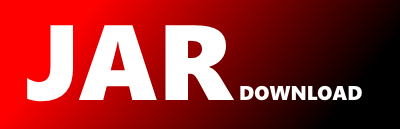
slib.tools.ontofocus.cli.utils.OntoFocusCmdHandlerCst Maven / Gradle / Ivy
/*
* Copyright or © or Copr. Ecole des Mines d'Alès (2012-2014)
*
* This software is a computer program whose purpose is to provide
* several functionalities for the processing of semantic data
* sources such as ontologies or text corpora.
*
* This software is governed by the CeCILL license under French law and
* abiding by the rules of distribution of free software. You can use,
* modify and/ or redistribute the software under the terms of the CeCILL
* license as circulated by CEA, CNRS and INRIA at the following URL
* "http://www.cecill.info".
*
* As a counterpart to the access to the source code and rights to copy,
* modify and redistribute granted by the license, users are provided only
* with a limited warranty and the software's author, the holder of the
* economic rights, and the successive licensors have only limited
* liability.
* In this respect, the user's attention is drawn to the risks associated
* with loading, using, modifying and/or developing or reproducing the
* software by the user in light of its specific status of free software,
* that may mean that it is complicated to manipulate, and that also
* therefore means that it is reserved for developers and experienced
* professionals having in-depth computer knowledge. Users are therefore
* encouraged to load and test the software's suitability as regards their
* requirements in conditions enabling the security of their systems and/or
* data to be ensured and, more generally, to use and operate it in the
* same conditions as regards security.
*
* The fact that you are presently reading this means that you have had
* knowledge of the CeCILL license and that you accept its terms.
*/
package slib.tools.ontofocus.cli.utils;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import org.apache.commons.cli.Option;
import org.apache.commons.cli.OptionBuilder;
import slib.graph.io.util.GFormat;
import slib.tools.module.ToolCmdHandlerCst;
/**
*
* @author Sébastien Harispe
*/
public class OntoFocusCmdHandlerCst extends ToolCmdHandlerCst {
public static final String _appCmdName = "OntoFocus.jar ";
public static final GFormat format_default = GFormat.OBO;
public static final GFormat[] acceptedFormats = {GFormat.OBO, GFormat.RDF_XML};
public static final boolean addRelsVal_default = false;
public static boolean addRelsVal = addRelsVal_default;
/**
*separator used to defined multiple relationships to consider during the process
*/
public static final String incR_Separator = ",";
public static final String errorNoOntology = "[ERROR] Please specify an ontology, supported format are " + Arrays.toString(OntoFocusCmdHandlerCst.acceptedFormats);
public static final String errorNoOutput = "[ERROR] Please specify an output file prefix";
public static final String errorNoQueries = "[ERROR] Please specify a query file which specifies the concepts to consider for the reduction(s) to be performed";
/*
* Options
*/
public static final Option help = new Option("help", "print this message");
public static final Option addR = new Option("addR", "post-process: add all directed relationships which involves two nodes of the final reduction\n"
+ "(optional, default:" + addRelsVal_default + ")");
public static final Option tr = new Option("tr", "post-process (applied after addR): perform a transitive reduction of the taxonomic graph generated by the treatment, i.e. remove taxonomic redundancies");
@SuppressWarnings("static-access")
public static final Option ontology = OptionBuilder.withArgName("file")
.hasArg()
.withDescription("Input file which defines the knowledge representation. The file format must correspond to the default/specified format (required)")
.create("onto");
@SuppressWarnings("static-access")
public static final Option ontoFormat = OptionBuilder.withArgName("value")
.hasArg()
.withDescription("The format of the knowledge representation (optional, default:" + format_default + ", values: " + Arrays.toString(acceptedFormats) + ")")
.create("format");
@SuppressWarnings("static-access")
public static final Option queryFile = OptionBuilder.withArgName("file")
.hasArg()
.withDescription("Input file which defines the reduction(s) to perform (required). For each reduction a configuration is required, it must be specified in a dedicated line according to the following pattern [query_id][TAB][URI],[URI],...,[URI][newline] with [query_id] the identifier of the reduction process (must be unique), [TAB] a tabulation, [URI] an URI specified in the ontology which maps a concept defined in the taxonomy (at least two URIs must be specified, separator ',') ")
.create("queries");
@SuppressWarnings("static-access")
public static final Option incR = OptionBuilder.withArgName("URI")
.hasArg()
.withDescription("URI of other relationships to consider during topological sort and graph reduction."
+ " Taxonomical relationships are always considered."
+ " Useful to include other transitive relationship (over taxonomical relationhips), e.g part-of. "
+ " Notice that the graph composed of all the relationships of the specified types must be acyclic. "
+ " Multiple relationship id/URI can be specified using '" + incR_Separator + "' separator \n"
+ "(optional, default: {taxonomic relationship})")
.create("incR");
@SuppressWarnings("static-access")
public static final Option outprefix = OptionBuilder.withArgName("String")
.hasArg()
.withDescription("The prefix which must be used to generate the output file name (optional). An output file will be generated for each query according to the following pattern [prefix]_[query_id].dot or [query_id].dot if no prefix specified")
.create("outprefix");
@SuppressWarnings("static-access")
public static final Option prefixes = OptionBuilder.withArgName("value")
.hasArg()
.withDescription("URI Prefixes which must be used to resolve prefixed URIs, e.g., GO:XXXXX. Multiple prefixes can be specified using comma as separator (optional, default:none, e.g. : GO=http://go/). Commonly used prefixes such as rdf, owl are already loaded")
.create("prefixes");
@SuppressWarnings("static-access")
public static final Option forceInclude = OptionBuilder.withArgName("value")
.hasArg()
.withDescription("Force specific URIs of concepts to be included in the final reduction (optional), these URIs will be considered as query entries but they will not be colored, e.g., can be useful to include MF, BP, and CC roots of the Gene Ontology (2013) GO:0003674,GO:0005575,GO:0008150. Multiple URIs can be specified using comma as separator and prefixes which have been specified can be used.")
.create("finclude");
/**
* Ordering of the options
*/
public final static Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy