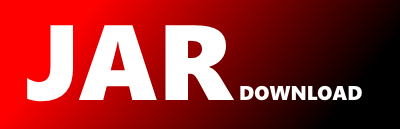
shiver.me.timbers.aws.iot.TopicRuleAction Maven / Gradle / Ivy
Show all versions of smt-cloudformation-objects Show documentation
package shiver.me.timbers.aws.iot;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
import shiver.me.timbers.aws.Property;
/**
* TopicRuleAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-action.html
*
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@JsonPropertyOrder({
"CloudwatchAlarm",
"CloudwatchMetric",
"DynamoDB",
"DynamoDBv2",
"Elasticsearch",
"Firehose",
"Http",
"IotAnalytics",
"IotEvents",
"IotSiteWise",
"Kinesis",
"Lambda",
"Republish",
"S3",
"Sns",
"Sqs",
"StepFunctions"
})
public class TopicRuleAction implements Property
{
/**
* TopicRuleCloudwatchAlarmAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-cloudwatchalarmaction.html
*
*/
@JsonProperty("CloudwatchAlarm")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-cloudwatchalarmaction.html")
private Property cloudwatchAlarm;
/**
* TopicRuleCloudwatchMetricAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-cloudwatchmetricaction.html
*
*/
@JsonProperty("CloudwatchMetric")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-cloudwatchmetricaction.html")
private Property cloudwatchMetric;
/**
* TopicRuleDynamoDBAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-dynamodbaction.html
*
*/
@JsonProperty("DynamoDB")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-dynamodbaction.html")
private Property dynamoDB;
/**
* TopicRuleDynamoDBv2Action
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-dynamodbv2action.html
*
*/
@JsonProperty("DynamoDBv2")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-dynamodbv2action.html")
private Property dynamoDBv2;
/**
* TopicRuleElasticsearchAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-elasticsearchaction.html
*
*/
@JsonProperty("Elasticsearch")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-elasticsearchaction.html")
private Property elasticsearch;
/**
* TopicRuleFirehoseAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-firehoseaction.html
*
*/
@JsonProperty("Firehose")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-firehoseaction.html")
private Property firehose;
/**
* TopicRuleHttpAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-httpaction.html
*
*/
@JsonProperty("Http")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-httpaction.html")
private Property http;
/**
* TopicRuleIotAnalyticsAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-iotanalyticsaction.html
*
*/
@JsonProperty("IotAnalytics")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-iotanalyticsaction.html")
private Property iotAnalytics;
/**
* TopicRuleIotEventsAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-ioteventsaction.html
*
*/
@JsonProperty("IotEvents")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-ioteventsaction.html")
private Property iotEvents;
/**
* TopicRuleIotSiteWiseAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-iotsitewiseaction.html
*
*/
@JsonProperty("IotSiteWise")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-iotsitewiseaction.html")
private Property iotSiteWise;
/**
* TopicRuleKinesisAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-kinesisaction.html
*
*/
@JsonProperty("Kinesis")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-kinesisaction.html")
private Property kinesis;
/**
* TopicRuleLambdaAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-lambdaaction.html
*
*/
@JsonProperty("Lambda")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-lambdaaction.html")
private Property lambda;
/**
* TopicRuleRepublishAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-republishaction.html
*
*/
@JsonProperty("Republish")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-republishaction.html")
private Property republish;
/**
* TopicRuleS3Action
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-s3action.html
*
*/
@JsonProperty("S3")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-s3action.html")
private Property s3;
/**
* TopicRuleSnsAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-snsaction.html
*
*/
@JsonProperty("Sns")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-snsaction.html")
private Property sns;
/**
* TopicRuleSqsAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-sqsaction.html
*
*/
@JsonProperty("Sqs")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-sqsaction.html")
private Property sqs;
/**
* TopicRuleStepFunctionsAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-stepfunctionsaction.html
*
*/
@JsonProperty("StepFunctions")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-stepfunctionsaction.html")
private Property stepFunctions;
/**
* TopicRuleCloudwatchAlarmAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-cloudwatchalarmaction.html
*
*/
@JsonIgnore
public Property getCloudwatchAlarm() {
return cloudwatchAlarm;
}
/**
* TopicRuleCloudwatchAlarmAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-cloudwatchalarmaction.html
*
*/
@JsonIgnore
public void setCloudwatchAlarm(Property cloudwatchAlarm) {
this.cloudwatchAlarm = cloudwatchAlarm;
}
public TopicRuleAction withCloudwatchAlarm(Property cloudwatchAlarm) {
this.cloudwatchAlarm = cloudwatchAlarm;
return this;
}
/**
* TopicRuleCloudwatchMetricAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-cloudwatchmetricaction.html
*
*/
@JsonIgnore
public Property getCloudwatchMetric() {
return cloudwatchMetric;
}
/**
* TopicRuleCloudwatchMetricAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-cloudwatchmetricaction.html
*
*/
@JsonIgnore
public void setCloudwatchMetric(Property cloudwatchMetric) {
this.cloudwatchMetric = cloudwatchMetric;
}
public TopicRuleAction withCloudwatchMetric(Property cloudwatchMetric) {
this.cloudwatchMetric = cloudwatchMetric;
return this;
}
/**
* TopicRuleDynamoDBAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-dynamodbaction.html
*
*/
@JsonIgnore
public Property getDynamoDB() {
return dynamoDB;
}
/**
* TopicRuleDynamoDBAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-dynamodbaction.html
*
*/
@JsonIgnore
public void setDynamoDB(Property dynamoDB) {
this.dynamoDB = dynamoDB;
}
public TopicRuleAction withDynamoDB(Property dynamoDB) {
this.dynamoDB = dynamoDB;
return this;
}
/**
* TopicRuleDynamoDBv2Action
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-dynamodbv2action.html
*
*/
@JsonIgnore
public Property getDynamoDBv2() {
return dynamoDBv2;
}
/**
* TopicRuleDynamoDBv2Action
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-dynamodbv2action.html
*
*/
@JsonIgnore
public void setDynamoDBv2(Property dynamoDBv2) {
this.dynamoDBv2 = dynamoDBv2;
}
public TopicRuleAction withDynamoDBv2(Property dynamoDBv2) {
this.dynamoDBv2 = dynamoDBv2;
return this;
}
/**
* TopicRuleElasticsearchAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-elasticsearchaction.html
*
*/
@JsonIgnore
public Property getElasticsearch() {
return elasticsearch;
}
/**
* TopicRuleElasticsearchAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-elasticsearchaction.html
*
*/
@JsonIgnore
public void setElasticsearch(Property elasticsearch) {
this.elasticsearch = elasticsearch;
}
public TopicRuleAction withElasticsearch(Property elasticsearch) {
this.elasticsearch = elasticsearch;
return this;
}
/**
* TopicRuleFirehoseAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-firehoseaction.html
*
*/
@JsonIgnore
public Property getFirehose() {
return firehose;
}
/**
* TopicRuleFirehoseAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-firehoseaction.html
*
*/
@JsonIgnore
public void setFirehose(Property firehose) {
this.firehose = firehose;
}
public TopicRuleAction withFirehose(Property firehose) {
this.firehose = firehose;
return this;
}
/**
* TopicRuleHttpAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-httpaction.html
*
*/
@JsonIgnore
public Property getHttp() {
return http;
}
/**
* TopicRuleHttpAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-httpaction.html
*
*/
@JsonIgnore
public void setHttp(Property http) {
this.http = http;
}
public TopicRuleAction withHttp(Property http) {
this.http = http;
return this;
}
/**
* TopicRuleIotAnalyticsAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-iotanalyticsaction.html
*
*/
@JsonIgnore
public Property getIotAnalytics() {
return iotAnalytics;
}
/**
* TopicRuleIotAnalyticsAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-iotanalyticsaction.html
*
*/
@JsonIgnore
public void setIotAnalytics(Property iotAnalytics) {
this.iotAnalytics = iotAnalytics;
}
public TopicRuleAction withIotAnalytics(Property iotAnalytics) {
this.iotAnalytics = iotAnalytics;
return this;
}
/**
* TopicRuleIotEventsAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-ioteventsaction.html
*
*/
@JsonIgnore
public Property getIotEvents() {
return iotEvents;
}
/**
* TopicRuleIotEventsAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-ioteventsaction.html
*
*/
@JsonIgnore
public void setIotEvents(Property iotEvents) {
this.iotEvents = iotEvents;
}
public TopicRuleAction withIotEvents(Property iotEvents) {
this.iotEvents = iotEvents;
return this;
}
/**
* TopicRuleIotSiteWiseAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-iotsitewiseaction.html
*
*/
@JsonIgnore
public Property getIotSiteWise() {
return iotSiteWise;
}
/**
* TopicRuleIotSiteWiseAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-iotsitewiseaction.html
*
*/
@JsonIgnore
public void setIotSiteWise(Property iotSiteWise) {
this.iotSiteWise = iotSiteWise;
}
public TopicRuleAction withIotSiteWise(Property iotSiteWise) {
