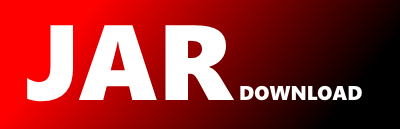
com.power.common.util.IpUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common-util Show documentation
Show all versions of common-util Show documentation
ApplicationPower common-util
package com.power.common.util;
import javax.servlet.http.HttpServletRequest;
import java.net.Inet4Address;
import java.net.Inet6Address;
import java.net.InetAddress;
import java.net.NetworkInterface;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.Map;
public class IpUtil {
private static String serverIp;
static {
InetAddress ia;
try {
ia = InetAddress.getLocalHost();
serverIp = ia.getHostAddress();
} catch (Exception e) {
e.printStackTrace();
}
}
/**
* Get IP address via http request
*
* @param request HttpServletRequest
* @return string
*/
public static String getIpAddress(HttpServletRequest request) {
String ip = request.getHeader("x-forwarded-for");
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("Proxy-Client-IP");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("WL-Proxy-Client-IP");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getRemoteAddr();
}
//cast localhost
if ("0:0:0:0:0:0:0:1".equals(ip)) {
ip = getServerIp();
}
return ip;
}
/**
* Get local server ip address
*
* @return String
*/
public static String getServerIp() {
return serverIp;
}
/**
* Get IPV4 address
*
* @return hash map,key is net interface, value is ip address
*/
public static Map getLocalIPV4() {
Map map = new HashMap<>();
InetAddress ip;
try {
Enumeration netInterfaces = NetworkInterface.getNetworkInterfaces();
while (netInterfaces.hasMoreElements()) {
NetworkInterface ni = netInterfaces.nextElement();
Enumeration ips = ni.getInetAddresses();
while (ips.hasMoreElements()) {
ip = ips.nextElement();
if (ip instanceof Inet4Address) {
map.put(ni.getName(), ip.getHostAddress());
}
}
}
} catch (Exception e) {
e.printStackTrace();
}
return map;
}
/**
* Get IPV6 address
*
* @return hash map,key is net interface, value is ip address
*/
public static Map getLocalIPV6() {
Map map = new HashMap<>();
InetAddress ip;
try {
Enumeration netInterfaces = NetworkInterface.getNetworkInterfaces();
while (netInterfaces.hasMoreElements()) {
NetworkInterface ni = netInterfaces.nextElement();
Enumeration ips = ni.getInetAddresses();
while (ips.hasMoreElements()) {
ip = ips.nextElement();
if (ip instanceof Inet6Address) {
map.put(ni.getName(), ip.getHostAddress());
}
}
}
} catch (Exception e) {
e.printStackTrace();
}
return map;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy