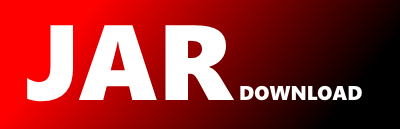
shiver.me.timbers.aws.iot.TopicRuleDynamoDBv2Action Maven / Gradle / Ivy
Show all versions of smt-cloudformation-objects Show documentation
package shiver.me.timbers.aws.iot;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
import shiver.me.timbers.aws.Property;
/**
* TopicRuleDynamoDBv2Action
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-dynamodbv2action.html
*
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@JsonPropertyOrder({
"PutItem",
"RoleArn"
})
public class TopicRuleDynamoDBv2Action implements Property
{
/**
* TopicRulePutItemInput
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-putiteminput.html
*
*/
@JsonProperty("PutItem")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-putiteminput.html")
private Property putItem;
/**
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-dynamodbv2action.html#cfn-iot-topicrule-dynamodbv2action-rolearn
*
*/
@JsonProperty("RoleArn")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-dynamodbv2action.html#cfn-iot-topicrule-dynamodbv2action-rolearn")
private CharSequence roleArn;
/**
* TopicRulePutItemInput
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-putiteminput.html
*
*/
@JsonIgnore
public Property getPutItem() {
return putItem;
}
/**
* TopicRulePutItemInput
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-putiteminput.html
*
*/
@JsonIgnore
public void setPutItem(Property putItem) {
this.putItem = putItem;
}
public TopicRuleDynamoDBv2Action withPutItem(Property putItem) {
this.putItem = putItem;
return this;
}
/**
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-dynamodbv2action.html#cfn-iot-topicrule-dynamodbv2action-rolearn
*
*/
@JsonIgnore
public CharSequence getRoleArn() {
return roleArn;
}
/**
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-iot-topicrule-dynamodbv2action.html#cfn-iot-topicrule-dynamodbv2action-rolearn
*
*/
@JsonIgnore
public void setRoleArn(CharSequence roleArn) {
this.roleArn = roleArn;
}
public TopicRuleDynamoDBv2Action withRoleArn(CharSequence roleArn) {
this.roleArn = roleArn;
return this;
}
@Override
public String toString() {
return new ToStringBuilder(this).append("putItem", putItem).append("roleArn", roleArn).toString();
}
@Override
public int hashCode() {
return new HashCodeBuilder().append(putItem).append(roleArn).toHashCode();
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof TopicRuleDynamoDBv2Action) == false) {
return false;
}
TopicRuleDynamoDBv2Action rhs = ((TopicRuleDynamoDBv2Action) other);
return new EqualsBuilder().append(putItem, rhs.putItem).append(roleArn, rhs.roleArn).isEquals();
}
}