this.iotSiteWise = iotSiteWise;
return this;
}
/**
* TopicRuleKinesisAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-kinesisaction.html
*
*/
@JsonIgnore
public Property getKinesis() {
return kinesis;
}
/**
* TopicRuleKinesisAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-kinesisaction.html
*
*/
@JsonIgnore
public void setKinesis(Property kinesis) {
this.kinesis = kinesis;
}
public TopicRuleAction withKinesis(Property kinesis) {
this.kinesis = kinesis;
return this;
}
/**
* TopicRuleLambdaAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-lambdaaction.html
*
*/
@JsonIgnore
public Property getLambda() {
return lambda;
}
/**
* TopicRuleLambdaAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-lambdaaction.html
*
*/
@JsonIgnore
public void setLambda(Property lambda) {
this.lambda = lambda;
}
public TopicRuleAction withLambda(Property lambda) {
this.lambda = lambda;
return this;
}
/**
* TopicRuleRepublishAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-republishaction.html
*
*/
@JsonIgnore
public Property getRepublish() {
return republish;
}
/**
* TopicRuleRepublishAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-republishaction.html
*
*/
@JsonIgnore
public void setRepublish(Property republish) {
this.republish = republish;
}
public TopicRuleAction withRepublish(Property republish) {
this.republish = republish;
return this;
}
/**
* TopicRuleS3Action
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-s3action.html
*
*/
@JsonIgnore
public Property getS3() {
return s3;
}
/**
* TopicRuleS3Action
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-s3action.html
*
*/
@JsonIgnore
public void setS3(Property s3) {
this.s3 = s3;
}
public TopicRuleAction withS3(Property s3) {
this.s3 = s3;
return this;
}
/**
* TopicRuleSnsAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-snsaction.html
*
*/
@JsonIgnore
public Property getSns() {
return sns;
}
/**
* TopicRuleSnsAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-snsaction.html
*
*/
@JsonIgnore
public void setSns(Property sns) {
this.sns = sns;
}
public TopicRuleAction withSns(Property sns) {
this.sns = sns;
return this;
}
/**
* TopicRuleSqsAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-sqsaction.html
*
*/
@JsonIgnore
public Property getSqs() {
return sqs;
}
/**
* TopicRuleSqsAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-sqsaction.html
*
*/
@JsonIgnore
public void setSqs(Property sqs) {
this.sqs = sqs;
}
public TopicRuleAction withSqs(Property sqs) {
this.sqs = sqs;
return this;
}
/**
* TopicRuleStepFunctionsAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-stepfunctionsaction.html
*
*/
@JsonIgnore
public Property getStepFunctions() {
return stepFunctions;
}
/**
* TopicRuleStepFunctionsAction
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-stepfunctionsaction.html
*
*/
@JsonIgnore
public void setStepFunctions(Property stepFunctions) {
this.stepFunctions = stepFunctions;
}
public TopicRuleAction withStepFunctions(Property stepFunctions) {
this.stepFunctions = stepFunctions;
return this;
}
@Override
public String toString() {
return new ToStringBuilder(this).append("cloudwatchAlarm", cloudwatchAlarm).append("cloudwatchMetric", cloudwatchMetric).append("dynamoDB", dynamoDB).append("dynamoDBv2", dynamoDBv2).append("elasticsearch", elasticsearch).append("firehose", firehose).append("http", http).append("iotAnalytics", iotAnalytics).append("iotEvents", iotEvents).append("iotSiteWise", iotSiteWise).append("kinesis", kinesis).append("lambda", lambda).append("republish", republish).append("s3", s3).append("sns", sns).append("sqs", sqs).append("stepFunctions", stepFunctions).toString();
}
@Override
public int hashCode() {
return new HashCodeBuilder().append(s3).append(firehose).append(dynamoDBv2).append(stepFunctions).append(kinesis).append(dynamoDB).append(iotAnalytics).append(lambda).append(elasticsearch).append(iotEvents).append(republish).append(sqs).append(cloudwatchAlarm).append(http).append(sns).append(iotSiteWise).append(cloudwatchMetric).toHashCode();
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof TopicRuleAction) == false) {
return false;
}
TopicRuleAction rhs = ((TopicRuleAction) other);
return new EqualsBuilder().append(s3, rhs.s3).append(firehose, rhs.firehose).append(dynamoDBv2, rhs.dynamoDBv2).append(stepFunctions, rhs.stepFunctions).append(kinesis, rhs.kinesis).append(dynamoDB, rhs.dynamoDB).append(iotAnalytics, rhs.iotAnalytics).append(lambda, rhs.lambda).append(elasticsearch, rhs.elasticsearch).append(iotEvents, rhs.iotEvents).append(republish, rhs.republish).append(sqs, rhs.sqs).append(cloudwatchAlarm, rhs.cloudwatchAlarm).append(http, rhs.http).append(sns, rhs.sns).append(iotSiteWise, rhs.iotSiteWise).append(cloudwatchMetric, rhs.cloudwatchMetric).isEquals();
}
}