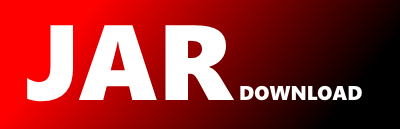
shiver.me.timbers.aws.wafv2.RuleGroupStatementOne Maven / Gradle / Ivy
Show all versions of smt-cloudformation-objects Show documentation
package shiver.me.timbers.aws.wafv2;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
import shiver.me.timbers.aws.Property;
/**
* RuleGroupStatementOne
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-statementone.html
*
*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@JsonPropertyOrder({
"ByteMatchStatement",
"SqliMatchStatement",
"XssMatchStatement",
"SizeConstraintStatement",
"GeoMatchStatement",
"IPSetReferenceStatement",
"RegexPatternSetReferenceStatement",
"RateBasedStatement",
"AndStatement",
"OrStatement",
"NotStatement"
})
public class RuleGroupStatementOne implements Property
{
/**
* RuleGroupByteMatchStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-bytematchstatement.html
*
*/
@JsonProperty("ByteMatchStatement")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-bytematchstatement.html")
private Property byteMatchStatement;
/**
* RuleGroupSqliMatchStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-sqlimatchstatement.html
*
*/
@JsonProperty("SqliMatchStatement")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-sqlimatchstatement.html")
private Property sqliMatchStatement;
/**
* RuleGroupXssMatchStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-xssmatchstatement.html
*
*/
@JsonProperty("XssMatchStatement")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-xssmatchstatement.html")
private Property xssMatchStatement;
/**
* RuleGroupSizeConstraintStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-sizeconstraintstatement.html
*
*/
@JsonProperty("SizeConstraintStatement")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-sizeconstraintstatement.html")
private Property sizeConstraintStatement;
/**
* RuleGroupGeoMatchStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-geomatchstatement.html
*
*/
@JsonProperty("GeoMatchStatement")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-geomatchstatement.html")
private Property geoMatchStatement;
/**
* RuleGroupIPSetReferenceStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-ipsetreferencestatement.html
*
*/
@JsonProperty("IPSetReferenceStatement")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-ipsetreferencestatement.html")
private Property iPSetReferenceStatement;
/**
* RuleGroupRegexPatternSetReferenceStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-regexpatternsetreferencestatement.html
*
*/
@JsonProperty("RegexPatternSetReferenceStatement")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-regexpatternsetreferencestatement.html")
private Property regexPatternSetReferenceStatement;
/**
* RuleGroupRateBasedStatementOne
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-ratebasedstatementone.html
*
*/
@JsonProperty("RateBasedStatement")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-ratebasedstatementone.html")
private Property rateBasedStatement;
/**
* RuleGroupAndStatementOne
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-andstatementone.html
*
*/
@JsonProperty("AndStatement")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-andstatementone.html")
private Property andStatement;
/**
* RuleGroupOrStatementOne
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-orstatementone.html
*
*/
@JsonProperty("OrStatement")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-orstatementone.html")
private Property orStatement;
/**
* RuleGroupNotStatementOne
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-notstatementone.html
*
*/
@JsonProperty("NotStatement")
@JsonPropertyDescription("http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-notstatementone.html")
private Property notStatement;
/**
* RuleGroupByteMatchStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-bytematchstatement.html
*
*/
@JsonIgnore
public Property getByteMatchStatement() {
return byteMatchStatement;
}
/**
* RuleGroupByteMatchStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-bytematchstatement.html
*
*/
@JsonIgnore
public void setByteMatchStatement(Property byteMatchStatement) {
this.byteMatchStatement = byteMatchStatement;
}
public RuleGroupStatementOne withByteMatchStatement(Property byteMatchStatement) {
this.byteMatchStatement = byteMatchStatement;
return this;
}
/**
* RuleGroupSqliMatchStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-sqlimatchstatement.html
*
*/
@JsonIgnore
public Property getSqliMatchStatement() {
return sqliMatchStatement;
}
/**
* RuleGroupSqliMatchStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-sqlimatchstatement.html
*
*/
@JsonIgnore
public void setSqliMatchStatement(Property sqliMatchStatement) {
this.sqliMatchStatement = sqliMatchStatement;
}
public RuleGroupStatementOne withSqliMatchStatement(Property sqliMatchStatement) {
this.sqliMatchStatement = sqliMatchStatement;
return this;
}
/**
* RuleGroupXssMatchStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-xssmatchstatement.html
*
*/
@JsonIgnore
public Property getXssMatchStatement() {
return xssMatchStatement;
}
/**
* RuleGroupXssMatchStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-xssmatchstatement.html
*
*/
@JsonIgnore
public void setXssMatchStatement(Property xssMatchStatement) {
this.xssMatchStatement = xssMatchStatement;
}
public RuleGroupStatementOne withXssMatchStatement(Property xssMatchStatement) {
this.xssMatchStatement = xssMatchStatement;
return this;
}
/**
* RuleGroupSizeConstraintStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-sizeconstraintstatement.html
*
*/
@JsonIgnore
public Property getSizeConstraintStatement() {
return sizeConstraintStatement;
}
/**
* RuleGroupSizeConstraintStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-sizeconstraintstatement.html
*
*/
@JsonIgnore
public void setSizeConstraintStatement(Property sizeConstraintStatement) {
this.sizeConstraintStatement = sizeConstraintStatement;
}
public RuleGroupStatementOne withSizeConstraintStatement(Property sizeConstraintStatement) {
this.sizeConstraintStatement = sizeConstraintStatement;
return this;
}
/**
* RuleGroupGeoMatchStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-geomatchstatement.html
*
*/
@JsonIgnore
public Property getGeoMatchStatement() {
return geoMatchStatement;
}
/**
* RuleGroupGeoMatchStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-geomatchstatement.html
*
*/
@JsonIgnore
public void setGeoMatchStatement(Property geoMatchStatement) {
this.geoMatchStatement = geoMatchStatement;
}
public RuleGroupStatementOne withGeoMatchStatement(Property geoMatchStatement) {
this.geoMatchStatement = geoMatchStatement;
return this;
}
/**
* RuleGroupIPSetReferenceStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-ipsetreferencestatement.html
*
*/
@JsonIgnore
public Property getIPSetReferenceStatement() {
return iPSetReferenceStatement;
}
/**
* RuleGroupIPSetReferenceStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-ipsetreferencestatement.html
*
*/
@JsonIgnore
public void setIPSetReferenceStatement(Property iPSetReferenceStatement) {
this.iPSetReferenceStatement = iPSetReferenceStatement;
}
public RuleGroupStatementOne withIPSetReferenceStatement(Property iPSetReferenceStatement) {
this.iPSetReferenceStatement = iPSetReferenceStatement;
return this;
}
/**
* RuleGroupRegexPatternSetReferenceStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-regexpatternsetreferencestatement.html
*
*/
@JsonIgnore
public Property getRegexPatternSetReferenceStatement() {
return regexPatternSetReferenceStatement;
}
/**
* RuleGroupRegexPatternSetReferenceStatement
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-regexpatternsetreferencestatement.html
*
*/
@JsonIgnore
public void setRegexPatternSetReferenceStatement(Property regexPatternSetReferenceStatement) {
this.regexPatternSetReferenceStatement = regexPatternSetReferenceStatement;
}
public RuleGroupStatementOne withRegexPatternSetReferenceStatement(Property regexPatternSetReferenceStatement) {
this.regexPatternSetReferenceStatement = regexPatternSetReferenceStatement;
return this;
}
/**
* RuleGroupRateBasedStatementOne
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-ratebasedstatementone.html
*
*/
@JsonIgnore
public Property getRateBasedStatement() {
return rateBasedStatement;
}
/**
* RuleGroupRateBasedStatementOne
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-ratebasedstatementone.html
*
*/
@JsonIgnore
public void setRateBasedStatement(Property rateBasedStatement) {
this.rateBasedStatement = rateBasedStatement;
}
public RuleGroupStatementOne withRateBasedStatement(Property rateBasedStatement) {
this.rateBasedStatement = rateBasedStatement;
return this;
}
/**
* RuleGroupAndStatementOne
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-andstatementone.html
*
*/
@JsonIgnore
public Property getAndStatement() {
return andStatement;
}
/**
* RuleGroupAndStatementOne
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-andstatementone.html
*
*/
@JsonIgnore
public void setAndStatement(Property andStatement) {
this.andStatement = andStatement;
}
public RuleGroupStatementOne withAndStatement(Property andStatement) {
this.andStatement = andStatement;
return this;
}
/**
* RuleGroupOrStatementOne
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-orstatementone.html
*
*/
@JsonIgnore
public Property getOrStatement() {
return orStatement;
}
/**
* RuleGroupOrStatementOne
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-orstatementone.html
*
*/
@JsonIgnore
public void setOrStatement(Property orStatement) {
this.orStatement = orStatement;
}
public RuleGroupStatementOne withOrStatement(Property orStatement) {
this.orStatement = orStatement;
return this;
}
/**
* RuleGroupNotStatementOne
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-notstatementone.html
*
*/
@JsonIgnore
public Property getNotStatement() {
return notStatement;
}
/**
* RuleGroupNotStatementOne
*
* http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-wafv2-rulegroup-notstatementone.html
*
*/
@JsonIgnore
public void setNotStatement(Property notStatement) {
this.notStatement = notStatement;
}
public RuleGroupStatementOne withNotStatement(Property notStatement) {
this.notStatement = notStatement;
return this;
}
@Override
public String toString() {
return new ToStringBuilder(this).append("byteMatchStatement", byteMatchStatement).append("sqliMatchStatement", sqliMatchStatement).append("xssMatchStatement", xssMatchStatement).append("sizeConstraintStatement", sizeConstraintStatement).append("geoMatchStatement", geoMatchStatement).append("iPSetReferenceStatement", iPSetReferenceStatement).append("regexPatternSetReferenceStatement", regexPatternSetReferenceStatement).append("rateBasedStatement", rateBasedStatement).append("andStatement", andStatement).append("orStatement", orStatement).append("notStatement", notStatement).toString();
}
@Override
public int hashCode() {
return new HashCodeBuilder().append(sqliMatchStatement).append(xssMatchStatement).append(notStatement).append(byteMatchStatement).append(sizeConstraintStatement).append(regexPatternSetReferenceStatement).append(andStatement).append(geoMatchStatement).append(iPSetReferenceStatement).append(orStatement).append(rateBasedStatement).toHashCode();
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof RuleGroupStatementOne) == false) {
return false;
}
RuleGroupStatementOne rhs = ((RuleGroupStatementOne) other);
return new EqualsBuilder().append(sqliMatchStatement, rhs.sqliMatchStatement).append(xssMatchStatement, rhs.xssMatchStatement).append(notStatement, rhs.notStatement).append(byteMatchStatement, rhs.byteMatchStatement).append(sizeConstraintStatement, rhs.sizeConstraintStatement).append(regexPatternSetReferenceStatement, rhs.regexPatternSetReferenceStatement).append(andStatement, rhs.andStatement).append(geoMatchStatement, rhs.geoMatchStatement).append(iPSetReferenceStatement, rhs.iPSetReferenceStatement).append(orStatement, rhs.orStatement).append(rateBasedStatement, rhs.rateBasedStatement).isEquals();
}
}