" - regular expression which matches expected test class names. If test class name does not matches this regex then Check will log violation. This option defaults to ".+Test\\d*|.+Tests\\d*|Test.+|Tests.+|.+IT|.+ITs|.+TestCase\\d*|.+TestCases\\d*".
"classAnnotationNameRegex" - regular expression which matches test annotation names on classes. If class annotated with matching annotation, it is considered to be a test. This option defaults to empty regex(one that matches nothing). If for example this option set to "RunWith", then class "SomeClass" is considered to be a test:
@RunWith(Parameterized.class)
class SomeClass
{
}
"methodAnnotationNameRegex" - regular expression which matches test annotation names on methods. If class contains a method annotated with matching annotation, it is considered to be a test. This option defaults to "Test|org.junit.Test". For example, if this option set to "Test", then class SomeClass" is considered to be a test.
class SomeClass
{
@Test
void method() {
}
}
Annotation names must be specified exactly the same way it specified in code, thus if Check must match annotation with fully qualified name, corresponding options must contain qualified annotation name and vice versa. For example, if annotation regex is "org.junit.Test" Check will recognize "@org.junit.Test" annotation and will skip "@Test" annotation and vice versa if annotation regex is "Test" Check will recognize "@Test" annotation and skip "@org.junit.Test" annotation.
@author Zuy Alexey NameConventionForJunit4TestClassesCheck.expectedClassNameRegex = Regular expression which matches expected test class names. NameConventionForJunit4TestClassesCheck.classAnnotationNameRegex = Regular expression which matches test annotation names on classes. NameConventionForJunit4TestClassesCheck.methodAnnotationNameRegex = Regular expression which matches test annotation names on methods. NoNullForCollectionReturnCheck.name = No null for collection return NoNullForCollectionReturnCheck.desc = Check report you, when method, that must return array or collection, return null value instead of empty collection or empty array. NoNullForCollectionReturnCheck.collectionList = List of the collections that will be check NoNullForCollectionReturnCheck.searchThroughMethodBody = Search null value not only into return block NumericLiteralNeedsUnderscoreCheck.name = Numeric Literal Needs Underscore NumericLiteralNeedsUnderscoreCheck.desc = Warn that long numeric literals should be spaced by underscores. NumericLiteralNeedsUnderscoreCheck.minDecimalSymbolLength = The minimum number of symbols in a decimal literal before the check begins to look for underscores NumericLiteralNeedsUnderscoreCheck.maxDecimalSymbolsUntilUnderscore = The maximum number of symbols in a decimal literal allowed before the check demands an underscore NumericLiteralNeedsUnderscoreCheck.minHexSymbolLength = The minimum number of symbols in a hex literal before the check begins to look for underscores NumericLiteralNeedsUnderscoreCheck.maxHexSymbolsUntilUnderscore = The maximum number of symbols in a hex literal allowed before the check demands an underscore NumericLiteralNeedsUnderscoreCheck.minBinarySymbolLength = The minimum number of symbols in a binary literal before the check begins to look for underscores NumericLiteralNeedsUnderscoreCheck.maxBinarySymbolsUntilUnderscore = The maximum number of symbols in a binary literal allowed before the check demands an underscore NumericLiteralNeedsUnderscoreCheck.ignoreFieldNamePattern = Regexp for fields to ignore OverridableMethodInConstructorCheck.desc =Prevents any calls to overridable methods that are take place in:
- Any constructor body (verification is always done by default and not configurable).
- Any method which works same as a constructor: clone() method from Cloneable interface and readObject() method from Serializable interface (you can individually switch on/of these methods verification by changing CheckCloneMethod and CheckReadObjectMethod properties).
Rationale:
Constructors must not invoke overridable methods, directly or indirectly.If you violate this rule, program failure will result. The superclass constructor runs before the subclass constructor, so the overriding method in the subclass will be invoked before the subclass constructor has run. If the overriding method depends on any initialization performed by the subclass constructor, the method will not behave as expected.
If you do decide to implement Cloneable or Serializable in a class designed for inheritance, you should be aware that because the clone() and readObject() methods behave a lot like constructors, a similar restriction applies: neither clone nor readObject may invoke an overridable method, directly or indirectly.
[Joshua Bloch - Effective Java 2nd Edition,Chapter 4, Item 17]
OverridableMethodInConstructorCheck.name = Overridable Method In Constructor OverridableMethodInConstructorCheck.checkCloneMethod = Enables the searching of calls to overridable methods from body of any clone() method is implemented from Cloneable interface. OverridableMethodInConstructorCheck.checkReadObjectMethod = Enables the searching of calls to overridable methods from the body of any readObject() method is implemented from Serializable interface. OverridableMethodInConstructorCheck.matchMethodsByArgCount = Enables matching methods by number of their parameters ReturnBooleanFromTernaryCheck.name = Returning Boolean from Ternary Operator ReturnBooleanFromTernaryCheck.desc = Avoid returning boolean values from ternary operator - use the boolean value from the inside directly. ReturnCountExtendedCheck.name=Return Count Extended ReturnCountExtendedCheck.desc=Checks that method/ctor "return" literal count is not greater than the given value ("maxReturnCount" property).
Rationale:
One return per method is a good practice as its ease understanding of method logic.
Reasoning is that:
- Methods by name ("ignoreMethodsNames" property). Note, that the "ignoreMethodsNames" property type is NOT regexp: using this property you can list the names of ignored methods separated by comma.
- Methods which linelength less than given value ("linesLimit" property).
- "return" statements which depth is greater or equal to the given value ("returnDepthLimit" property). There are few supported
coding blocks when depth counting: "if-else", "for", "while"/"do-while" and "switch". - "Empty" return statements = return statements in void methods and ctors that have not any expression ("ignoreEmptyReturns" property).
- Return statements, which are located in the top lines of method/ctor (you can specify the count of top method/ctor lines that will be ignored using "rowsToIgnoreCount" property).
ReturnCountExtendedCheck.maxReturnCount=maximum allowed number of return statements per method/ctor (1 by default). ReturnCountExtendedCheck.ignoreMethodLinesCount=Option to ignore methods/ctors which body has the linelength is less than given (20 lines by default). Set "0" to switch this option off and check all methods/ctors. ReturnCountExtendedCheck.minIgnoreReturnDepth=Option to ignore methods/ctors that have return statement(s) with depth value is less than N levels(scopes). 4 by default. 0 is the min depth. Depth is 0 when the "return" statement is not wrapped on one of the supported coding blocks. ReturnCountExtendedCheck.ignoreEmptyReturns=Option to ignore "empty" (with no any expression) return statements in void methods and ctors. 'False' by default. ReturnCountExtendedCheck.topLinesToIgnoreCount=Option to set the count of code lines that will be ignored in top of all methods. ReturnCountExtendedCheck.ignoreMethodsNames=Option to set the RegExp patterns for methods' names which would be ignored by check. ReturnNullInsteadOfBooleanCheck.name = Returning Null Instead of Boolean ReturnNullInsteadOfBooleanCheck.desc = Method declares to return Boolean, but returns null RedundantReturnCheck.name=Redundant Return RedundantReturnCheck.desc=
Highlight usage redundant returns inside constructors and methods with void result.
For example:
1. Non empty constructor
public HelloWorld(){
doStuff();
return;
}
2. Method with void result
public void testMethod1(){
doStuff();
return;
}
However, if your IDE does not support breakpoints on the method entry, you can allow the use of redundant returns in constructors and methods with void result without code except for 'return;'.
For example:
1. Empty constructor
public HelloWorld(){
return;
}
2. Method with void result and empty body
public void testMethod1(){
return;
}
@author Alexey Nesterenko@author Troshin Sergey
@author Max Vetrenko RedundantReturnCheck.allowReturnInEmptyMethodsAndConstructors=If True, allow 'return' in empty constructors and methods that return void. SimpleAccessorNameNotationCheck.name=Simple Accessor Name Notation SimpleAccessorNameNotationCheck.desc=This check verify incorrect name of setter or getter methods if it used field with other name. For example, method has name 'setXXX', but define field with name 'YYY' SimpleAccessorNameNotationCheck.prefix=prefix of field's name SingleBreakOrContinueCheck.name = Single break or continue inside a loop SingleBreakOrContinueCheck.desc =
This check restricts the number of break and continue statements inside cycle body (only one is allowed).
Restricting the number of break and continue statements in a loop is done in the interest of good structured programming.
UnnecessaryParenthesesExtendedCheck.desc = Checks for the use of unnecessary parentheses. UnnecessaryParenthesesExtendedCheck.name = Unnecessary Parentheses Extended UnnecessaryParenthesesExtendedCheck.ignoreCalculationOfBooleanVariables = Cancel validation setups of unnecessary parentheses in Boolean computations. UnnecessaryParenthesesExtendedCheck.ignoreCalculationOfBooleanVariablesWithReturn = Cancel validation setups of unnecessary parentheses in Boolean computations with return state. UnnecessaryParenthesesExtendedCheck.ignoreCalculationOfBooleanVariablesWithAssert = Cancel validation setups of unnecessary parentheses in Boolean computations with assert state. UselessSingleCatchCheck.desc =Checks for the presence of useless single catch blocks.
Catch block can be considered useless if it is the only catch block for try,contains only one statement which rethrows catched exception. Fox example:
try {
...
