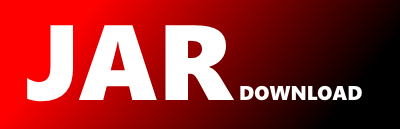
com.power.common.util.CollectionUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common-util Show documentation
Show all versions of common-util Show documentation
ApplicationPower common-util
package com.power.common.util;
import java.util.*;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
import java.util.stream.Stream;
/**
* @author yu
* @since JDK 1.8+
*/
public class CollectionUtil {
/**
* isNotEmpty:(Check if the collection is empty, not empty return true)
*
* @param type of param
* @param collection collection
* @return boolean
*/
public static boolean isNotEmpty(Collection collection) {
return !isEmpty(collection);
}
/**
* checkEmpty:(Check if the collection is empty, return true if it is empty)
*
* @param type of param
* @param collection collection
* @return boolean
*/
public static boolean isEmpty(Collection collection) {
return null == collection || collection.isEmpty();
}
/**
* slice list
*
* @param type of param
* @param source data of list
* @param from from index(included)
* @param to to index(not included)
* @return list
*/
public static List slice(List source, int from, int to) {
if (CollectionUtil.isEmpty(source)) {
return null;
}
int size = source.size();
if (to > size) {
return slice(source.stream(), from, size).collect(Collectors.toList());
} else {
return slice(source.stream(), from, to).collect(Collectors.toList());
}
}
/**
* slice
*
* @param stream Stream
* @param startIndex (included)
* @param endIndex (not included)
* @param type of param
* @return Stream
*/
public static Stream slice(Stream stream, int startIndex, int endIndex) {
return stream
// Convert the stream to list
.collect(Collectors.toList())
// Fetch the subList between the specified index
.subList(startIndex, endIndex)
// Convert the subList to stream
.stream();
}
/**
* partition list by size
*
* @param list List of data
* @param size page size
* @param type of param
* @return List
*/
public static List> partition(List list, final int size) {
if (list != null && list.size() > 0) {
return new ArrayList<>(IntStream.range(0, list.size()).boxed().collect(
Collectors.groupingBy(e -> e / size, Collectors.mapping(e -> list.get(e), Collectors.toList())
)).values());
} else {
throw new NullPointerException("List can't be null");
}
}
/**
* Converts an array to a List, and returns an empty list if the array is empty
*
* @param type of param
* @param a object array
* @return List
*/
public static List asList(T... a) {
if (null != a) {
return Arrays.asList(a);
} else {
return new ArrayList<>(0);
}
}
/**
* Poorly merge the values of the two lists cross-merge, merge in sequence,
* for the case where the set data is not empty,
* The value of result1 The first value is placed first
*
* @param type of param
* @param result1 first of list
* @param result2 second of list
* @return List
*/
public static List mergeAndSwap(List result1, List result2) {
//两个数据都为空,则返回空的结合
if (CollectionUtil.isEmpty(result1) && CollectionUtil.isEmpty(result2)) {
return new ArrayList<>(0);
}
//近使用result2的结果,无需合并
if (CollectionUtil.isEmpty(result1) && CollectionUtil.isNotEmpty(result2)) {
return result2;
}
//仅仅使用result1的结果,无需合并
if (CollectionUtil.isNotEmpty(result1) && CollectionUtil.isEmpty(result2)) {
return result1;
}
int a = result1.size();
int b = result2.size();
int size = a + b;
//两个list的值交叉合并算法(暂时未经过测试)
List finalResult = new ArrayList<>(size);
if (a >= b) {
for (int i = 0; i < size; i++) {
if (i > (b << 1) - 1) {
finalResult.add(result1.get(i - b));
} else {
if ((i & 1) == 0 && i >> 1 < a) {
finalResult.add(result1.get(i >> 1));
}
}
if ((i & 1) == 1 && (i - 1) >> 1 < b) {
finalResult.add(result2.get((i - 1) >> 1));
}
}
} else {
for (int i = 0; i < size; i++) {
if ((i & 1) == 0 && (i >> 1) < a) {
finalResult.add(result1.get(i >> 1));
}
if ((i & 1) == 1 && (i >> 1) < a - 1) {
finalResult.add(result2.get((i - 1) >> 1));
}
if (i >= (a << 1) - 1) {
finalResult.add(result2.get(i - a));
}
}
}
return finalResult;
}
/**
* 将List集合中的无效的map数据清空,被指定为排除的字段
* 即使map中这些指定字段有值,只要其他的key是无效的数据都会被被移除,但是0并不代表没有值
*
* @param list list of map data
* @param exceptKeys except keys
* @return List
*/
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy