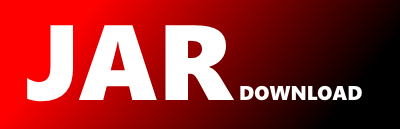
shiver.me.timbers.aws.synthetics.CanaryVPCConfig Maven / Gradle / Ivy
Show all versions of smt-cloudformation-objects Show documentation
package shiver.me.timbers.aws.synthetics;
import java.util.ArrayList;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
import shiver.me.timbers.aws.Property;
/**
* CanaryVPCConfig
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-synthetics-canary-vpcconfig.html
*
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@JsonPropertyOrder({
"VpcId",
"SubnetIds",
"SecurityGroupIds"
})
public class CanaryVPCConfig implements Property
{
/**
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-synthetics-canary-vpcconfig.html#cfn-synthetics-canary-vpcconfig-vpcid
*
*/
@JsonProperty("VpcId")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-synthetics-canary-vpcconfig.html#cfn-synthetics-canary-vpcconfig-vpcid")
private CharSequence vpcId;
/**
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-synthetics-canary-vpcconfig.html#cfn-synthetics-canary-vpcconfig-subnetids
*
*/
@JsonProperty("SubnetIds")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-synthetics-canary-vpcconfig.html#cfn-synthetics-canary-vpcconfig-subnetids")
private List subnetIds = new ArrayList();
/**
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-synthetics-canary-vpcconfig.html#cfn-synthetics-canary-vpcconfig-securitygroupids
*
*/
@JsonProperty("SecurityGroupIds")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-synthetics-canary-vpcconfig.html#cfn-synthetics-canary-vpcconfig-securitygroupids")
private List securityGroupIds = new ArrayList();
/**
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-synthetics-canary-vpcconfig.html#cfn-synthetics-canary-vpcconfig-vpcid
*
*/
@JsonIgnore
public CharSequence getVpcId() {
return vpcId;
}
/**
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-synthetics-canary-vpcconfig.html#cfn-synthetics-canary-vpcconfig-vpcid
*
*/
@JsonIgnore
public void setVpcId(CharSequence vpcId) {
this.vpcId = vpcId;
}
public CanaryVPCConfig withVpcId(CharSequence vpcId) {
this.vpcId = vpcId;
return this;
}
/**
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-synthetics-canary-vpcconfig.html#cfn-synthetics-canary-vpcconfig-subnetids
*
*/
@JsonIgnore
public List getSubnetIds() {
return subnetIds;
}
/**
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-synthetics-canary-vpcconfig.html#cfn-synthetics-canary-vpcconfig-subnetids
*
*/
@JsonIgnore
public void setSubnetIds(List subnetIds) {
this.subnetIds = subnetIds;
}
public CanaryVPCConfig withSubnetIds(List subnetIds) {
this.subnetIds = subnetIds;
return this;
}
/**
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-synthetics-canary-vpcconfig.html#cfn-synthetics-canary-vpcconfig-securitygroupids
*
*/
@JsonIgnore
public List getSecurityGroupIds() {
return securityGroupIds;
}
/**
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-synthetics-canary-vpcconfig.html#cfn-synthetics-canary-vpcconfig-securitygroupids
*
*/
@JsonIgnore
public void setSecurityGroupIds(List securityGroupIds) {
this.securityGroupIds = securityGroupIds;
}
public CanaryVPCConfig withSecurityGroupIds(List securityGroupIds) {
this.securityGroupIds = securityGroupIds;
return this;
}
@Override
public String toString() {
return new ToStringBuilder(this).append("vpcId", vpcId).append("subnetIds", subnetIds).append("securityGroupIds", securityGroupIds).toString();
}
@Override
public int hashCode() {
return new HashCodeBuilder().append(securityGroupIds).append(vpcId).append(subnetIds).toHashCode();
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof CanaryVPCConfig) == false) {
return false;
}
CanaryVPCConfig rhs = ((CanaryVPCConfig) other);
return new EqualsBuilder().append(securityGroupIds, rhs.securityGroupIds).append(vpcId, rhs.vpcId).append(subnetIds, rhs.subnetIds).isEquals();
}
}