}
catch(Exception e) {
throw e;
}
UselessSingleCatchCheck.name = Useless single catch check
UselessSuperCtorCallCheck.name = Useless super constructor call
UselessSuperCtorCallCheck.desc = Checks for useless "super()" calls in ctors.
"super()" call could be considered by Check as "useless" in two cases:
Case 1. no-argument "super()" is called from class ctor if class is not derived, for example:
class Dummy {
Dummy() {
super();
}
"super()" call is useless because class "Dummy" is not derived.Case 2. no-argument "super()" is called without parameters from class ctor if class is derived, for example:
class Derived extends Base {
Derived() {
super();
}
}
Java compiler automatically inserts a call to the no-args constructor of the super class, so there is no need to call super ctor explicitly. Check has options "allowCallToNoArgsSuperCtor" and "allowCallToNoArgsSuperCtorIfMultiplePublicCtor" to adjust check behavior for such cases(see Check`s options description for details).Check has following options:
"allowCallToNoArgsSuperCtor" - if this option set to true, Check will not generate violations when "super()" called inside derived class. This option defaults to "false". If for example this option set to "true", then Check will not generate violation for cases like following:
class Base {
public Base() {
}
}
class Derived extends Base {
public Derived() {
super();
}
}
"allowCallToNoArgsSuperCtorIfMultiplePublicCtor" - if this option set to "true",then Check will not generate violation when "super()" called inside class ctor when class has multiple public ctors(however, setting this option to"true" will not prevent Check from logging violation if class does not extend anything). This option defaults to "false". This option may be usefull for cases in which class`s ctors just forward its arguments to super ctors, thus removing "super()" in this case will make default ctors look not like others.For example:
class Base {
public Base() {
}
public Base(int i) {
}
}
class Derived extends Base {
public Derived() {
super(); // this "super()" will not be considered useless if option is set to true,
// because "Derived" has multiple public ctors.
}
public Derived(int i) {
super(i); // this "super()" will not be considered useless if option is set to true,
// because "Derived" has multiple public ctors.
}
}
class NotDerived{
public NotDerived() {
super(); // this "super()" will be considered useless regardless of option value,
// because "NotDerived" does not extend anything.
}
public NotDerived(int i) {
super(); // this "super()" will be considered useless regardless of option value,
// because "NotDerived" does not extend anything.
}
}
@author Zuy Alexey
UselessSuperCtorCallCheck.allowCallToNoArgsSuperCtor = Allow calls to no-arguments super constructor from derived class.
UselessSuperCtorCallCheck.allowCallToNoArgsSuperCtorIfMultiplePublicCtor = Allow calls to no-arguments super constructor from derived class if it has multiple public constructors.
EitherLogOrThrowCheck.desc = Either log the exception, or throw it, but never do both. Logging and throwing results in multiple log messages for a single problem in the code, and makes problems for the support engineer who is trying to dig through the logs. This is one of the most annoying error-handling antipatterns. All of these examples are equally wrong.
Examples:
catch (NoSuchMethodException e) {\t\nLOG.error("Message", e);\t\nthrow e;\n}or
catch (NoSuchMethodException e) {\t\nLOG.error("Message", e);\t\nthrow new MyServiceException("AnotherMessage", e);\n}or
catch (NoSuchMethodException e) {\t\ne.printStackTrace();\t\nthrow new MyServiceException("Message", e);\n}
What check can detect:
Loggers
- logger is declared as class field
- logger is declared as method's local variable
- logger is declared as local variable in
catch
block - logger is passed through method's parameters
- logger logs
catch
parameter exception or it's message - throw
catch
parameter exception - throw another exception which is based on
catch
parameter exception - printStackTrace was called on
catch
parameter exception
- loggers that is used like method's return value. Example:
getLogger().error("message", e)
- loggers that is used like static fields from another classes:
MyAnotherClass.LOGGER.error("message", e);
Default parameters are:
- loggerFullyQualifiedClassName - fully qualified class name of logger type. Default value is "org.slf4j.Logger".
- loggingMethodNames - comma separated names of logging methods. Default value is "error, warn, info, debug".
Note that check works with only one logger type. If you have multiple different loggers, then create another instance of this check.
EitherLogOrThrowCheck.name = Either log exception or throw exception. EitherLogOrThrowCheck.loggerFullyQualifiedClassName = Logger fully qualified class name. Example: "org.slf4j.Logger". EitherLogOrThrowCheck.loggingMethodNames = Logging method names separated with commas. Example: "error,warn". WhitespaceBeforeArrayInitializerCheck.name = Whitespace Before Array Initializer WhitespaceBeforeArrayInitializerCheck.desc = This checks enforces whitespace before array initializer. MoveVariableInsideIfCheck.name = Move Variable Inside If Check MoveVariableInsideIfCheck.desc = Checks if a variable is only used inside if statements and asks for it's declaration to be moved there too.